Overcoming Challenges in Parallel Programming
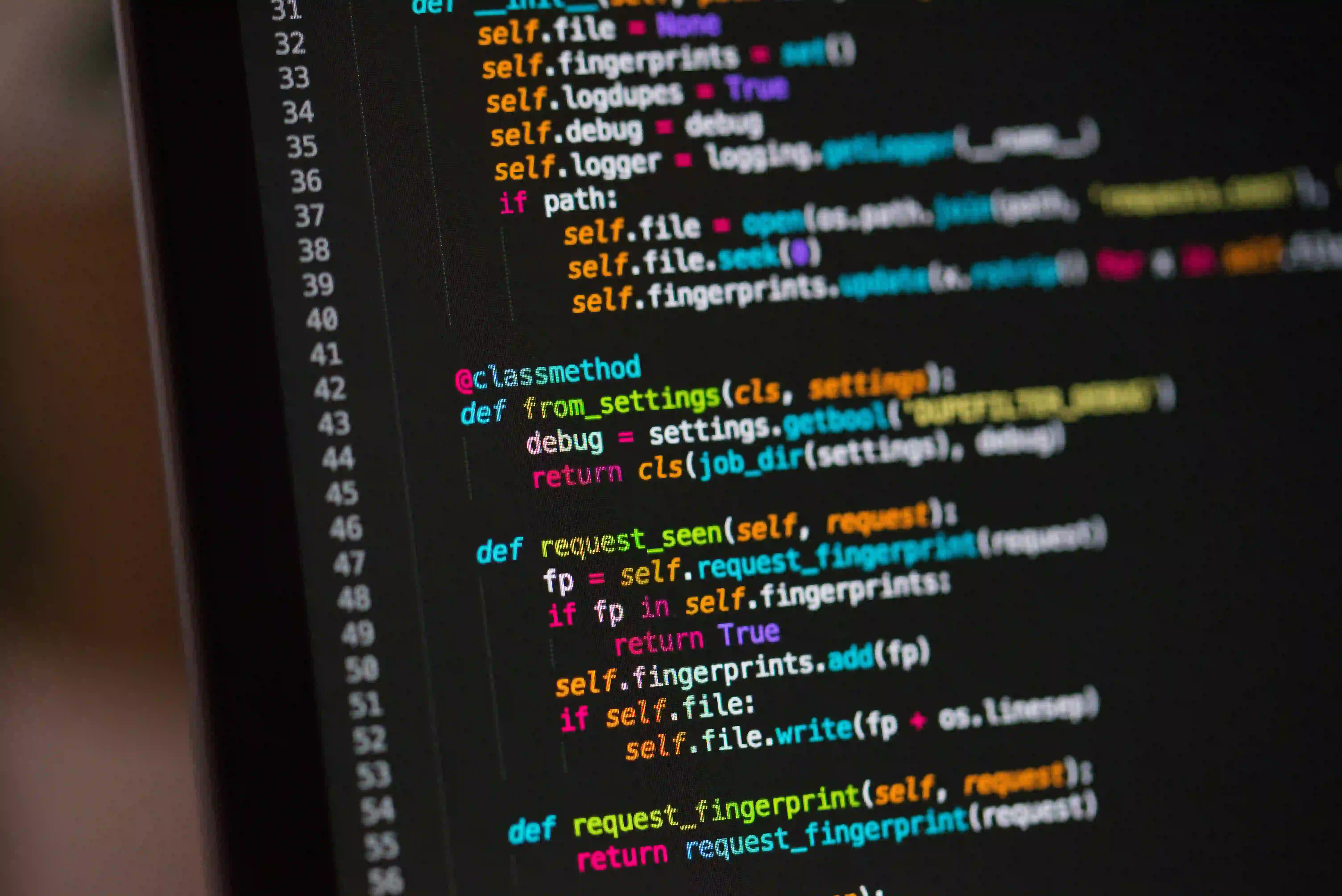
Overcoming Challenges in Parallel Programming
Parallel programming has become increasingly important in the field of software development due to the need for high-performance computing. However, it also brings with it a set of challenges that developers must overcome to ensure optimal performance and reliability. In this blog post, we will discuss some of the common challenges in parallel programming and explore strategies to address them using Java.
Understanding the Challenges
Parallel programming involves the use of multiple processors or cores to execute tasks concurrently, which can significantly improve the performance of an application. However, this concurrency introduces challenges such as race conditions, deadlocks, and thread synchronization issues.
Race Conditions
Race conditions occur when multiple threads access shared resources simultaneously, leading to unpredictable behavior and incorrect results. This can happen, for example, when two threads attempt to write to the same variable without proper synchronization.
Deadlocks
Deadlocks occur when two or more threads are blocked forever, each waiting for the other to release a resource. This can happen when threads acquire multiple locks in different orders, leading to a circular waiting condition.
Thread Synchronization
Ensuring proper synchronization between threads is crucial to avoid race conditions and deadlocks. This requires careful management of shared resources and the use of synchronization constructs such as locks, semaphores, and barriers.
Strategies for Overcoming Challenges
Using Thread-Safe Data Structures
In Java, the java.util.concurrent
package provides a variety of thread-safe data structures such as ConcurrentHashMap
, ConcurrentLinkedQueue
, and CopyOnWriteArrayList
. These data structures are designed to be accessed by multiple threads concurrently without the need for external synchronization.
ConcurrentMap<String, String> concurrentMap = new ConcurrentHashMap<>();
Using thread-safe data structures can reduce the likelihood of race conditions and eliminate the need for manual synchronization, making the code more reliable and easier to maintain.
Explicit Locking
Java provides the Lock
interface and its implementations such as ReentrantLock
for explicit locking. Unlike implicit locking with synchronized methods or blocks, explicit locking gives the developer more control over the locking and unlocking of resources.
Lock lock = new ReentrantLock();
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
Using explicit locking can help prevent race conditions and also enables more advanced locking mechanisms such as condition variables for inter-thread communication.
Thread Synchronization with synchronized
Keyword
The synchronized
keyword in Java provides a built-in mechanism for thread synchronization. It can be used to create synchronized methods or blocks to control access to critical sections of code.
public synchronized void synchronizedMethod() {
// Critical section
}
While the synchronized
keyword simplifies the process of synchronization, it may lead to performance overhead due to its intrinsic locking mechanism.
Asynchronous Programming with CompletableFuture
Java 8 introduced the CompletableFuture
class, which provides a powerful way to perform asynchronous operations and compose asynchronous computations. By leveraging CompletableFuture
, developers can write non-blocking, asynchronous code that can improve the responsiveness and scalability of an application.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello")
.thenApplyAsync(s -> s + " World");
Using CompletableFuture
can help manage concurrent tasks more effectively and handle dependencies between asynchronous operations.
Key Takeaways
Parallel programming in Java presents various challenges related to race conditions, deadlocks, and thread synchronization. By leveraging techniques such as the use of thread-safe data structures, explicit locking, and asynchronous programming with CompletableFuture
, developers can overcome these challenges and build high-performance, reliable parallel applications.
In conclusion, understanding the intricacies of parallel programming and employing the right strategies can lead to efficient utilization of resources and improved application performance.
To delve deeper into the world of parallel programming in Java, check out Java Concurrency in Practice for comprehensive insights and best practices.
Happy parallel programming!