Securing Java EE Applications from Data Theft
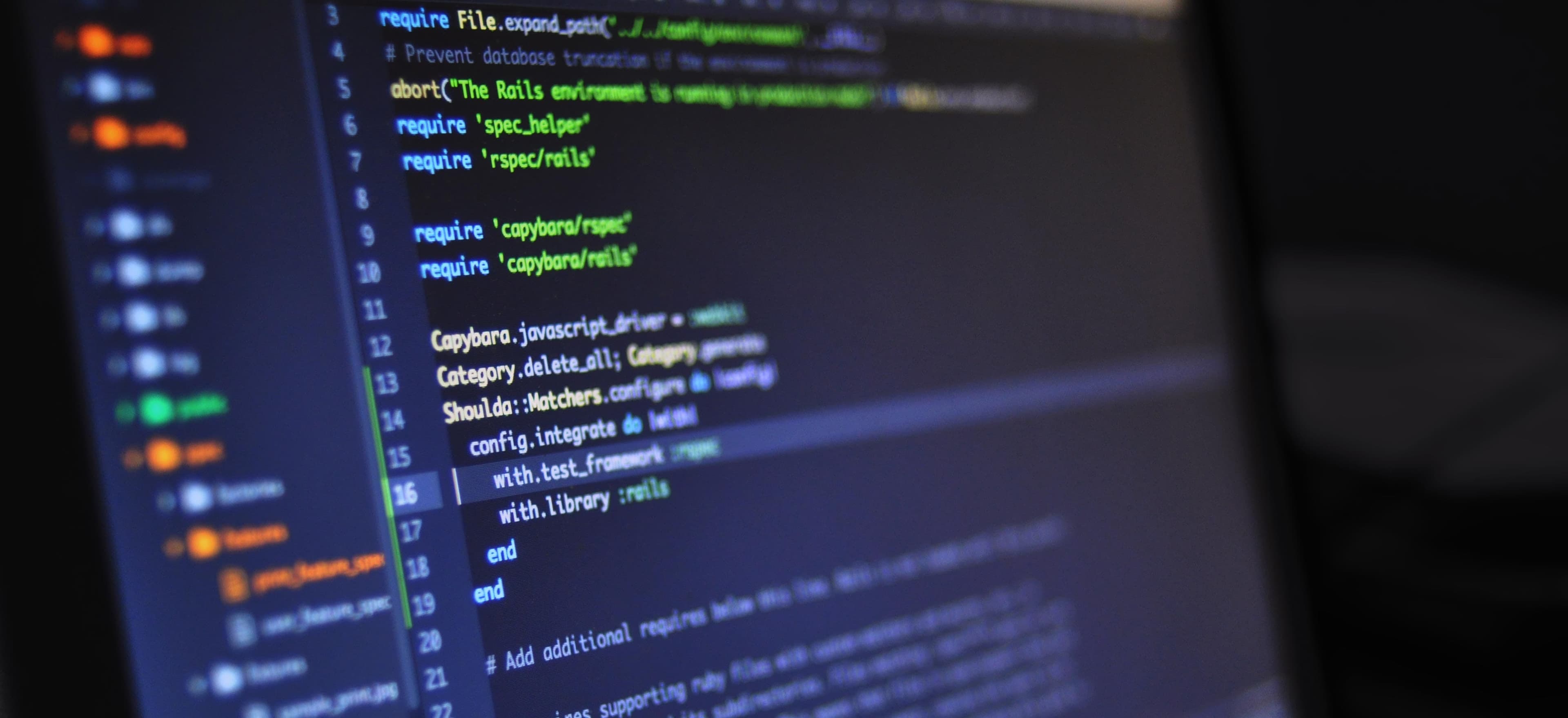
- Published on
Securing Java EE Applications from Data Theft
Java EE (Enterprise Edition) applications are often used to handle sensitive and valuable data, making them prime targets for cyber attacks. Therefore, it is crucial to implement robust security measures to protect against data theft and unauthorized access. In this article, we will explore best practices for securing Java EE applications from data theft.
