Optimizing Spring's WebClient Testing
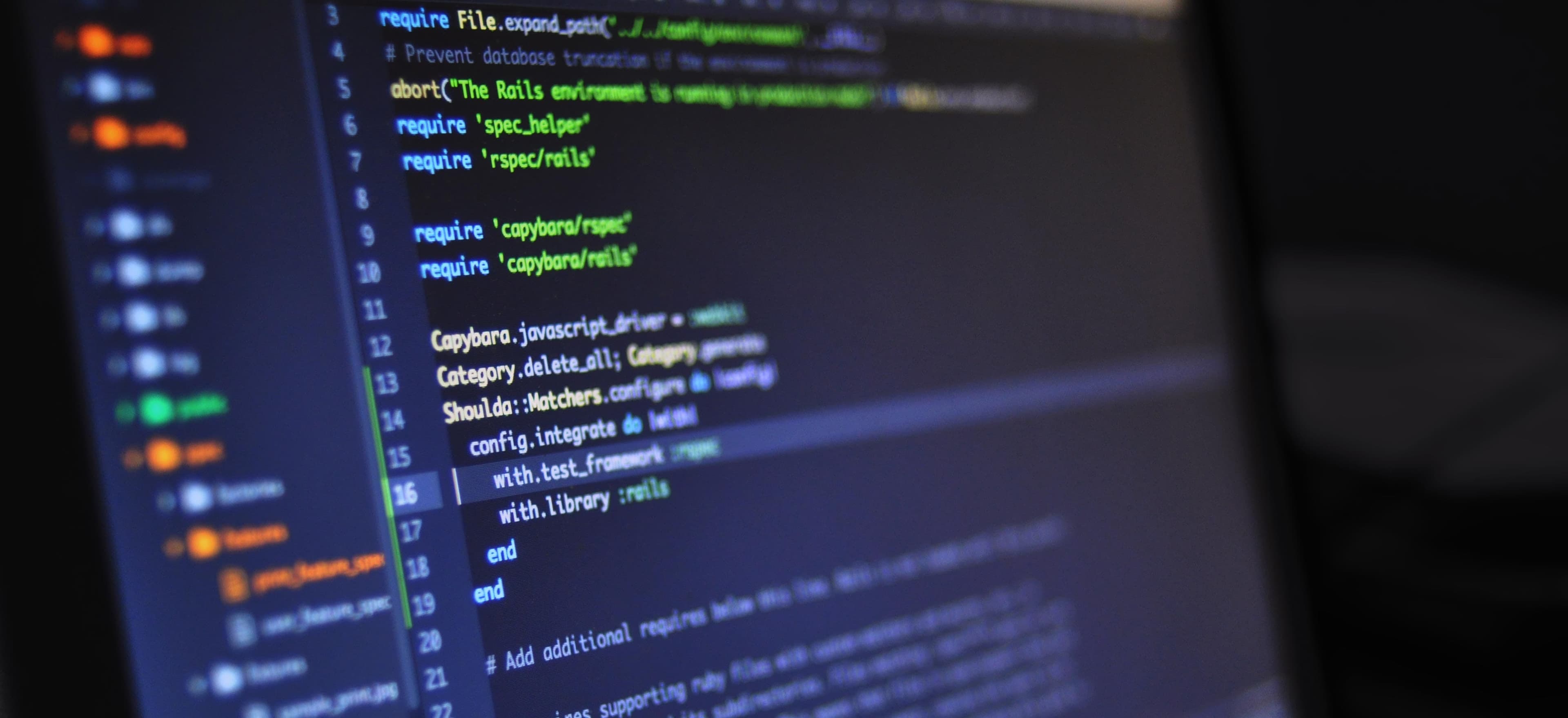
- Published on
Optimizing Spring's WebClient Testing
When it comes to testing WebClient in a Spring application, it's essential to ensure that the tests are not only comprehensive but also optimized for efficiency. In this article, we'll explore some tips and best practices for optimizing WebClient testing in a Java application using the Spring framework.
Understanding WebClient Testing
WebClient is a non-blocking, reactive HTTP client provided by the Spring Framework. It is widely used for making HTTP requests to external services or APIs. When testing code that uses WebClient, it's crucial to write tests that not only validate the functionality but also execute efficiently.
