Injecting Mockito Test Doubles in Spring
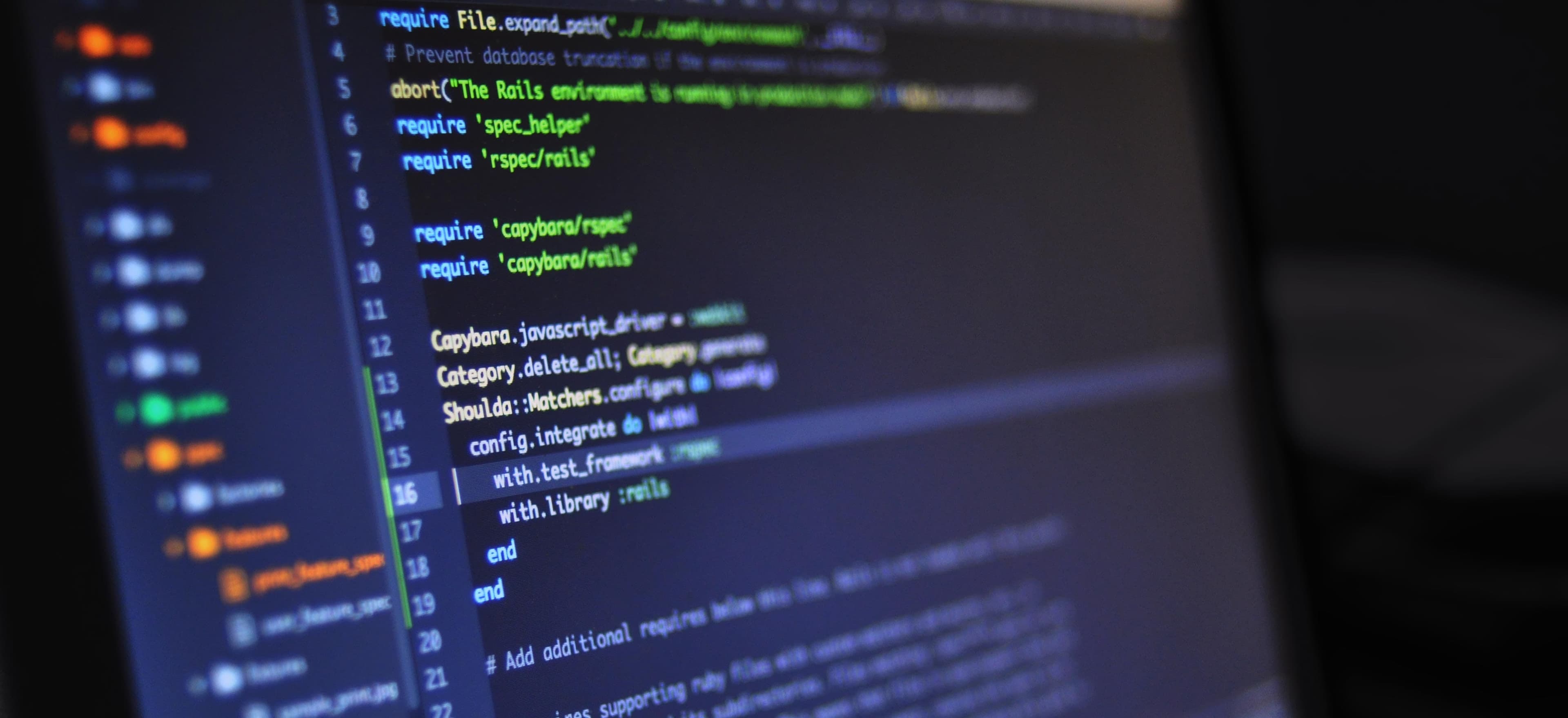
- Published on
Injecting Mockito Test Doubles in Spring: A Comprehensive Guide
Mockito is a powerful Java mocking framework that facilitates the creation of test doubles for unit testing. When it comes to Spring applications, integrating Mockito test doubles becomes essential for writing effective unit tests. In this blog post, we will explore how to effectively integrate Mockito test doubles in Spring for seamless and robust unit testing.
