Spring MVC: Managing Domain Objects Efficiently
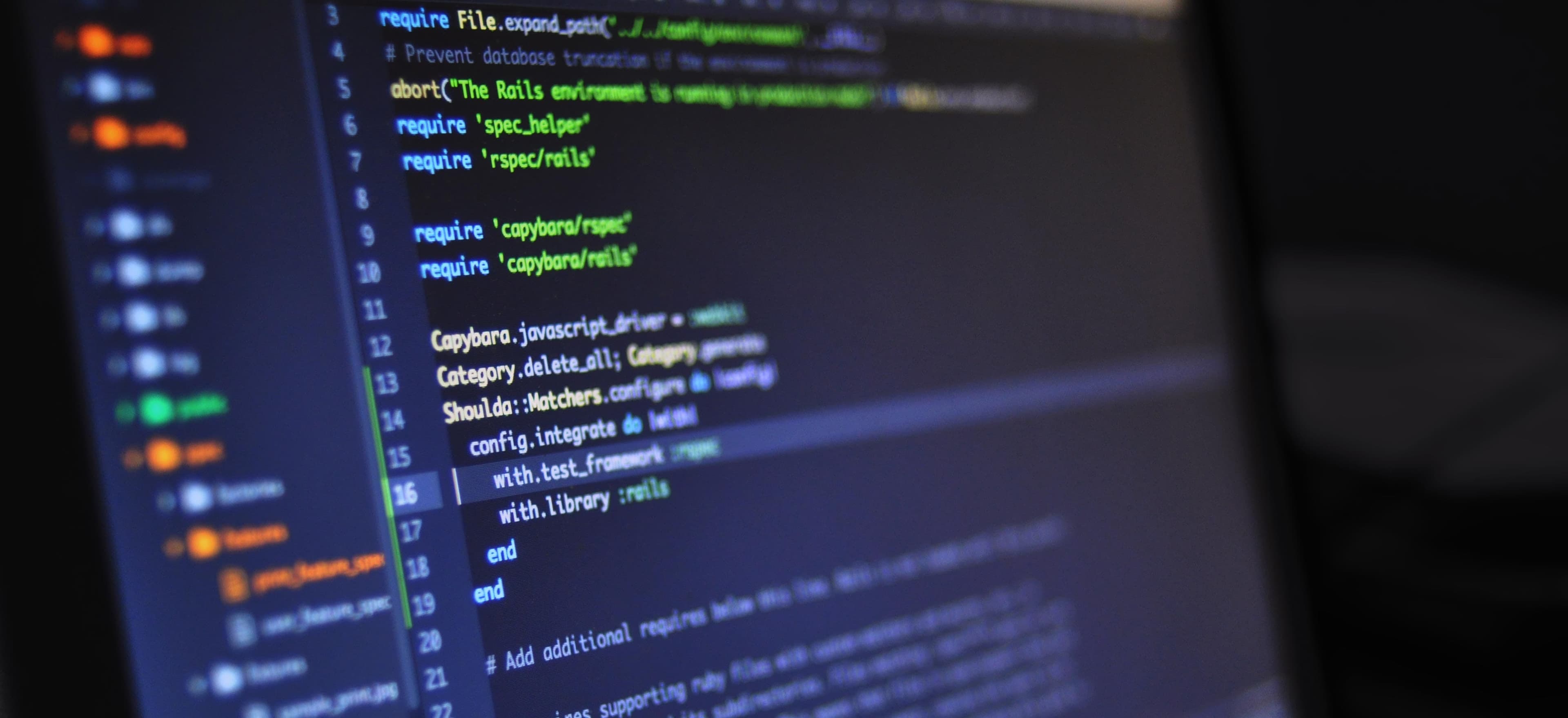
- Published on
Spring MVC: Managing Domain Objects Efficiently
When developing a web application using Spring MVC, efficient management of domain objects is crucial for creating a scalable, maintainable, and performant system. In this article, we will explore best practices for managing domain objects in Spring MVC, including domain object design, data validation, and persistence.
Designing Domain Objects
1. Encapsulate Business Logic
When designing domain objects, it's important to encapsulate business logic within the domain classes. This promotes the concept of object-oriented programming and ensures that each domain object is responsible for its own behavior. For example, if you have a User
domain object, it should contain methods for user-related operations such as register
, login
, and updateProfile
.
public class User {
private String username;
private String password;
public boolean authenticate(String enteredPassword) {
return this.password.equals(enteredPassword);
}
// Other methods
}
2. Use Data Transfer Objects (DTOs)
When working with Spring MVC, it's common to use Data Transfer Objects (DTOs) to transfer data between the client and the server. DTOs are lightweight objects that contain only the necessary data to transfer between layers of the application. This helps in reducing the amount of data being transferred over the network and prevents over-fetching of data.
public class UserDTO {
private String username;
private String email;
// Getters and setters
}
3. Validation in Domain Objects
Domain objects should also handle data validation to ensure that only valid data is persisted. Spring provides validation support through the javax.validation
package and annotations such as @NotNull
, @Size
, and @Email
. By annotating fields in the domain objects, you can perform declarative validation.
public class User {
@NotNull
@Size(min=6, max=20)
private String username;
@NotNull
@Email
private String email;
// Other fields
}
Managing Persistence
1. Use Spring Data JPA for Easy Data Access
Spring Data JPA is a powerful feature of the Spring Framework that provides a more concise and fluent way to access the database. By defining interfaces that extend JpaRepository
, you can automatically generate the necessary CRUD (Create, Read, Update, Delete) methods for your domain objects.
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
// Other custom queries
}
2. Optimistic Locking
Optimistic locking is a strategy for handling concurrent updates to the same record in the database. Spring Data JPA provides support for optimistic locking through the use of the @Version
annotation on a field in the domain object. When an update is performed, Spring Data JPA automatically checks the version to prevent conflicting updates.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Version
private Long version;
// Other fields
}
3. Managing Transactions
In Spring MVC, managing transactions is essential for ensuring data integrity. By annotating service methods with @Transactional
, you can define the scope of a transaction. This ensures that a series of database operations either complete entirely or are rolled back if an error occurs.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void updateUser(UserDTO userDTO) {
User user = userRepository.findByUsername(userDTO.getUsername());
// Update user
}
}
Final Considerations
Efficient management of domain objects is fundamental to the success of a Spring MVC application. By following best practices in domain object design, data validation, and persistence management, you can create a robust and scalable system. Utilizing features such as Spring Data JPA, optimistic locking, and transaction management further enhances the efficiency and reliability of the application.
In summary, a well-designed domain model, combined with effective persistence management, lays the foundation for a high-performing and maintainable Spring MVC application.
To read more about Spring MVC and its features, check out the official documentation for detailed insights.
Start implementing these best practices in your Spring MVC project and witness the significant boost in performance and maintainability. Happy coding!