Data Breach Prevention: Protecting Your Valuable Information
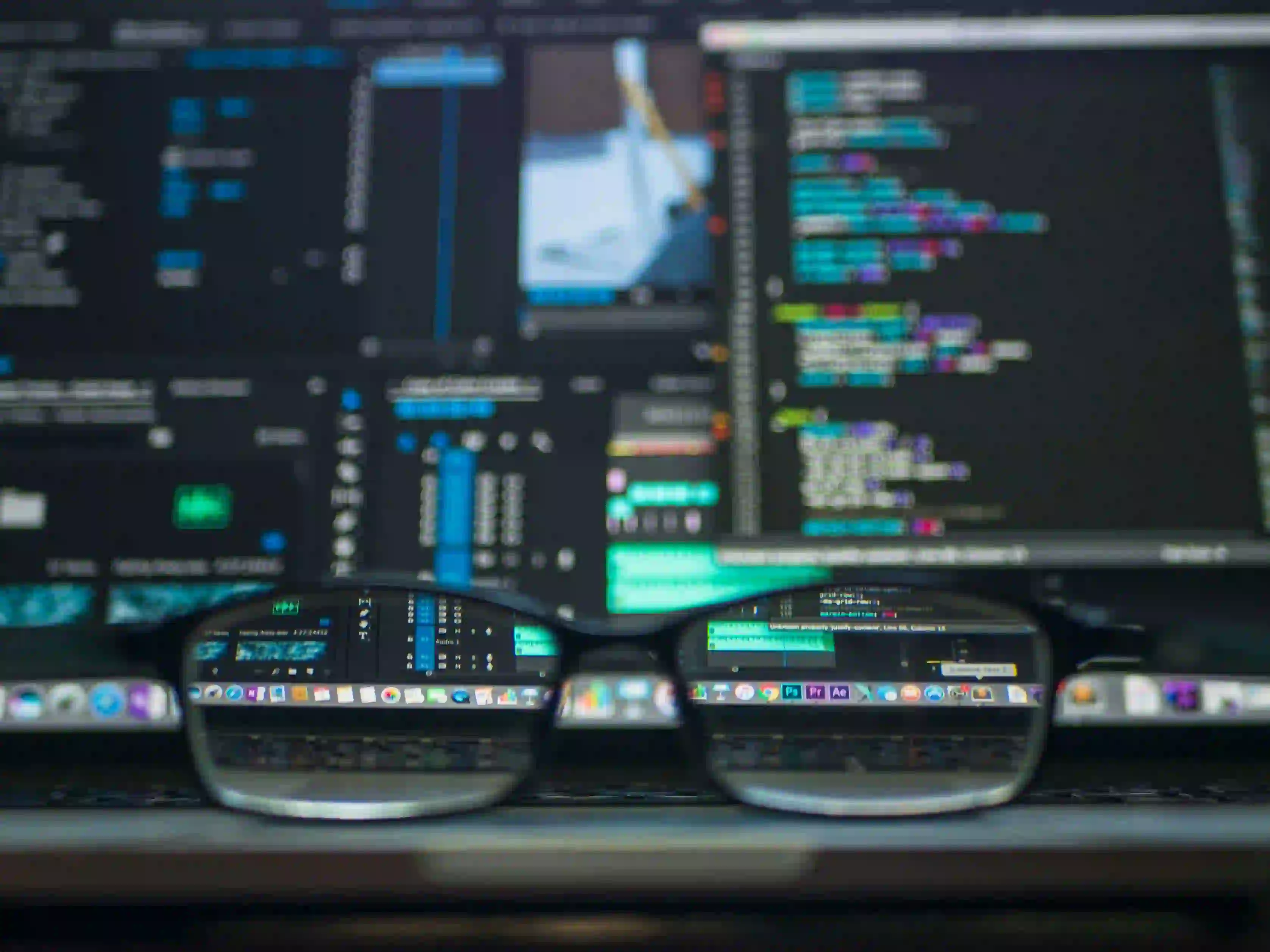
The Importance of Data Breach Prevention in Java Applications
In today's digital world, data breaches have become a common concern for businesses and individuals alike. As a Java developer, it's crucial to prioritize data security and implement measures to prevent unauthorized access to sensitive information. In this post, we'll explore the importance of data breach prevention in Java applications and discuss best practices for ensuring the security of your valuable data.
Understanding the Impact of Data Breaches
Data breaches can have far-reaching consequences for organizations, including financial losses, reputational damage, and legal implications. In the context of Java applications, a data breach can expose sensitive user data, such as usernames, passwords, and personal information. This can lead to serious repercussions for both the users and the organization responsible for safeguarding their data.
Best Practices for Data Breach Prevention in Java
1. Input Validation and Sanitization
One common entry point for attackers is through unsanitized user input. By validating and sanitizing input data, Java developers can prevent common attacks such as SQL injection and cross-site scripting (XSS). Utilizing libraries like OWASP ESAPI can assist in input validation and sanitization, reducing the risk of data breaches.
Example:
String userInput = request.getParameter("input");
// Validate and sanitize userInput
2. Secure Authentication and Authorization
Implementing robust authentication and authorization mechanisms is essential for protecting sensitive data. Utilize industry-standard protocols such as OAuth or OpenID Connect for single sign-on (SSO) functionality. Additionally, consider utilizing frameworks like Spring Security to enforce secure authentication and access control within your Java applications.
Example:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
}
3. Data Encryption
Utilize strong encryption algorithms to protect sensitive data both at rest and in transit. Java provides robust cryptographic libraries, such as Java Cryptography Architecture (JCA), for implementing encryption and decryption functionalities. Whether it's encrypting data in the database or securing communication channels, encryption plays a vital role in data breach prevention.
Example:
public class EncryptionUtil {
public static String encryptData(String data, SecretKey key) {
// Implement encryption logic using JCA
}
public static String decryptData(String encryptedData, SecretKey key) {
// Implement decryption logic using JCA
}
}
4. Proper Error Handling
Effective error handling is crucial in preventing data exposure through unintended exceptions. Implement centralized error handling mechanisms to ensure that sensitive information is not inadvertently leaked in error messages. Utilize logging frameworks like Log4j to capture and manage application errors securely.
Example:
try {
// Perform sensitive operations
} catch (Exception e) {
logger.error("An error occurred while processing the request", e);
// Handle the error gracefully
}
5. Regular Security Patching
Keep your Java environment and dependencies up to date with the latest security patches. Vulnerabilities in third-party libraries or the Java runtime itself can be exploited by attackers. Regularly monitor security advisories and update your dependencies to mitigate potential security risks.
Closing Remarks
Data breach prevention should be a top priority for Java developers, given the potential impact of security incidents on businesses and users. By incorporating robust security practices, such as input validation, secure authentication, data encryption, proper error handling, and regular patching, Java applications can significantly reduce the risk of data breaches and enhance overall security posture.
Embracing a proactive approach to data security not only safeguards sensitive information but also instills trust and confidence among users and stakeholders. As the digital landscape continues to evolve, staying vigilant and proactive in data breach prevention is vital for the long-term success of Java applications.
In conclusion, prioritizing data breach prevention in Java is not only a best practice but a fundamental responsibility for developers and organizations entrusted with sensitive information. By adhering to best practices and embracing a security-first mindset, Java developers can mitigate the risk of data breaches and uphold the integrity of their applications and the data they handle.
Remember, the security of your Java applications is not just a feature – it's a fundamental requirement.