Why Use Mockito Test Doubles?
Before delving into the integration process, let’s highlight the significance of using Mockito test doubles in Spring applications. Mockito enables the creation of mock objects, allowing developers to isolate the functionality they want to test and simulate the behavior of dependencies. This not only helps in writing focused and efficient unit tests but also reduces the reliance on external systems or complex setups during testing.
Adding Mockito Dependency
To begin with, let’s ensure that the Mockito dependency is included in the project’s pom.xml
file. Mockito can be added as a dependency using the following configuration:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.9.0</version>
<scope>test</scope>
</dependency>
By including Mockito as a test scope dependency, it clearly signifies that it is utilized solely for testing purposes.
Creating Mockito Test Doubles
In Spring applications, the need often arises to create test doubles for classes and interfaces that are injected as dependencies. Mockito provides a simple and concise way to create mock objects for these dependencies. Let’s take a look at how to create a Mockito mock object for a specific dependency.
import static org.mockito.Mockito.*;
// Creating a mock object for the DependencyClass
DependencyClass mockedDependency = mock(DependencyClass.class);
The mock
method provided by Mockito allows the creation of a mock object for the specified class. This mock object can then be used to define the behavior of methods and verify interactions during unit testing.
Integrating Mockito Test Doubles in Spring
Now, let’s dive into the process of integrating Mockito test doubles in Spring. There are several approaches to achieve this, and we will explore the two most commonly used methods.
Method 1: Using @Mock Annotation and MockitoJUnitRunner
One approach to integrate Mockito test doubles in Spring is by utilizing the @Mock
annotation along with the MockitoJUnitRunner
for JUnit 4 or MockitoExtension
for JUnit 5. This approach provides seamless integration of Mockito mock objects with the Spring test context.
Here’s an example of how to use the @Mock
annotation and MockitoJUnitRunner
:
import org.junit.runner.RunWith;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnitRunner;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit.jupiter.SpringExtension;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(MockitoJUnitRunner.class)
@SpringBootTest
public class SampleServiceTest {
@Mock
private DependencyClass mockedDependency;
@Autowired
private SampleService sampleService;
// Test methods using mockedDependency
}
In this example, the @Mock
annotation is used to create a mock object for the DependencyClass
which is injected into the SampleService
. The MockitoJUnitRunner
ensures that the mock objects are properly integrated with the Spring test context.
Method 2: Using @MockBean Annotation
Another approach to integrate Mockito test doubles in Spring is by leveraging the @MockBean
annotation provided by the Spring Test support. This approach is specifically tailored for injecting Mockito test doubles into Spring beans for unit testing.
Here’s an example of how to use the @MockBean
annotation:
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.test.context.junit.jupiter.SpringExtension;
@SpringBootTest
public class SampleServiceTest {
@MockBean
private DependencyClass mockedDependency;
@Autowired
private SampleService sampleService;
// Test methods using mockedDependency
}
In this example, the @MockBean
annotation is used to inject a Mockito mock object for the DependencyClass
into the Spring test context. This enables seamless integration of the mock object with the SampleService
for unit testing.
Wrapping Up
In conclusion, integrating Mockito test doubles in Spring applications is crucial for writing effective and focused unit tests. By leveraging Mockito’s capabilities, developers can create mock objects for dependencies and seamlessly integrate them into their Spring unit tests. Whether it’s using the @Mock
annotation with MockitoJUnitRunner
or the @MockBean
annotation provided by Spring Test support, Mockito test doubles play a significant role in enhancing the robustness of unit tests within Spring applications.
By following the approaches outlined in this guide, developers can ensure that their Spring unit tests are well-equipped with Mockito test doubles, paving the way for comprehensive test coverage and reliable code quality.
Gain a deeper understanding of Mockito and Spring Framework to refine your Java development skills further.
Happy testing!