Understanding Java 8 Sorting Performance
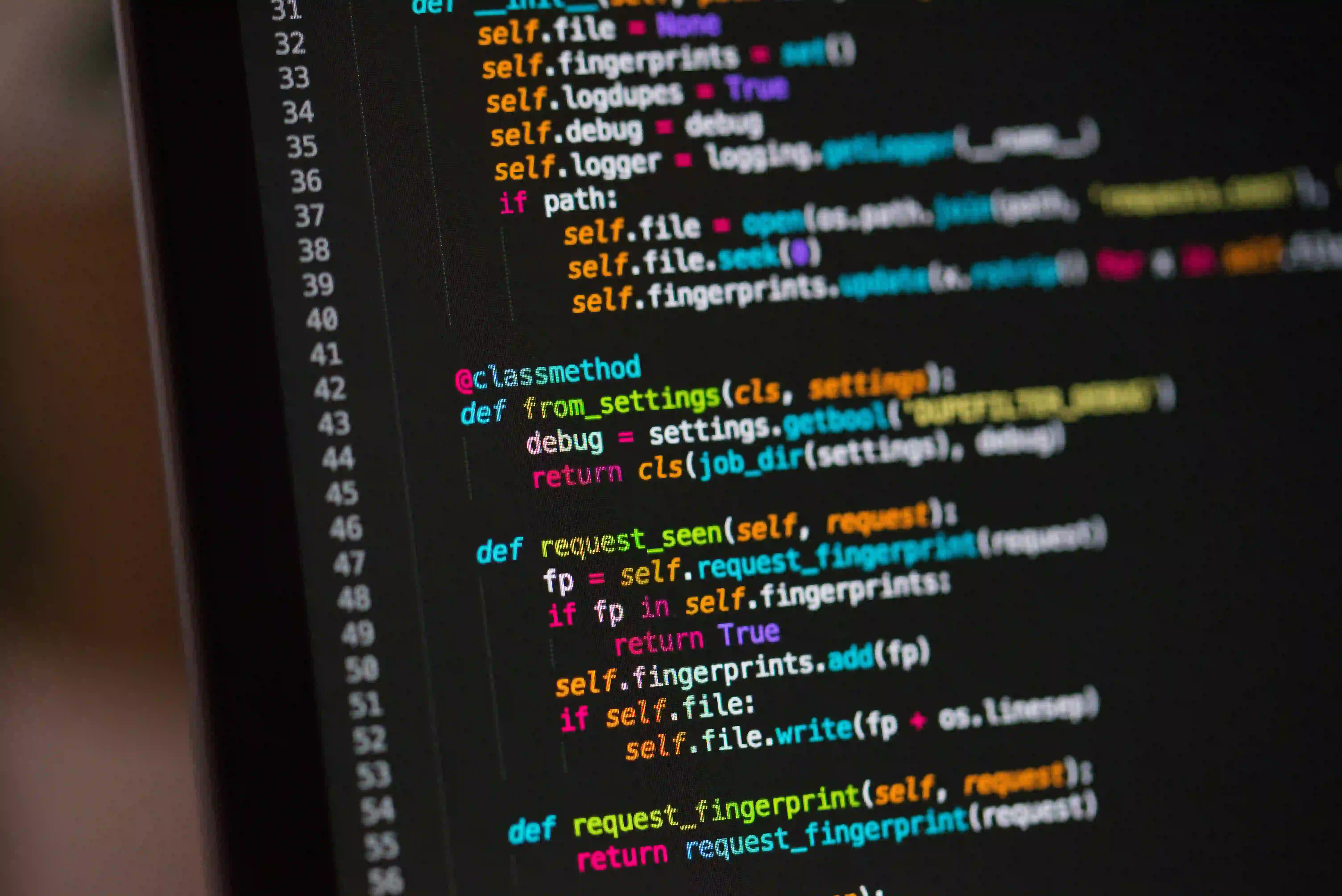
In the world of Java programming, efficiency and performance are crucial considerations. When it comes to sorting data, the Java platform offers various methods and techniques for achieving this task. In this article, we will delve into the performance aspects of sorting in Java 8, exploring different sorting algorithms and their impact on the runtime. By understanding these intricacies, you can make informed decisions about which sorting approach to employ in your Java applications.
