Why JSON and XML Fall Short for Internal Data Transfers
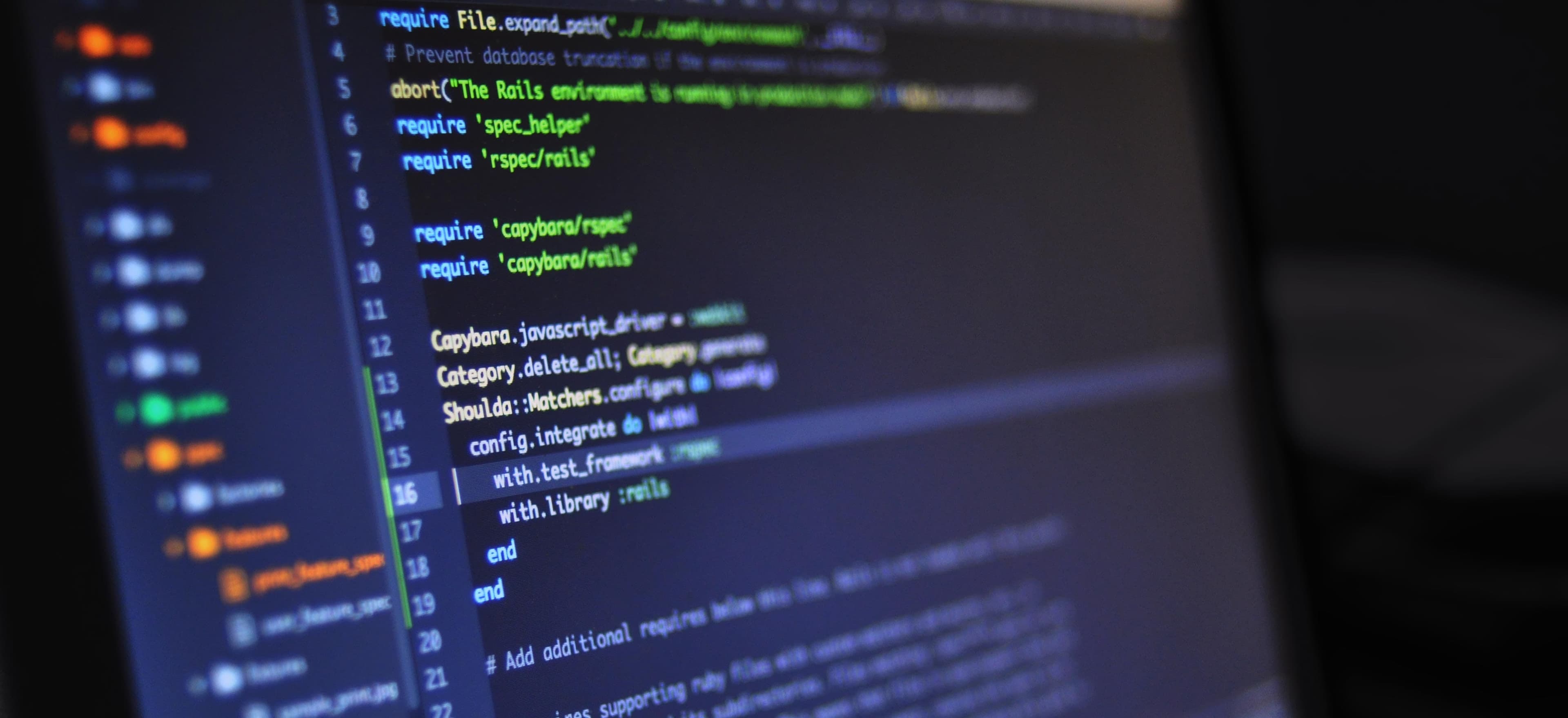
- Published on
Why JSON and XML Fall Short for Internal Data Transfers
In an era where data is dubbed the "new oil," the backbone of effective data management lies in how we transfer and store this information. Among the various data formats available, JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are the giants, widely adopted due to their flexibility and usability. However, they face inherent limitations when used for internal data transfers in modern applications. In this blog post, we will explore these limitations, delve into potential solutions, and offer a look at more effective alternatives.
Understanding JSON and XML
Before we dive into their shortcomings, let's briefly understand what JSON and XML are.
JSON
JSON is a lightweight data interchange format, easy for human reading and writing. It is primarily used in web applications to transmit data between a server and a web client.
