Navigating the Accountability Maze: Balancing Autonomy and Responsibility
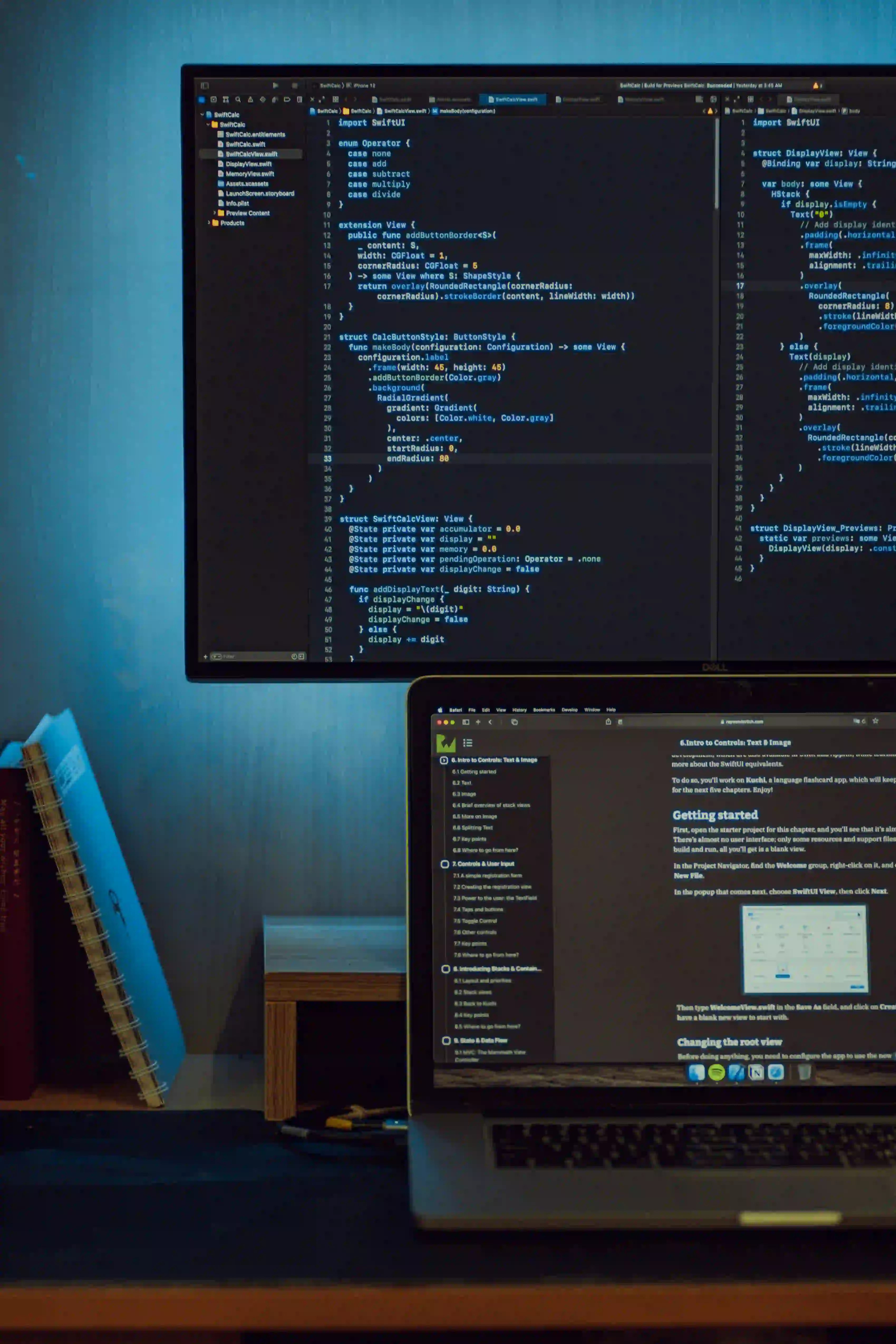
Navigating the Accountability Maze: Balancing Autonomy and Responsibility in Java
Accountability is a crucial aspect of software development. In Java, striking the right balance between autonomy and responsibility is essential for creating robust and maintainable code. In this article, we will explore the concept of accountability in Java programming, the challenges it presents, and strategies for navigating this complex landscape.
