Challenges in Analyzing Large-Scale Java Projects
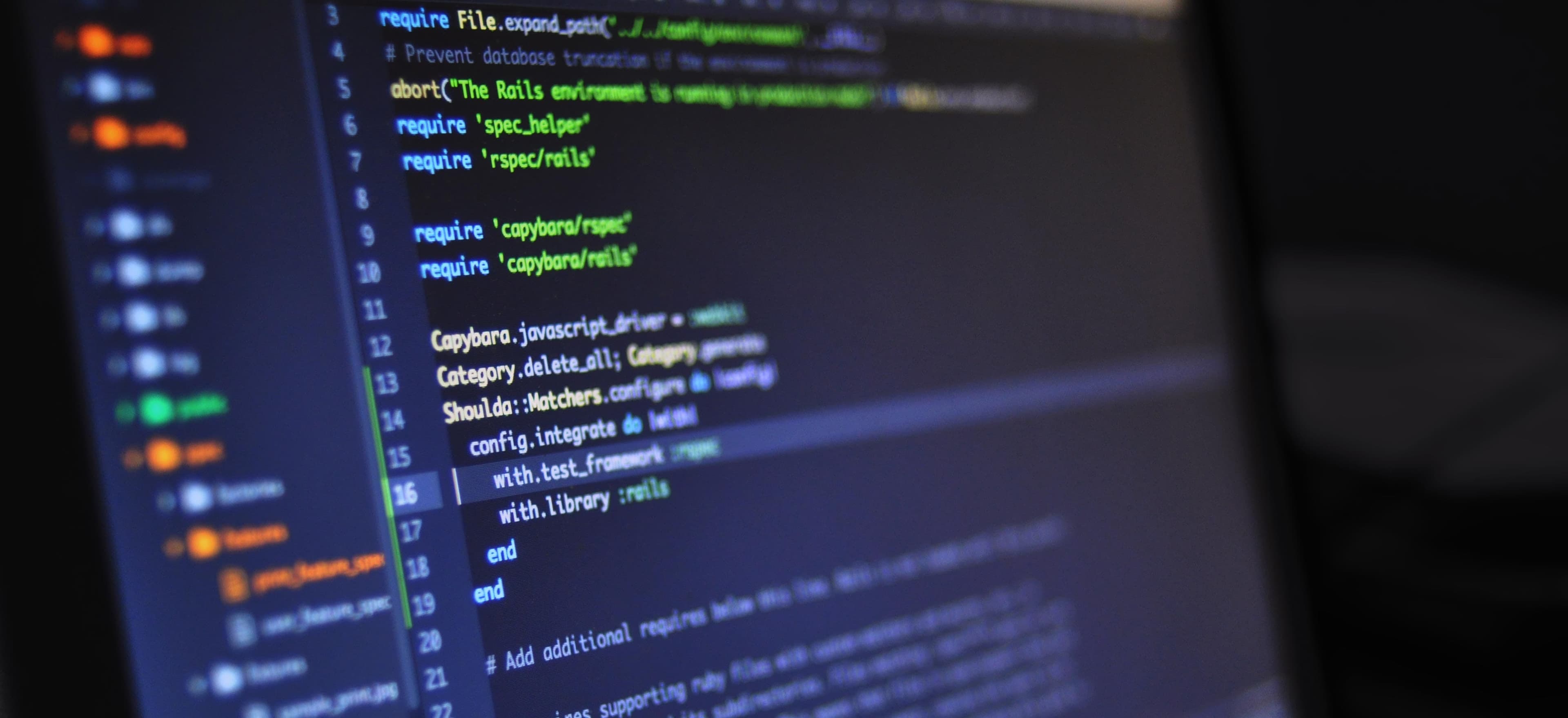
- Published on
Challenges in Analyzing Large-Scale Java Projects
Analyzing large-scale Java projects can be a daunting task, especially when dealing with thousands of classes, complex dependencies, and extensive codebases. In this blog post, we'll explore some of the challenges that developers and teams face when working with large-scale Java projects and discuss strategies and tools to mitigate these challenges.
1. Build and Dependency Management
Large-scale Java projects often have numerous dependencies, libraries, and modules, making build and dependency management a critical aspect of the development process. With a plethora of dependencies, ensuring consistent and efficient builds becomes challenging. Gradle and Maven are popular build tools in the Java ecosystem, offering powerful dependency management capabilities. Using a build tool that efficiently manages dependencies and builds can significantly streamline the development workflow for large-scale projects.
// Example of a Gradle build file
dependencies {
implementation 'com.example:library:1.0.0'
testImplementation 'junit:junit:4.12'
// More dependencies...
}
In the code snippet above, the Gradle build file defines dependencies for the project. By specifying dependencies and their versions, build tools can resolve and download the necessary libraries, simplifying the management of dependencies in large-scale projects.
2. Code Quality and Static Analysis
Maintaining code quality in large-scale Java projects is essential for long-term maintainability, readability, and extensibility. Static code analysis tools such as SonarQube, Checkstyle, and FindBugs help identify code smells, potential bugs, and adherence to coding standards.
Integrating these static analysis tools into the build process allows for continuous assessment of code quality, ensuring that the codebase remains robust and adheres to best practices.
// Example of a static code analysis configuration in a build file
plugins {
id 'java'
id 'checkstyle'
}
checkstyle {
toolVersion = "8.44"
configFile file("config/checkstyle.xml")
// More configuration...
}
The code snippet demonstrates the configuration of Checkstyle in a Gradle build file. By applying static code analysis as part of the build process, teams can proactively identify and address potential issues in the codebase, promoting overall code quality.
3. Performance and Scalability
Large-scale Java projects often face performance and scalability challenges, especially when handling substantial amounts of data or concurrent requests. Profiling tools like YourKit and VisualVM enable developers to analyze the runtime performance of their Java applications, identify bottlenecks, and optimize resource utilization.
Additionally, employing scalability patterns such as caching, load balancing, and asynchronous processing can help mitigate performance issues in large-scale Java applications, ensuring that they can effectively handle increased load and data volume.
4. Testing and Test Automation
Testing large-scale Java projects comprehensively is a complex endeavor. Unit tests, integration tests, and end-to-end tests are essential components of ensuring the correctness and reliability of the application. Test automation frameworks like JUnit, Mockito, and Selenium facilitate automated testing, allowing for thorough test coverage and rapid feedback on changes.
// Example of a JUnit test case
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
// More test cases...
}
The code snippet presents a simple JUnit test case for a Calculator
class. Automating the execution of such tests aids in maintaining the stability and functionality of large-scale Java projects, enabling confident refactoring and evolution of the codebase.
Closing the Chapter
Navigating the intricacies of large-scale Java projects demands careful consideration of build and dependency management, code quality, performance optimization, and comprehensive testing. By leveraging appropriate tools, integrating best practices, and adopting scalability patterns, teams can effectively address the challenges associated with analyzing and developing large-scale Java projects.
In this blog post, we've only scratched the surface of the complexities involved in handling large-scale Java projects. To delve deeper into these topics and explore advanced techniques, consider exploring resources such as DZone and Baeldung, which offer in-depth insights into Java development and best practices.