Max Connections Exhausted in Tomcat JDBC Connection Pool
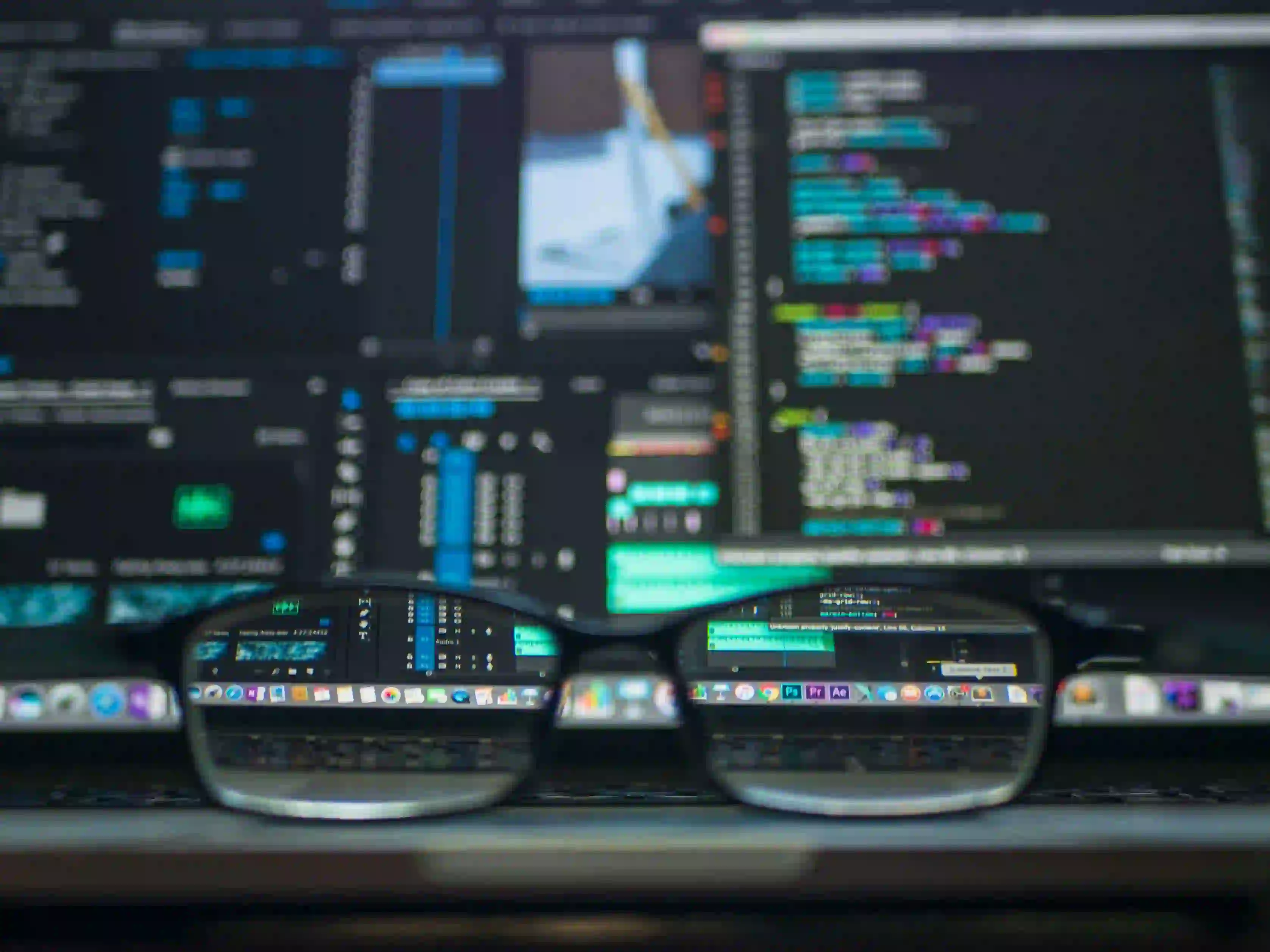
Solving Max Connections Exhausted issue in Tomcat JDBC Connection Pool
In a high-traffic Java web application, database connection management is crucial for maintaining optimal performance. However, even with a robust connection pool like Tomcat JDBC, you may encounter the dreaded "Max Connections Exhausted" error. This occurs when the application consumes all available database connections, leading to a bottleneck in the system.