Tip 1: Using WebTestClient
When testing WebClient in a Spring application, the preferred approach is to use WebTestClient. This specialized test client is designed specifically for testing web applications and provides a fluent API for making requests and validating responses. Let's consider an example of using WebTestClient to test a simple endpoint:
@WebFluxTest
public class MyControllerTest {
@Autowired
private WebTestClient webTestClient;
@Test
public void testGetEndpoint() {
webTestClient.get().uri("/api/resource")
.exchange()
.expectStatus().isOk()
.expectBody(String.class)
.isEqualTo("Hello, World!");
}
}
In this example, we're using WebTestClient to make a GET request to the /api/resource
endpoint and validating the response. It provides a concise and expressive way to write tests for WebClient-based code.
Tip 2: Mocking External Services
When testing code that uses WebClient to communicate with external services, it's crucial to mock those external services to avoid making actual network requests during the tests. This not only enhances the speed of the tests but also makes them less brittle and deterministic.
@WebFluxTest
public class MyServiceTest {
@Autowired
private MyService myService;
@MockBean
private WebClient.Builder webClientBuilder;
@Test
public void testApiCall() {
given(webClientBuilder.build()).willReturn(mock(WebClient.class));
// Mock the response from the external API
when(webClientBuilder.build().get().uri(anyString()).retrieve())
.thenReturn(Mono.just(ClientResponse.create(HttpStatus.OK)
.body("Hello, World!").build()));
String result = myService.callExternalApi();
assertThat(result).isEqualTo("Hello, World!");
}
}
In this example, we're mocking the WebClient.Builder bean and simulating the response from the external API using given
and when
from the Mockito library. This enables us to test the behavior of the service without actually hitting the external API.
Tip 3: Managing Dependencies
When writing tests for code that uses WebClient, it's important to manage the dependencies in such a way that the tests remain isolated and independent. This involves properly setting up and tearing down any resources used by the WebClient during the tests.
@WebFluxTest
public class MyServiceTest {
@MockBean
private WebClient.Builder webClientBuilder;
@Test
public void testApiCall() {
// Test code here
}
@AfterEach
public void cleanup() {
reset(webClientBuilder); // Reset the mock to ensure independence between tests
}
}
In this example, we're using JUnit's @AfterEach
annotation to ensure that the WebClient.Builder mock is reset after each test, thereby maintaining the independence of the tests.
Tip 4: Concurrency Testing
Given that WebClient is a non-blocking, reactive client, it's important to test its behavior under concurrent conditions. This involves writing tests that simulate concurrent requests and ensuring that the WebClient-based code behaves as expected under such scenarios.
@WebFluxTest
public class MyServiceTest {
@MockBean
private WebClient.Builder webClientBuilder;
@Test
public void testConcurrentApiCalls() {
Flux<String> results = Flux.range(1, 10)
.parallel()
.runOn(Schedulers.parallel())
.flatMap(i -> Mono.fromCallable(() -> callExternalApi()))
.ordered(String::compareTo);
StepVerifier.create(results)
.expectNextCount(10)
.verifyComplete();
}
}
In this example, we're using Project Reactor's Flux
and StepVerifier
to test the behavior of the WebClient-based code under concurrent API calls. By leveraging reactive programming constructs, we can ensure that the code performs as expected in concurrent scenarios.
The Closing Argument
Optimizing WebClient testing in a Spring application is crucial for ensuring the reliability and performance of the code. By using WebTestClient, mocking external services, managing dependencies, and testing concurrency, developers can write efficient and effective tests for code that uses WebClient.
In conclusion, optimizing WebClient testing not only enhances the reliability of the application but also improves the overall development process. By following these best practices, developers can ensure that their WebClient-based code is thoroughly tested and performs optimally in a variety of scenarios.
Testing WebClient in a Spring application doesn’t have to be daunting. By adopting these best practices, developers can streamline the testing process and ensure that their WebClient-based code is robust and reliable.
For further reading on WebClient testing, refer to the Spring Framework documentation and the Project Reactor documentation. These resources provide comprehensive insights into testing WebClient and reactive programming in a Spring application.