Cybersecurity: The Art of Learning from Hacker Attacks
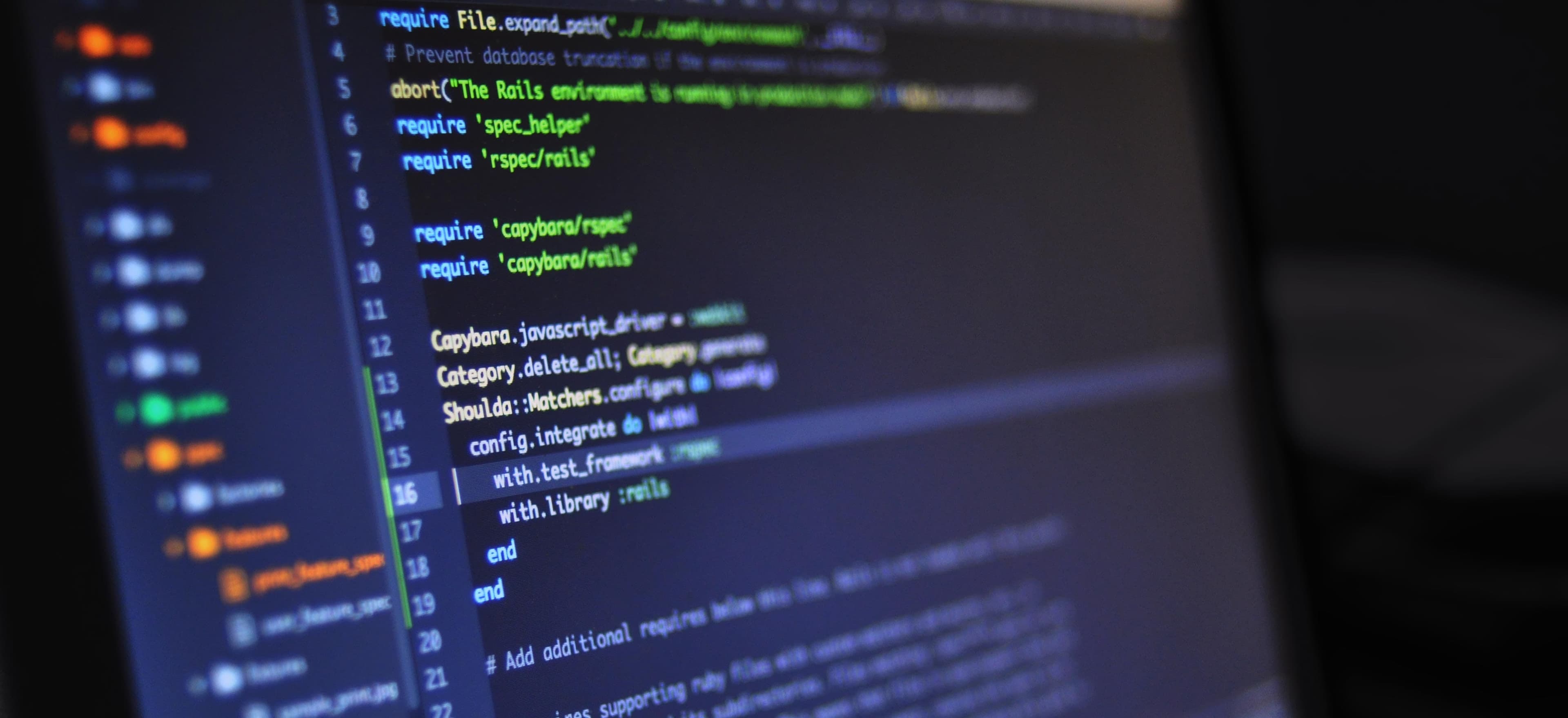
- Published on
Understanding Cybersecurity through Java
In the digital age, cybersecurity plays a critical role in safeguarding systems and data from malicious attacks. As a developer, understanding cybersecurity, and how to implement secure practices in Java, is paramount. In this blog post, we will delve into the intricacies of cybersecurity, explore common attack vectors, and discuss how Java can be used to mitigate these threats.
