Optimizing Test Processes for Agile Transition
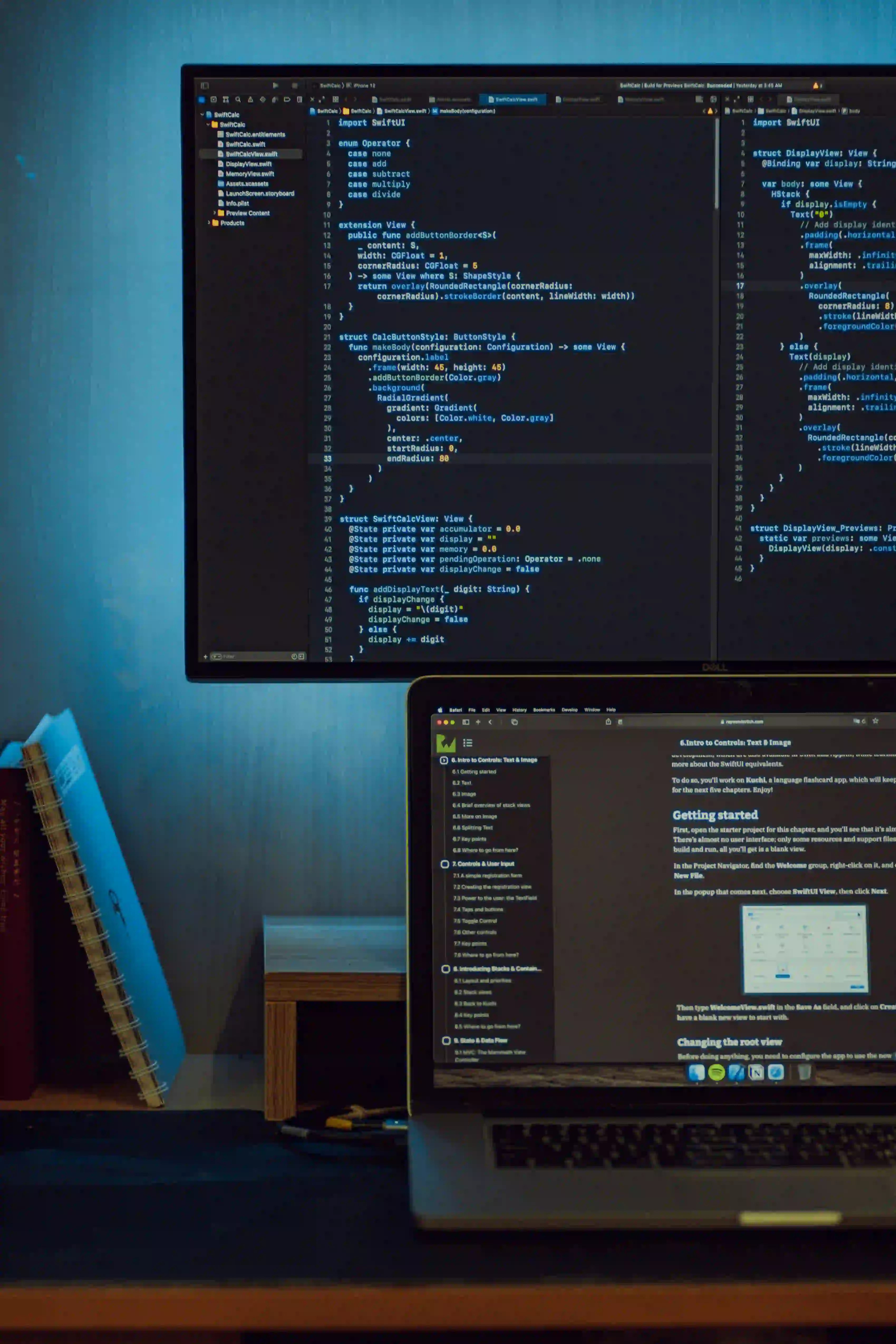
Optimizing Test Processes for Agile Transition
In the world of software development, the Agile methodology has become the gold standard for its emphasis on iterative development, collaboration, and customer feedback. However, transitioning to Agile can pose challenges, particularly when it comes to testing processes. In this article, we'll explore how to optimize test processes for an Agile transition, focusing on the use of Java as the programming language for test automation.
The Role of Testing in Agile
In Agile development, testing plays a crucial role in ensuring that each iterative cycle delivers high-quality software. Test automation, in particular, is vital for maintaining the rapid pace of Agile development while ensuring that software quality is not compromised.
Choosing Java for Test Automation
Java is a preferred language for test automation due to its platform independence, mature ecosystem, and extensive testing libraries such as JUnit and TestNG. Additionally, Java's object-oriented nature makes it particularly well-suited for creating maintainable and scalable test suites.
Optimizing Test Processes with Java
1. Leveraging JUnit for Test Case Management
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MyUnitTest {
@Test
public void testSomething() {
assertEquals(2, 1 + 1);
}
}
Why Java and JUnit?
JUnit is a popular testing framework for Java, providing annotations for test methods, assertions for test validations, and test runners for execution. By utilizing JUnit, test case management becomes more streamlined and efficient.
2. Implementing Page Object Model for UI Testing
public class LoginPage {
private WebDriver driver;
By usernameField = By.id("username");
By passwordField = By.id("password");
By loginButton = By.id("loginButton");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
driver.findElement(usernameField).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(passwordField).sendKeys(password);
}
public void clickLogin() {
driver.findElement(loginButton).click();
}
}
Why Page Object Model?
The Page Object Model (POM) pattern enhances test maintenance and readability by encapsulating the functionality of the web pages. This approach reduces code duplication and allows for easy updates when the UI changes.
3. Integrating TestNG for Enhanced Test Configuration
import org.testng.annotations.Test;
public class TestNGExample {
@Test(groups = { "fast" })
public void aFastTest() {
// Test logic
}
@Test(groups = { "slow" })
public void aSlowTest() {
// Test logic
}
}
Why TestNG?
TestNG offers additional features such as flexible test configuration, parallel test execution, and detailed reporting. By incorporating TestNG into the testing process, teams can achieve greater control and visibility over their test suites.
4. Employing Maven for Build Automation
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-project</artifactId>
<version>1.0.0</version>
<dependencies>
<!-- Test dependencies -->
</dependencies>
<build>
<plugins>
<!-- Maven plugins -->
</plugins>
</build>
</project>
Why Maven?
Maven simplifies the build process, manages dependencies, and facilitates project structuring. By using Maven, teams can streamline the build automation for their Java-based test projects.
5. Utilizing Continuous Integration with Jenkins
By integrating the test automation process within a continuous integration (CI) system like Jenkins, teams can achieve automated build and test execution, ensuring that any code changes are continuously validated.
My Closing Thoughts on the Matter
Optimizing test processes for Agile transition is essential for maintaining the quality and speed of software delivery. By leveraging Java for test automation and adopting best practices and frameworks such as JUnit, TestNG, and Maven, teams can streamline their testing processes and ensure a smooth transition to Agile development.
Remember, the ultimate goal of test process optimization is to enable Agile teams to deliver high-quality software at a rapid pace, and Java, with its rich ecosystem and robust testing capabilities, is an ideal choice for achieving this goal.
By implementing these strategies and tools, teams can effectively navigate the challenges of Agile transition and position themselves for success in the dynamic world of software development.
To delve deeper into Agile testing and Java automation, you can explore the following resources:
- JUnit Official Website
- TestNG Official Website
- Maven Official Website
- Selenium WebDriver
- Jenkins Official Website