Fixing Mockito Error: Unable to Instantiate @InjectMocks Field
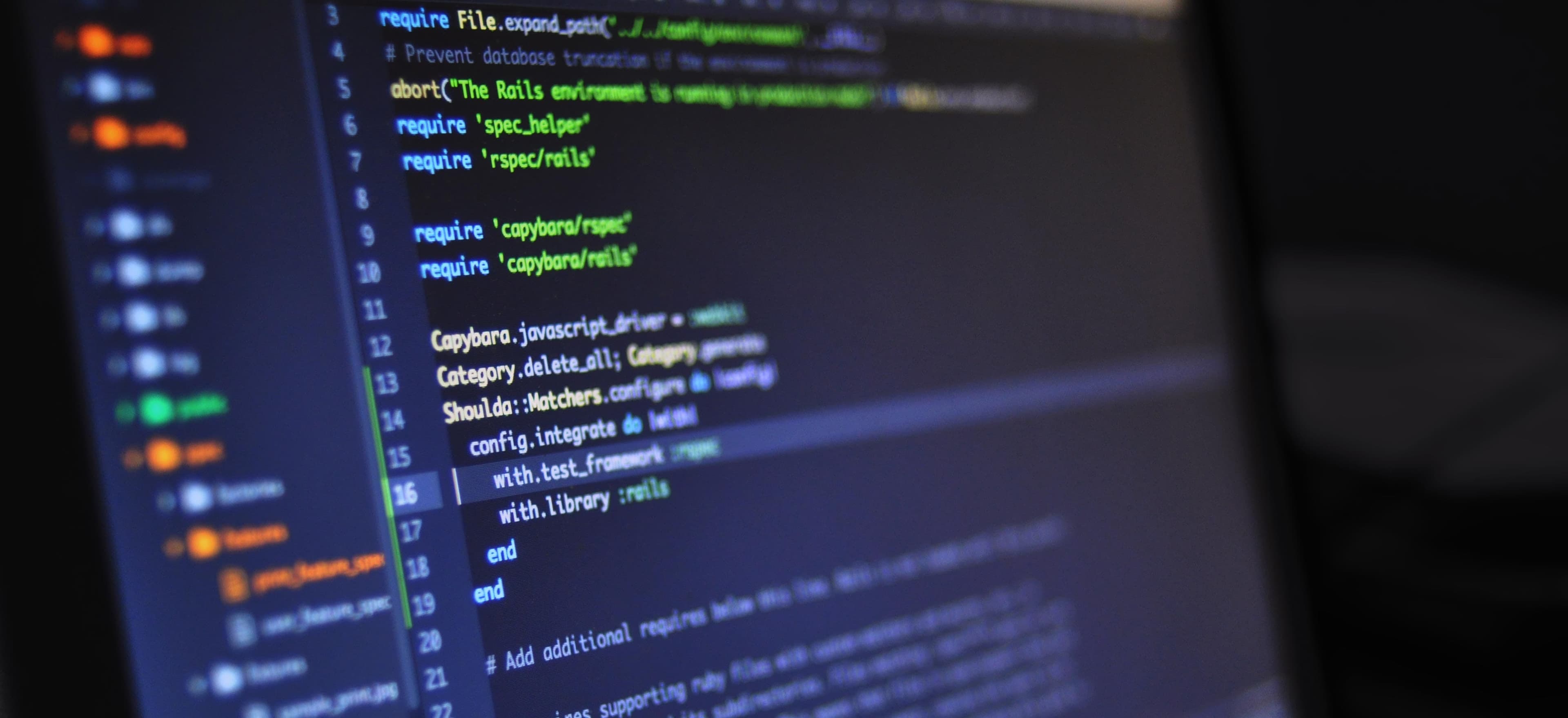
- Published on
Fixing Mockito Error: Unable to Instantiate @InjectMocks Field
Mockito is a popular framework for creating and working with mock objects in Java. It's commonly used in unit testing to isolate the code under test and simulate the behavior of dependencies. One common feature of Mockito is the @InjectMocks
annotation, which can be used to automatically inject mock objects into the fields of a test class. However, sometimes you may encounter an error stating "Unable to instantiate @InjectMocks field", which can be frustrating to deal with. In this article, we'll explore the possible causes of this error and provide solutions to fix it.
Understanding @InjectMocks
Before we delve into fixing the error, let's have a brief overview of the @InjectMocks
annotation. This annotation is used to inject dependent mock instances into the test class. When Mockito creates mocks, it can either inject them by type or by name into the test class. The @InjectMocks
annotation facilitates this process by injecting the mocks automatically.
Common Causes of the Error
The "Unable to instantiate @InjectMocks field" error can occur due to various reasons, some of which include:
-
Missing No-Argument Constructor: The target class being instantiated lacks a no-argument constructor, making it impossible for Mockito to create an instance of the class for injection.
-
Circular Dependencies: If the dependencies of the class being mocked or the test class itself create a circular dependency loop, Mockito may struggle to instantiate the mocks correctly, resulting in the error.
-
Final Classes or Methods: Mockito has some limitations when it comes to mocking final classes or methods. If the class being mocked or the methods being called are final, Mockito may not be able to instantiate them, leading to the error.
Now that we have an understanding of why this error may occur, let's explore the solutions to address each of these causes.
Fixing the Error
1. Adding a Default Constructor
If the target class lacks a no-argument constructor, one solution is to add a default constructor to the class. This allows Mockito to create an instance of the class for injection. Here's an example of how you can add a default constructor:
public class TargetClass {
public TargetClass() {
// Default constructor logic, if needed
}
// Other class methods and fields
}
2. Resolving Circular Dependencies
To address circular dependencies, consider refactoring the code to remove the circular dependencies. This may involve restructuring the classes or dependencies to break the cycle. Alternatively, you can explicitly specify the mocks and inject them using setter methods or constructor injection instead of relying solely on @InjectMocks
.
3. Dealing with Final Classes or Methods
When dealing with final classes or methods, Mockito provides a way to mock them using the Mockito.spy()
method. By using this approach, you can partially mock the final class or method and avoid the instantiation issues. Here's an example of how you can use Mockito.spy()
:
FinalClass finalInstance = new FinalClass();
FinalClass spiedFinalInstance = Mockito.spy(finalInstance);
By applying these solutions, you can effectively address the "Unable to instantiate @InjectMocks field" error and ensure that your Mockito tests run smoothly.
Closing the Chapter
In this article, we've discussed the @InjectMocks
annotation in Mockito, the common causes of the "Unable to instantiate @InjectMocks field" error, and the corresponding solutions to fix it. By understanding the underlying reasons for the error and applying the appropriate fixes, you can overcome this issue and enhance the robustness of your unit tests using Mockito.
While Mockito is a powerful tool for creating mock objects, it's essential to be aware of its limitations and know how to troubleshoot errors that may arise. With a solid understanding of Mockito's features and how to address potential issues, you can leverage it effectively in your Java projects.
Remember, Mockito is just one aspect of Java unit testing. It's always beneficial to have a comprehensive understanding of various testing frameworks and methodologies. If you're interested in diving deeper into the world of Java testing, be sure to check out our guide on Improving Java Unit Tests for additional insights.
Happy testing!