Why Focus on Java 8?
Java 8 introduced several enhancements, including the Stream API and the java.util.stream package, which revolutionized data processing and manipulation. The Stream API provides a functional approach to processing collections, enabling concise and expressive code for operations such as filtering, mapping, and sorting. This article centers on Java 8 as it represents a significant milestone in the evolution of Java.
The Benchmarking Process
Before diving into specific sorting algorithms and their performance characteristics, it's crucial to outline the benchmarking process. Benchmarking allows us to evaluate the efficiency of different sorting techniques by comparing their execution times. In this article, we will employ the System.nanoTime()
method to measure the execution time of each sorting algorithm.
Now, let's shift our focus to some popular sorting algorithms and assess their performance in Java 8.
1. Arrays.sort()
The Arrays.sort()
method, which uses a tuned quicksort algorithm, represents one of the most commonly used sorting techniques in Java. It offers efficient sorting for arrays of primitive types and objects. Let's take a look at a simple example of using Arrays.sort()
to sort an array of integers:
int[] numbers = { 5, 2, 8, 3, 9, 4 };
Arrays.sort(numbers);
System.out.println(Arrays.toString(numbers));
In this example, the Arrays.sort()
method organizes the elements of the numbers
array in ascending order. The simplicity and built-in nature of this method make it a popular choice for many Java developers. The tuned quicksort algorithm, combined with the optimizations in the Java platform, contributes to its efficient performance.
2. Stream.sorted()
Java 8 introduced the stream-based approach to sorting through the sorted()
method, which is part of the Stream API. Let's consider an example that demonstrates sorting a list of strings in descending order using the stream-based sorting approach:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> sortedNames = names.stream()
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList());
System.out.println(sortedNames);
The sorted()
method, in conjunction with the Comparator.reverseOrder()
, efficiently sorts the list of strings in descending order. The Stream API provides a versatile and expressive way to handle sorting operations, making it a powerful addition to the Java ecosystem.
Benchmarking the Sorting Algorithms
To assess the performance of sorting algorithms, we can benchmark the Arrays.sort()
method and the stream-based sorting approach using different data set sizes. This enables us to observe how the algorithms scale with larger datasets.
Let's conduct a benchmark by comparing the performance of Arrays.sort()
and Stream.sorted()
with varying sizes of input data:
int[] largeArray = new Random().ints(100_000).toArray();
long arraySortTime = System.nanoTime();
Arrays.sort(largeArray);
arraySortTime = System.nanoTime() - arraySortTime;
System.out.println("Arrays.sort() time: " + arraySortTime + " ns");
List<Integer> largeList = new Random().ints(100_000).boxed().collect(Collectors.toList());
long streamSortTime = System.nanoTime();
List<Integer> sortedList = largeList.stream().sorted().collect(Collectors.toList());
streamSortTime = System.nanoTime() - streamSortTime;
System.out.println("Stream.sorted() time: " + streamSortTime + " ns");
By benchmarking the sorting algorithms with varied input sizes, we can gain insights into their performance characteristics and efficiency in handling larger datasets. This empirical approach empowers us to make informed decisions based on real-world scenarios.
A Final Look
In the realm of Java programming, understanding the performance of sorting algorithms is essential for crafting efficient and responsive applications. Java 8 introduced powerful features, such as the Stream API, which revolutionized the way sorting is performed. By benchmarking and comparing sorting techniques, we can make informed decisions about which approach to utilize based on the specific requirements and constraints of our applications.
With a solid grasp of the performance aspects of sorting in Java 8, developers can optimize their code to deliver exceptional user experiences and ensure smooth, performant application operations.
In conclusion, the performance of sorting algorithms in Java 8 should not be overlooked, as it can significantly impact the overall responsiveness and efficiency of Java applications. By leveraging the capabilities offered by Java 8 and understanding the intricacies of sorting algorithms, developers can elevate the performance of their applications to new heights.
Now, armed with this knowledge, go forth and optimize your sorting algorithms in Java 8 with confidence!