Optimizing GitHub and Jenkins Pull Request Validation
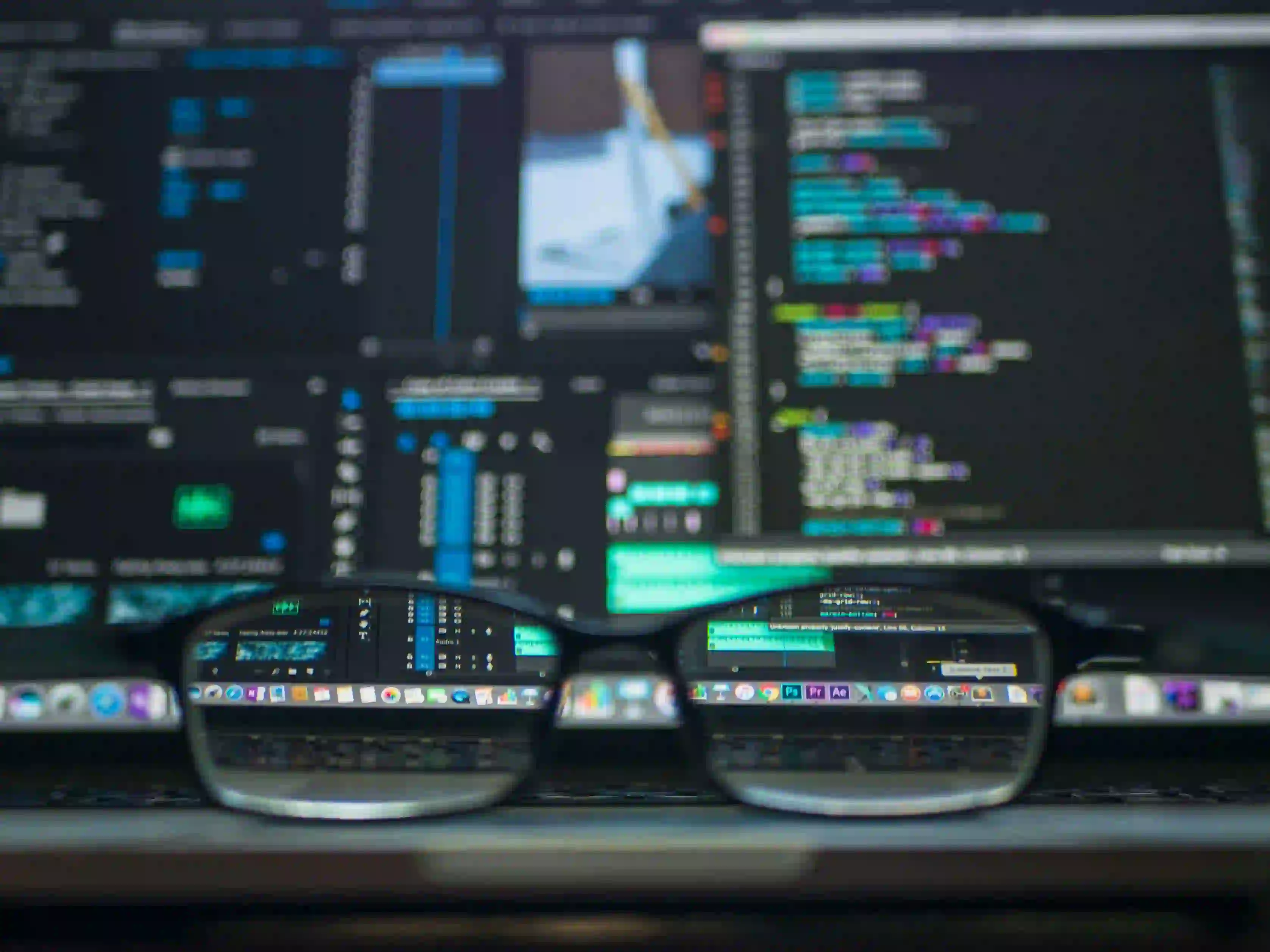
Optimizing GitHub and Jenkins Pull Request Validation
In modern software development, continuous integration and continuous delivery (CI/CD) are crucial for ensuring the quality of code and accelerating the release process. GitHub and Jenkins are two widely used platforms that play a vital role in this process. In this article, we'll explore the optimization of GitHub and Jenkins pull request validation to streamline the development workflow and ensure the reliability of the codebase.
