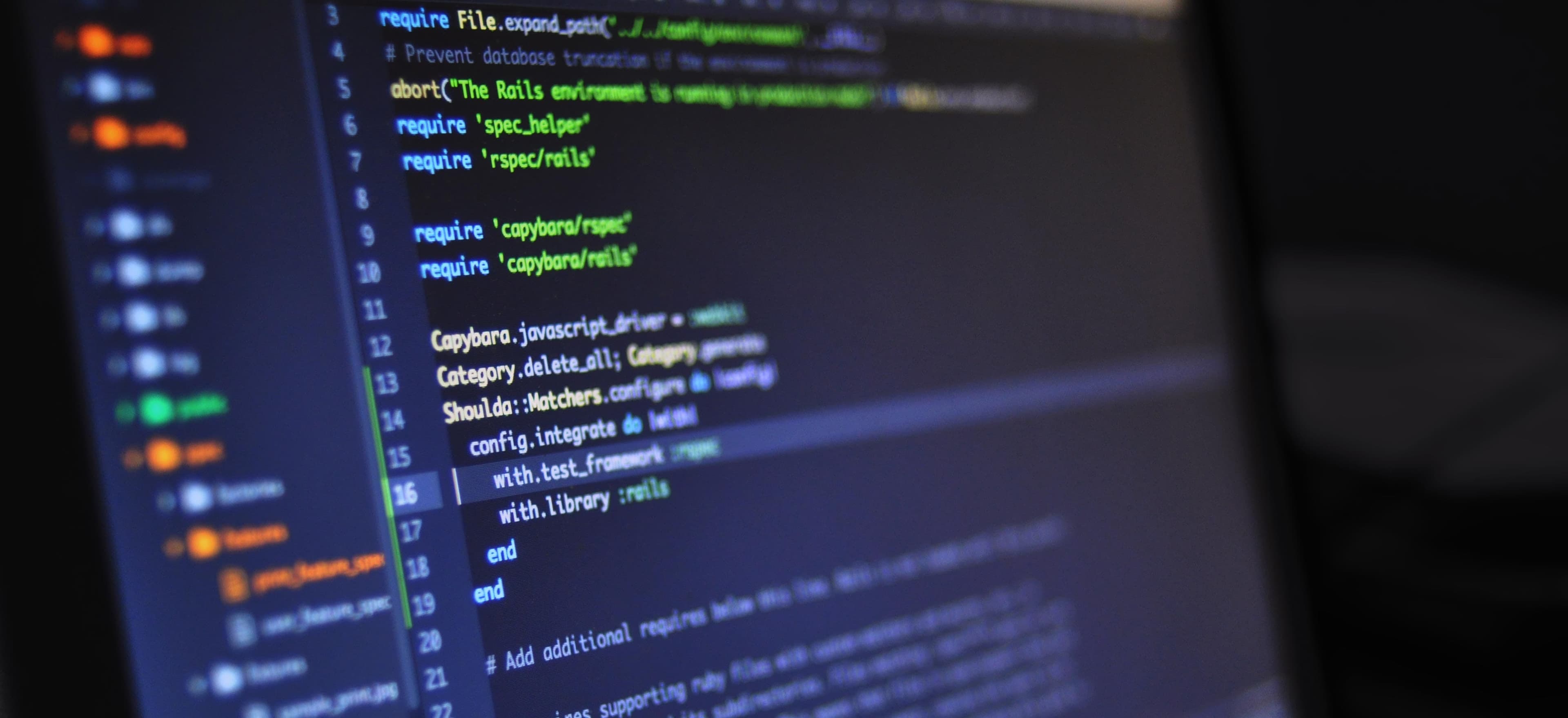
- Published on
Building Responsive UIs Using ConstraintLayout
In the fast-paced world of app development, creating user interfaces (UIs) that adapt seamlessly to various screen sizes and orientations is crucial. This is where ConstraintLayout, a powerful layout manager for Android, comes into play. In this guide, we'll explore how to leverage ConstraintLayout to build responsive UIs that look and behave consistently across different devices.
What is ConstraintLayout?
ConstraintLayout is a flexible Android layout that allows you to create large and complex layouts with a flat view hierarchy, making it efficient and easy to use. It enables you to define the position and size of each view using constraints, which are connections between the edges of the view and its parent or other views. This allows for complex responsive UI designs without the need for nested view groups.