Example of JSON:
{
"user": {
"name": "John Doe",
"age": 30,
"email": "johndoe@example.com"
}
}
XML
XML was designed to store and transport data, emphasizing both the readability of data by humans and machines. It allows users to define their own tags, making it versatile but also verbose.
Example of XML:
<user>
<name>John Doe</name>
<age>30</age>
<email>johndoe@example.com</email>
</user>
Why JSON and XML Aren't Ideal for Internal Data Transfers
While JSON and XML have their advantages, they also exhibit notable deficiencies for internal data transport, particularly as applications scale up. Here are several reasons why they may fall short.
1. Data Overhead
Both JSON and XML carry significant data overhead. XML, in particular, can be verbose due to its use of opening and closing tags, making the transmitted data bulkier.
Solution: Protobuf (Protocol Buffers)
Protocol Buffers, or Protobuf, developed by Google, offers a more efficient alternative by encoding data in a binary format, resulting in reduced data size.
Example of Protobuf:
message User {
required string name = 1;
required int32 age = 2;
required string email = 3;
}
This compact structure leads to higher performance and lower storage costs. Learn more about Protobuf here.
2. Parsing Complexity
Another downside is the parsing complexity involved with JSON and XML. Both formats demand a significant amount of processing power to decode and encode data, especially when compared to binary formats.
Solution: MessagePack
MessagePack is a binary format that is both compact and efficient. It allows for fast serialization and deserialization.
import org.msgpack.core.MessagePack;
import org.msgpack.core.MessageUnpacker;
import org.msgpack.core.MessagePacker;
// Serialization
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
MessagePacker packer = MessagePack.newDefaultPacker(byteArrayOutputStream);
packer.packString("John Doe")
.packInt(30)
.packString("johndoe@example.com");
packer.close();
// Deserialization
byte[] bytes = byteArrayOutputStream.toByteArray();
MessageUnpacker unpacker = MessagePack.newDefaultUnpacker(bytes);
String name = unpacker.unpackString();
int age = unpacker.unpackInt();
String email = unpacker.unpackString();
This approach significantly simplifies both the data transfer and the parsing processes.
3. Lack of Strong Typing
JSON lacks strong typing, making data validation and error-checking challenging. The absence of a schema can lead to unpredictable behavior and potential runtime errors.
Solution: Avro
Apache Avro offers a dynamic schema that provides a strong typing system.
{
"type": "record",
"name": "User",
"fields": [
{"name": "name", "type": "string"},
{"name": "age", "type": "int"},
{"name": "email", "type": "string"}
]
}
With Avro, data consistency remains intact across applications and environments. Check Avro Schema documentation for further information.
4. Compatibility Issues
Different programming languages manage JSON and XML in varied ways, resulting in compatibility issues during data exchange.
Solution: FlatBuffers
FlatBuffers offers cross-platform data serialization, providing consistent data structure irrespective of the programming language used.
// FlatBufferSchema Example
table User {
name:string;
age:int;
email:string;
}
FlatBuffers is especially helpful in gaming and mobile applications where performance and efficiency are critical. For further details on FlatBuffers, you can visit FlatBuffers Documentation.
5. Inefficient Handling of Large Data Sets
Both JSON and XML struggle when dealing with large volumes of data, resulting in slower processing times.
Solution: CBOR (Concise Binary Object Representation)
CBOR is designed to be efficient in both size and parsing speed for large data sets, thus providing a better choice for handling substantial amounts of data.
// Pseudo Code for CBOR Serialization
CBORObject user = CBORObject.NewMap();
user.Add("name", "John Doe");
user.Add("age", 30);
user.Add("email", "johndoe@example.com");
By improving performance on data-rich environments, CBOR presents itself as an attractive alternative. For more detailed information on CBOR, check out CBOR Official Documentation.
A Final Look
While JSON and XML have established themselves as reliable standards for data transfer, they bear limitations that can hinder internal data exchange in growing applications. From their verbose nature to parsing complexities and lack of strong typing, these formats may not meet the demands of modern data management.
The solutions presented—Protobuf, MessagePack, Avro, FlatBuffers, and CBOR—offer more efficient, versatile, and performant alternatives for internal data transfers.
As technology evolves, so should our approaches to data management. By understanding the limitations of JSON and XML, and exploring better alternatives, you can ensure that your data transport mechanisms are robust, efficient, and scalable.
Additional Resources
- JSON Official Site
- XML Official Site
- Protocol Buffers Documentation
- MessagePack Documentation
- Apache Avro
- FlatBuffers Documentation
- CBOR Specification
Utilizing the right data transfer format can make all the difference in building efficient applications. Choose wisely!