Understanding Pull Request Validation
Before diving into optimization, let's grasp the concept of pull request validation. When a developer creates a pull request in a GitHub repository, it's essential to verify the proposed changes to prevent introducing bugs or breaking existing functionality. This validation process involves running automated tests, code quality checks, and other validation steps to ensure that the code meets the defined standards.
Jenkins, as a popular automation server, seamlessly integrates with GitHub to automate various tasks, including pull request validation. By leveraging Jenkins, you can orchestrate diverse build and validation processes, providing timely feedback on pull requests and ensuring that only high-quality code gets merged into the main branch.
Optimization Strategies
Parallel Test Execution
One of the fundamental optimization strategies for pull request validation involves parallel test execution. By distributing tests across multiple agents or nodes, you can significantly reduce the overall test execution time, thereby accelerating the feedback loop for pull requests. Jenkins provides robust capabilities for parallelizing test execution, allowing you to harness the full potential of your CI/CD pipeline.
Consider the following parallel test execution example using Jenkins declarative pipeline syntax:
pipeline {
agent { label 'docker' }
stages {
stage('Parallel Tests') {
parallel {
stage('Run Unit Tests') {
steps {
sh 'mvn test'
}
}
stage('Run Integration Tests') {
steps {
sh 'mvn integration-test'
}
}
}
}
}
}
In the above example, the pipeline runs unit tests and integration tests in parallel, maximizing the utilization of available resources and expediting the validation process for pull requests.
Conditional Build Triggers
Optimizing pull request validation also involves leveraging conditional build triggers to selectively execute certain validation steps based on specific conditions. This approach minimizes unnecessary build and validation actions, conserving resources and reducing the overall validation time. Jenkins facilitates the implementation of conditional build triggers through its flexible pipeline syntax.
Consider the following example illustrating conditional build triggers in a Jenkins pipeline:
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build the project
}
}
stage('Run Tests') {
when {
expression {
return env.CHANGE_TARGET == 'develop'
}
}
steps {
// Execute tests
}
}
stage('Static Analysis') {
when {
changeRequest target: 'master', branch: 'feature/*'
}
steps {
// Perform static code analysis
}
}
}
}
In this example, the "Run Tests" stage is conditionally executed only when the pull request targets the 'develop' branch, while the "Static Analysis" stage is triggered exclusively for pull requests targeting the 'master' branch with the 'feature/*' pattern.
Caching Dependencies
Efficient management of dependencies is critical for optimizing pull request validation. By caching dependencies, you can minimize the overhead associated with downloading and installing the required dependencies for each pull request build, thereby expediting the build and validation process. Jenkins provides native support for caching dependencies within pipeline jobs, allowing you to enhance the overall build performance.
Consider the following snippet demonstrating dependency caching in a Jenkins pipeline:
pipeline {
agent any
options {
skipDefaultCheckout()
}
stages {
stage('Checkout') {
steps {
checkout scm
}
}
stage('Build') {
steps {
withMaven(maven: 'Maven 3.6.3') {
// Build the project and cache dependencies
}
}
}
}
}
In this example, the "Build" stage leverages the withMaven
block to build the project and automatically cache the dependencies, optimizing subsequent builds and validations.
The Bottom Line
In conclusion, optimizing GitHub and Jenkins pull request validation involves employing strategies such as parallel test execution, conditional build triggers, and dependency caching to enhance the efficiency and responsiveness of the validation process. By fine-tuning the CI/CD pipeline using these optimization techniques, development teams can ensure swift feedback on pull requests, maintain code quality, and accelerate the delivery of reliable software.
By implementing these optimization strategies, teams can refine their CI/CD pipelines, streamline the development process, and foster a culture of continuous improvement and innovation.
To dive deeper into pull request validation and Jenkins best practices, check out the following resources:
Optimize your pull request validation process today and elevate your software development workflow to new heights!