Why Use ConstraintLayout for Responsive UIs?
More Flexible than Other Layouts
Traditional layouts like RelativeLayout and LinearLayout are often limited in their ability to handle complex UI designs and responsive behaviors. ConstraintLayout, on the other hand, provides a more flexible and intuitive way to create responsive UIs without deeply nested view hierarchies.
Simplified UI Hierarchies
By using ConstraintLayout, you can avoid deeply nested view hierarchies, resulting in more efficient UI rendering and improved performance.
Improved Performance
ConstraintLayout is designed to be efficient in terms of layout rendering and performance, making it an ideal choice for building responsive UIs that perform well on a variety of devices.
Responsive Behavior
With ConstraintLayout, you can easily define responsive behaviors for your UI elements, ensuring that they adapt seamlessly to different screen sizes and orientations.
Building Responsive UIs with ConstraintLayout
Now, let's dive into the practical aspects of building responsive UIs using ConstraintLayout.
1. Adding ConstraintLayout to Your Project
To start using ConstraintLayout in your Android project, you need to make sure that it's included in your project's dependencies. In your app's build.gradle
file, add the following dependency:
implementation 'androidx.constraintlayout:constraintlayout:2.1.0'
2. Creating a Simple ConstraintLayout
Let's begin by creating a simple layout using ConstraintLayout. In your layout XML file, replace the root layout element with ConstraintLayout:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- Your UI elements go here -->
</androidx.constraintlayout.widget.ConstraintLayout>
3. Adding Views with Constraints
Now, let's add some views to the layout and define their constraints. For example, let's add a TextView and an EditText and constrain them within the ConstraintLayout:
<TextView
android:id="@+id/titleTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Title"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
<EditText
android:id="@+id/inputEditText"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintStart_toEndOf="@id/titleTextView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="@id/titleTextView"/>
In the above example, the EditText is constrained to start right after the title, filling the remaining space until the end of the parent, and aligned with the top of the title.
4. Using Guidelines for Responsive Layouts
ConstraintLayout provides a feature called guidelines, which can be very helpful in creating responsive layouts. Guidelines are vertical or horizontal lines that you can position relative to the layout, making it easier to create consistent spacing and alignments across different screen sizes.
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_percent="0.5"/>
In this example, a horizontal guideline is positioned at 50% of the layout's height.
5. Using Chains for Flexible Arrangements
Chains are a powerful feature of ConstraintLayout that allow you to create flexible arrangements of views. By creating horizontal or vertical chains, you can control how views are distributed and aligned within the chain, providing responsive behavior across different screen sizes.
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Text 1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Text 2"
app:layout_constraintStart_toEndOf="@id/textView1"
app:layout_constraintTop_toTopOf="@id/textView1"/>
By creating a horizontal chain between the two TextViews, you can ensure that they are dynamically spaced and aligned based on the available space.
6. Handling Different Screen Sizes and Orientations
In ConstraintLayout, you can use the layout_constraintDimensionRatio
attribute to create views that maintain a specific aspect ratio, regardless of the screen size or orientation. This is particularly useful for images and media elements that need to be displayed consistently across devices.
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="0dp"
android:scaleType="centerCrop"
app:layout_constraintDimensionRatio="16:9"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
The above ImageView will maintain a 16:9 aspect ratio, adapting seamlessly to different screen sizes and orientations.
Lessons Learned
ConstraintLayout is an essential tool for building responsive UIs in Android app development. Its flexibility and powerful features allow you to create complex layouts that adapt seamlessly to different screen sizes and orientations, all while maintaining a simple view hierarchy and improving performance. With the techniques and best practices discussed in this guide, you can take full advantage of ConstraintLayout to create intuitive and responsive user interfaces for your Android apps.
Mastering the art of responsive UI design with ConstraintLayout can greatly enhance the user experience across a diverse range of Android devices, making your apps more versatile and user-friendly.
Remember, the key to successful responsive UI design lies in understanding the various features and capabilities of ConstraintLayout and leveraging them to create visually appealing and functional interfaces for your users.
So go ahead, unleash the power of ConstraintLayout and build responsive UIs that shine on any Android device!
To learn more about ConstraintLayout and dive deeper into its advanced features, check out the official Android Developers documentation.
Happy coding!