1. Input Validation
One of the most common attack vectors is through unvalidated input. Attackers can inject malicious code or SQL queries through forms, URL parameters, or HTTP headers. To mitigate this risk, it is essential to validate and sanitize all user inputs.
// Example of input validation using regular expressions
String userInput = request.getParameter("input");
if (userInput.matches("^[a-zA-Z0-9]*$")) {
// Input is valid
} else {
// Input is invalid
}
By validating user inputs, we can prevent attackers from exploiting vulnerabilities such as SQL injection and cross-site scripting (XSS).
2. Authentication and Authorization
Implementing strong authentication and authorization mechanisms is critical for protecting sensitive data. Utilize frameworks like Spring Security or Java EE's built-in security features to enforce user authentication and authorization.
// Example of implementing authentication and authorization using Spring Security
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
}
By enforcing strong authentication and authorization, we can control access to sensitive data and functionalities, reducing the risk of unauthorized access.
3. Encryption
Sensitive data should be encrypted both at rest and in transit to prevent unauthorized access. Utilize strong encryption algorithms and protocols such as AES and TLS to secure data.
// Example of encrypting sensitive data using AES encryption
String originalData = "Sensitive information";
String encryptedData = AESEncryption.encrypt(originalData, secretKey);
Encrypting sensitive data ensures that even if attackers gain access to the data, it remains indecipherable and unusable.
4. Session Management
Proper session management is crucial to prevent session hijacking and unauthorized access. Use secure session tokens, employ session expiration, and implement measures to detect and prevent session fixation attacks.
// Example of setting secure session attributes
HttpSession session = request.getSession();
session.setAttribute("user", authenticatedUser);
session.setMaxInactiveInterval(1800); // Session expires after 30 minutes of inactivity
session.setAttribute("httpOnly", true); // HTTP Only attribute to prevent XSS attacks
By implementing robust session management techniques, we can minimize the risk of unauthorized access through compromised sessions.
5. Secure Configuration
Ensure that sensitive configuration parameters such as database credentials and API keys are stored securely. Avoid hardcoding sensitive information in the code and utilize secure storage mechanisms such as environment variables or configuration files with restricted access.
// Example of loading database credentials from a secure configuration file
Properties config = new Properties();
config.load(new FileInputStream("/path/to/config.properties"));
String dbUsername = config.getProperty("db.username");
String dbPassword = config.getProperty("db.password");
By securely storing configuration parameters, we can prevent unauthorized access to critical system resources.
6. Logging and Monitoring
Implement comprehensive logging and monitoring to detect suspicious activities and potential security breaches. Utilize tools like ELK stack (Elasticsearch, Logstash, Kibana) or Splunk to analyze and monitor application logs for security incidents.
// Example of logging security-related events
Logger logger = Logger.getLogger("SecurityLogger");
logger.info("User " + authenticatedUser + " attempted unauthorized access");
By logging and monitoring security events, we can proactively identify and respond to potential security threats before they escalate.
The Last Word
Securing Java EE applications from data theft requires a multi-faceted approach that encompasses input validation, authentication, encryption, session management, secure configuration, logging, and monitoring. By implementing these best practices, developers can effectively safeguard sensitive data and mitigate the risk of data theft and unauthorized access.
Remember, security is an ongoing process, and it is essential to stay updated with the latest security trends and vulnerabilities, regularly review and update security measures, and conduct periodic security audits and assessments.
For further reading, check out the OWASP (Open Web Application Security Project) Java EE Security Cheat Sheet for comprehensive guidance on securing Java EE applications.
Implementing robust security measures is not just a good practice – it is an absolute necessity in today's threat landscape. By securing Java EE applications from data theft, developers can build trust, reliability, and resilience into their applications, ensuring the protection of valuable data and the integrity of their systems.