In this blog post, we'll explore why this issue arises, its impact on the application, and most importantly, how to address and prevent it from occurring.
Understanding the issue
Before delving into the resolution, let's understand why "Max Connections Exhausted" occurs in the Tomcat JDBC connection pool.
Reasons for the error
The primary reasons for this issue can be attributed to a combination of factors:
-
Inefficient connection management: Inadequate or incorrect management of database connections within the application code can result in overutilization of the connection pool.
-
Surges in traffic: Sudden spikes in user traffic can lead to a surge in connection requests, overwhelming the connection pool and exhausting available connections.
-
Long-running queries: Lengthy database operations or poorly optimized queries can tie up connections, reducing the pool's availability.
Impact on the application
When the "Max Connections Exhausted" error occurs, it can have detrimental effects on the application:
- Increased response times: As the connection pool becomes saturated, requests for new connections experience delays, leading to slower response times.
- Resource contention: With all connections exhausted, new requests must wait for existing connections to be released, causing resource contention and potential service degradation.
- Potential outages: In extreme cases, the application may experience outages or become unresponsive due to the lack of available database connections.
Now that we understand the implications of this issue, let's move on to resolving it.
Resolving the issue
Analyzing connection usage
Before diving into code changes, it's essential to analyze the application's connection usage to identify the root cause. Tools like JavaMelody or database monitoring tools can provide insights into connection acquisition and release patterns, helping pinpoint areas of improvement.
Optimizing connection handling
Optimizing the management of database connections within the application can significantly mitigate the "Max Connections Exhausted" problem. Here are some best practices to consider:
1. Connection reuse
// Example: Reusing the connection within a try-with-resources block
try (Connection connection = dataSource.getConnection()) {
// Perform database operations using the connection
}
By properly reusing connections and promptly releasing them after use, you can prevent unnecessary accumulation of connections in the pool.
2. Connection pooling parameters
Reviewing and fine-tuning the connection pool parameters in the context.xml
file can also alleviate connection exhaustion.
<Resource name="jdbc/myDB"
global="jdbc/myDB"
auth="Container"
type="javax.sql.DataSource"
driverClassName="com.mysql.cj.jdbc.Driver"
url="jdbc:mysql://localhost:3306/mydb"
username="dbuser"
password="dbpass"
maxTotal="100"
maxIdle="30"
maxWaitMillis="10000" />
Adjusting maxTotal
, maxIdle
, and maxWaitMillis
based on the application's needs and database capacity can optimize connection utilization.
3. Connection error handling
Proper error handling for database connections is crucial. Always ensure connections are closed in a finally
block or by leveraging try-with-resources to prevent leaks and free up connections promptly.
// Example: Closing the connection in a finally block
Connection connection = null;
try {
connection = dataSource.getConnection();
// Perform database operations
} catch (SQLException e) {
// Handle the exception
} finally {
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
// Handle the exception
}
}
}
Addressing potential connection leaks is pivotal in maintaining a healthy and available connection pool.
Scaling database resources
In scenarios where optimizations within the application yield limited results, it may be necessary to scale database resources by increasing connection limits, fine-tuning database configurations, or implementing database caching solutions.
Closing the Chapter
The "Max Connections Exhausted" issue in the Tomcat JDBC connection pool can significantly impact the performance and reliability of a Java web application. By understanding the reasons behind this problem and employing best practices such as optimizing connection handling and scaling database resources, you can mitigate the occurrence of this error and ensure the efficient utilization of database connections.
Remember, proactive monitoring and periodic evaluations of connection usage are essential for maintaining a healthy and responsive application. With a well-managed connection pool and a thorough understanding of connection utilization patterns, you can preemptively address potential bottlenecks and keep your Java web application running smoothly.
Addressing this critical aspect of application performance not only ensures a seamless user experience but also contributes to the overall stability and scalability of your Java web application.
Understanding and effectively managing database connections are pivotal in maintaining a high-performing and responsive Java web application. By implementing best practices for connection handling, adjusting connection pool parameters, and scaling database resources when necessary, you can prevent the detrimental effects of connection exhaustion and ensure the efficient utilization of database connections.