Optimizing JavaScript Memory Usage
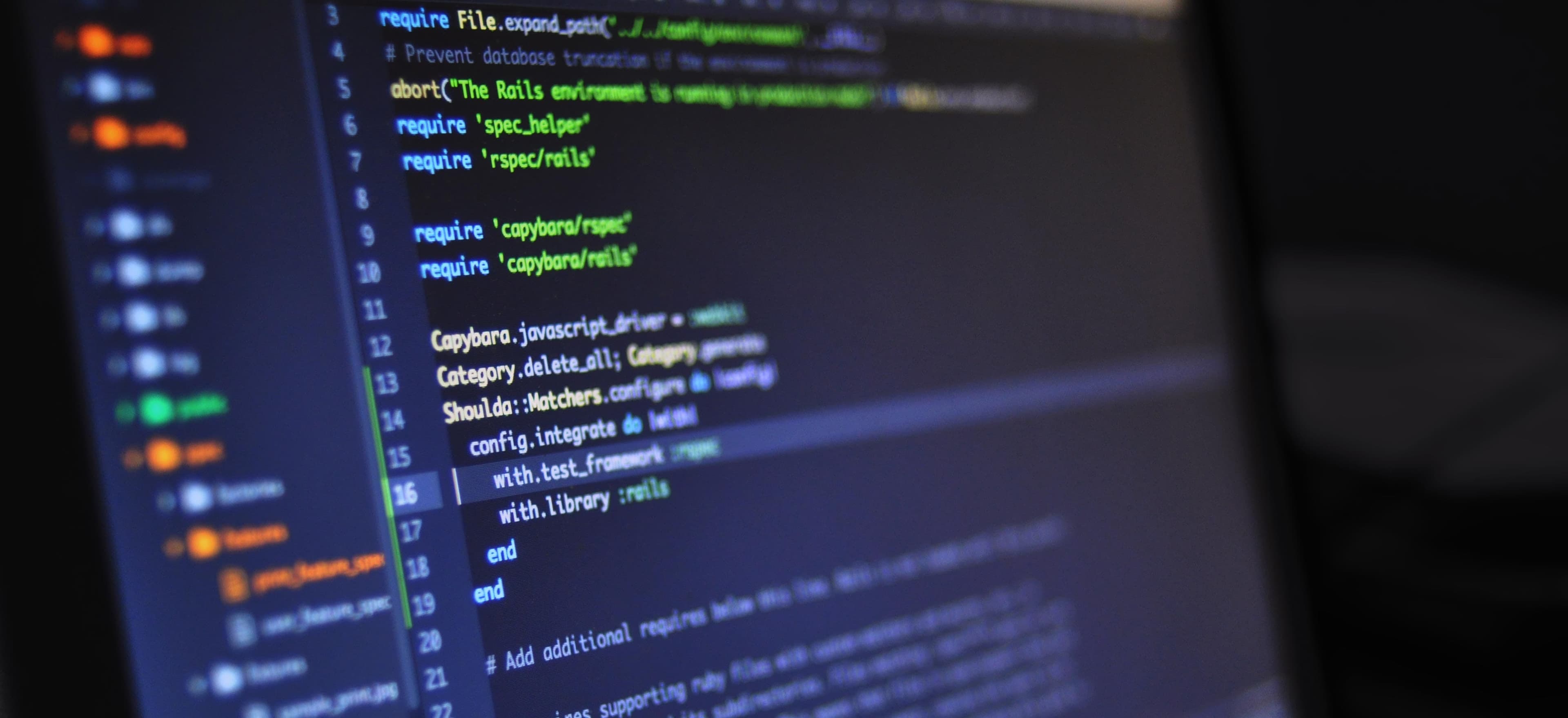
- Published on
Optimizing JavaScript Memory Usage
When it comes to developing efficient and performant applications in JavaScript, optimizing memory usage is a crucial factor. Bloated memory usage can lead to slower performance, increased load times, and even crashes in extreme cases. In this article, we'll delve into strategies for optimizing memory usage in JavaScript, including identifying memory leaks, minimizing memory usage, and employing best practices for efficient memory management.
Identifying Memory Leaks
Memory leaks occur when a piece of memory that is no longer needed is not released. In JavaScript, memory leaks often occur when references to objects are unintentionally maintained, preventing garbage collection from reclaiming them. Tools such as Chrome DevTools' Memory panel can help identify memory leaks by monitoring memory usage and detecting any abnormal increases over time.
Example of Identifying Memory Leaks:
// Setting up memory leak by creating global variable
let globalVar = [];
function createMemoryLeak() {
for (let i = 0; i < 10000; i++) {
globalVar.push(new Array(1000).join('*'));
}
}
createMemoryLeak();
In this code snippet, globalVar
maintains references to the created arrays, preventing them from being garbage collected, thus creating a memory leak.
Identifying and fixing memory leaks is the first step to optimizing memory usage in JavaScript applications.
Minimizing Memory Usage
1. Efficient Data Structures
Choosing the right data structures can significantly impact memory usage. For example, using Map
or Set
instead of plain objects or arrays for storing key-value pairs or unique values can result in better memory utilization.
Example of Efficient Data Structures:
// Using Map for efficient memory usage
let map = new Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
2. Limiting Variable Scope
Limiting the scope of variables to only where they are needed can help in optimizing memory usage. A smaller scope ensures that variables are eligible for garbage collection sooner, freeing up memory.
Example of Limiting Variable Scope:
// Variable with limited scope
function calculateSomething() {
let tempResult = performComplexCalculation();
// tempResult goes out of scope after this function
return tempResult;
}
3. Object Pooling
Object pooling involves reusing objects instead of creating and destroying them repeatedly. This approach can be beneficial for objects that are frequently instantiated, helping to reduce memory allocation and deallocation overhead.
Example of Object Pooling:
// Object pooling for reusing objects
let objPool = [];
function getObject() {
if (objPool.length) {
return objPool.pop();
} else {
return createNewObject();
}
}
function releaseObject(obj) {
obj.reset();
objPool.push(obj);
}
Employing strategies such as efficient data structures, limiting variable scope, and object pooling can contribute to minimizing memory usage in JavaScript applications.
Efficient Memory Management Best Practices
1. Garbage Collection Optimization
Understanding how the JavaScript garbage collector works can aid in optimizing memory management. For instance, being mindful of creating long-lived objects and minimizing the use of global variables can help in efficient garbage collection.
2. Memory Profiling
Utilizing tools for memory profiling, such as Chrome DevTools or the Node.js built-in inspector, allows developers to analyze memory consumption, detect memory leaks, and optimize memory usage in their applications.
3. Using Minification and Compression
Minifying and compressing JavaScript files can reduce memory usage by minimizing the size of the code. This, in turn, leads to faster downloads and optimized memory consumption.
Closing Thoughts
Optimizing memory usage in JavaScript is a critical aspect of building high-performance applications. By identifying memory leaks, minimizing memory usage through efficient data structures and variable scope, and following best practices for memory management, developers can ensure their JavaScript applications run smoothly with optimal memory utilization.
Implementing these strategies can lead to applications that are not only more responsive and faster but also more stable, ultimately providing a better user experience.
Remember, efficient memory usage is not just about writing code that works, but also about writing code that works well.
For additional reading on optimizing JavaScript memory usage, you can refer to the following resources:
Start optimizing your JavaScript memory usage today for better performing applications!