Understanding Accountability in Java
In the context of Java programming, accountability refers to the practice of ensuring that each component of a system takes responsibility for its actions and behaves in a predictable and reliable manner. This involves adhering to best practices, writing clean and understandable code, and handling errors gracefully.
One of the fundamental principles of Java development is the concept of object-oriented programming (OOP), which emphasizes the organization of code into reusable and modular components. Each object is accountable for its state and behavior, encapsulating data and methods within a defined scope.
Challenges in Navigating Accountability
In large-scale Java projects, maintaining accountability can be challenging due to the complexity of interactions between various components. The following challenges often arise:
-
Distributed Responsibility: As Java applications scale, it becomes increasingly challenging to ensure that each component maintains its accountability without becoming overly dependent on other parts of the system.
-
Concurrency: Dealing with concurrent behavior in Java applications introduces a new layer of complexity in ensuring accountability, especially when multiple threads are accessing shared resources.
-
Error Handling: Properly handling exceptions and errors while maintaining accountability across different layers of the application is crucial for robustness.
Strategies for Navigating the Accountability Maze
1. Encapsulation and Modularity
One of the core principles of OOP, encapsulation, plays a key role in maintaining accountability within Java programs. By encapsulating data within classes and providing well-defined interfaces for interaction, you can limit external access and enforce accountability at the object level.
public class EncapsulationExample {
private int data;
public int getData() {
return data;
}
public void setData(int newData) {
// Add validation logic to maintain accountability
if (newData > 0) {
this.data = newData;
} else {
throw new IllegalArgumentException("Data must be positive");
}
}
}
2. Design Patterns for Accountability
Utilizing design patterns such as the Singleton pattern or the Facade pattern can help in maintaining accountability by providing well-defined entry points and controlling the creation and access of objects.
3. Exception Handling
Proper exception handling is crucial for maintaining accountability in Java programs. By propagating exceptions to the appropriate level of the application and providing meaningful error messages, you can ensure that each component takes responsibility for its exceptional conditions.
try {
// Code that may throw an exception
} catch (CustomException e) {
// Log the error and propagate the exception
throw new CustomException("An error occurred", e);
}
4. Testing and Quality Assurance
Comprehensive unit testing and QA processes are essential for validating the accountability of Java code. Test-driven development (TDD) can help in ensuring that each component behaves as expected and maintains its responsibility in different scenarios.
5. Continuous Integration and Delivery (CI/CD)
Implementing CI/CD pipelines with automated build and deployment processes can help in enforcing accountability by quickly identifying integration issues and ensuring that the codebase remains consistent and reliable.
My Closing Thoughts on the Matter
Navigating the accountability maze in Java programming requires a combination of best practices, design patterns, and a thorough understanding of object-oriented principles. By embracing encapsulation, leveraging design patterns, handling exceptions diligently, and prioritizing testing and automation, developers can strike a balance between autonomy and responsibility, ultimately leading to more maintainable and accountable Java code.
In the ever-evolving landscape of software development, maintaining accountability remains a constant challenge, but with the right strategies and mindset, Java developers can navigate this maze with confidence and create robust, reliable, and accountable software systems.
By embracing encapsulation, leveraging design patterns, handling exceptions diligently, and prioritizing testing and automation, developers can strike a balance between autonomy and responsibility, ultimately leading to more maintainable and accountable Java code.
Remember, the Java language provides the tools and principles necessary to navigate the accountability maze effectively. Embracing these principles will not only result in robust and maintainable code but also foster a culture of accountability and professionalism within development teams.
So, the next time you write Java code, remember to tread the accountability maze with purpose, and let the principles of autonomy and responsibility guide your way. Happy coding!