The Importance of Cybersecurity in Today's World
In an era where cyber threats are becoming increasingly sophisticated, the importance of cybersecurity cannot be overstated. From ransomware attacks to data breaches, the repercussions of inadequate security measures can be severe for both individuals and organizations. Therefore, a proactive approach to cybersecurity is crucial in thwarting potential threats.
Understanding Common Attack Vectors
Before delving into cybersecurity measures, it's essential to understand the common attack vectors that malicious actors exploit. Some prevalent attack vectors include:
SQL Injection
A SQL injection occurs when an attacker inserts malicious SQL code into input fields, exploiting vulnerabilities in the application's SQL database. This can lead to unauthorized access, data manipulation, and even data deletion.
Cross-Site Scripting (XSS)
XSS exploits security vulnerabilities in web applications, allowing attackers to inject malicious scripts into web pages viewed by other users. This can result in the theft of sensitive information or unauthorized access to user sessions.
Securing Java Applications Against Cyber Attacks
Java, being a popular programming language in the development of web applications and enterprise systems, offers robust tools and libraries to fortify applications against cyber threats. Let's explore some essential security practices in Java.
1. Input Validation and Sanitization
One of the primary strategies to prevent SQL injection and XSS attacks is input validation and sanitization. By using frameworks like Hibernate Validator, developers can enforce constraints on input fields, ensuring that only valid and sanitized data is processed.
// Example of using Hibernate Validator for input validation
public class User {
@NotNull
@Size(min=2, message="Name is too short")
private String name;
@Email
private String email;
// Getters and setters
}
The above code snippet demonstrates how Hibernate Validator can be used to apply validation rules to the User
class, ensuring that the name
field has a minimum length and the email
field follows the email format.
2. Cross-Origin Resource Sharing (CORS) Configuration
To mitigate cross-origin attacks, it's crucial to configure CORS settings in Java web applications. By specifying permitted origins, methods, and headers, developers can control access to resources and prevent unauthorized cross-origin requests.
// Example of CORS configuration in a Java web application
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**")
.allowedOrigins("https://trusted-domain.com")
.allowedMethods("GET", "POST", "PUT", "DELETE")
.allowedHeaders("Authorization", "Content-Type")
.exposedHeaders("Authorization");
}
}
The above Java code demonstrates how CORS settings can be configured to allow cross-origin requests only from the specified trusted domain and restrict unauthorized methods and headers.
3. Strong Encryption and Hashing
Data protection is paramount in cybersecurity. Java provides extensive support for cryptographic operations through libraries like Bouncy Castle and Java Cryptography Architecture (JCA). By utilizing strong encryption algorithms and hash functions, sensitive data can be safeguarded from unauthorized access.
// Example of using JCA for encryption and decryption
public class EncryptionUtils {
public static byte[] encryptData(String data, Key key) {
// Implementation of encryption using JCA
}
public static String decryptData(byte[] encryptedData, Key key) {
// Implementation of decryption using JCA
}
}
The above code snippet showcases the usage of JCA for encrypting and decrypting data, demonstrating the implementation of encryption operations to secure sensitive information.
Closing Remarks
In today's interconnected digital landscape, cybersecurity is a fundamental aspect of software development. By understanding common attack vectors and implementing robust security measures in Java applications, developers can fortify their systems against potential threats. From input validation to encryption, Java provides a rich set of tools to bolster cybersecurity defenses. Embracing these practices is not only essential for building secure applications but also for upholding the integrity and trust of digital ecosystems.
In conclusion, cybersecurity is not a static concept but an evolving discipline that necessitates continuous learning and adaptation. As the cybersecurity landscape continues to evolve, it's imperative for developers to stay informed about emerging threats and best practices to mitigate them effectively.
By incorporating robust security measures and staying abreast of the latest developments in cybersecurity, developers can contribute to a more secure digital environment for both businesses and end users.
Remember, cybersecurity is not just about protecting data; it's about safeguarding trust and confidence in the digital realm.
For further insight into cybersecurity practices and Java development, consider exploring OWASP and Java Security Architecture.