Common Pitfalls to Avoid When Using Java 16 Stream.toList()
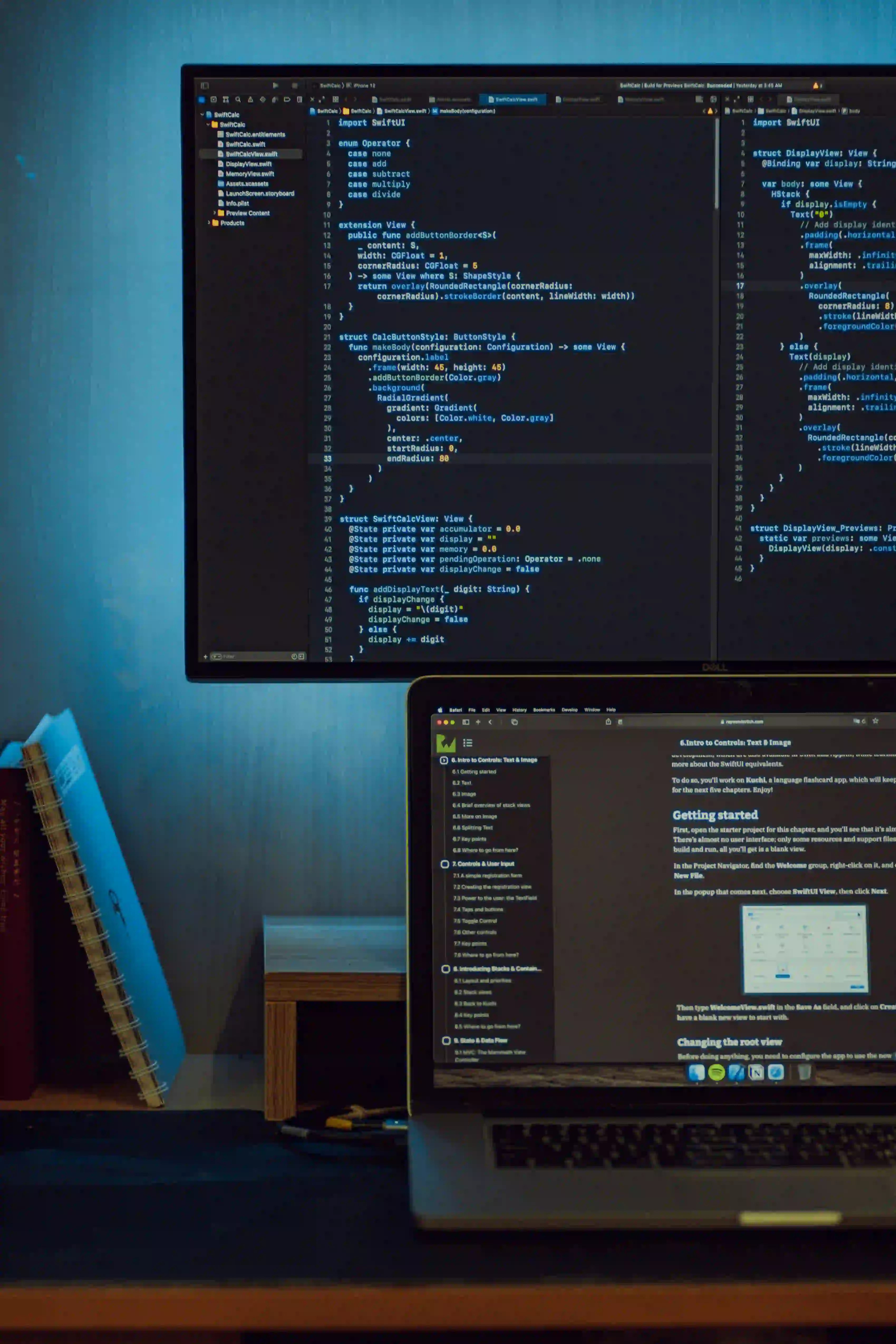
Common Pitfalls to Avoid When Using Java 16 Stream.toList()
When it comes to working with data in Java, the Stream API has become an essential tool for processing collections. However, like any powerful tool, it's essential to use it carefully to avoid potential pitfalls. The Stream.toList()
method in Java 16 is particularly powerful, but it's not without its challenges. In this article, we'll explore common pitfalls to avoid when using Stream.toList()
in Java 16, along with best practices for effective usage.
Pitfall 1: Misusing Stream.toList()
Let's start with the most common pitfall when using Stream.toList()
- misunderstanding its purpose. The Stream.toList()
method is used to convert a stream into a List
. It's essential to remember that streams are intended for processing data in a functional way, and converting them into collections should be done thoughtfully.
Here's an example of how Stream.toList()
can be misused:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> collectedNames = names.stream().toList();
In this example, using toList()
at the end of the stream may seem harmless, but it's unnecessary in this case. The names
list is already a collection, and calling toList()
here adds needless complexity.
To avoid this pitfall, always consider whether converting a stream into a list is truly necessary. In many cases, working directly with the stream may be more efficient and maintainable.
Pitfall 2: Forgetting Terminal Operations
Streams in Java are lazy, meaning they don't perform any processing until a terminal operation is invoked. When using Stream.toList()
, it's crucial to remember to include a terminal operation to trigger the stream processing.
Consider the following example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> collectedNames = names.stream().filter(name -> name.length() > 4).toList();
In this case, the filter()
operation is applied to the stream, but a terminal operation is missing. As a result, the stream does not process any data, and the collectedNames
list remains empty.
To address this pitfall, always ensure that a terminal operation, such as forEach()
, toArray()
, or toList()
, is included to trigger the stream processing and produce the desired result.
Pitfall 3: Unawareness of Stream Characteristics
When working with streams, it's important to understand the characteristics of the source and intermediate operations applied to the stream. The characteristics, such as whether the stream is ordered or sorted, can significantly impact the behavior of terminal operations like toList()
.
For example, consider the following scenario:
List<String> fruits = Arrays.asList("apple", "banana", "cherry");
List<String> collectedFruits = fruits.stream().filter(fruit -> fruit.startsWith("a")).toList();
In this case, the result of collectedFruits
may vary depending on the characteristics of the original stream and the intermediate operations. If the stream is unordered, the resulting list may not match the order of the original data.
To avoid potential issues related to stream characteristics, be mindful of the source of the stream and any intermediate operations applied to it. Understanding how these operations may affect the characteristics of the stream will help you use Stream.toList()
more effectively.
Best Practices for Using Stream.toList()
Now that we've explored common pitfalls, let's discuss best practices for using Stream.toList()
effectively in Java 16.
Only Convert When Necessary
Before applying Stream.toList()
, consider whether you truly need to convert the stream into a list. In many cases, working directly with the stream using operations like forEach()
or reduce()
may be more idiomatic and efficient.
Include Proper Error Handling
When working with streams and terminal operations like toList()
, ensure that you include proper error handling mechanisms, such as handling potential exceptions thrown during stream processing. This will help make your code more robust and maintainable.
Take Stream Characteristics into Account
Always consider the characteristics of the stream, including whether it's ordered, sorted, or distinct, and how these characteristics may affect the outcome of the terminal operation. Understanding these characteristics will allow you to predict the behavior of Stream.toList()
more accurately.
Test for Performance
If you're converting large streams into lists, consider testing the performance impact of Stream.toList()
compared to other stream operations. This will help you identify potential bottlenecks and optimize your code for efficiency.
By following these best practices and being mindful of the potential pitfalls, you can use Stream.toList()
effectively and gain the full benefits of Java 16's Stream API.
Closing the Chapter
The Stream.toList()
method in Java 16 is a powerful tool for converting streams into lists, but it requires careful consideration to avoid common pitfalls. By understanding when to use Stream.toList()
and how to handle stream characteristics, you can leverage this method effectively in your Java projects. Keeping best practices in mind, such as minimizing unnecessary conversions and considering performance implications, will help you write more robust and efficient code when working with streams.
Remember, the key to using Stream.toList()
successfully lies in a thorough understanding of stream processing and thoughtful consideration of when to convert streams into lists. With these principles in mind, you can make the most of Java 16's Stream API and write more efficient, readable, and maintainable code.
To dive deeper into Java Streams and explore advanced topics, consider exploring the official Java Streams documentation, where you can find additional insights and examples to expand your knowledge.