Troubleshooting Micrometer Integration with Prometheus
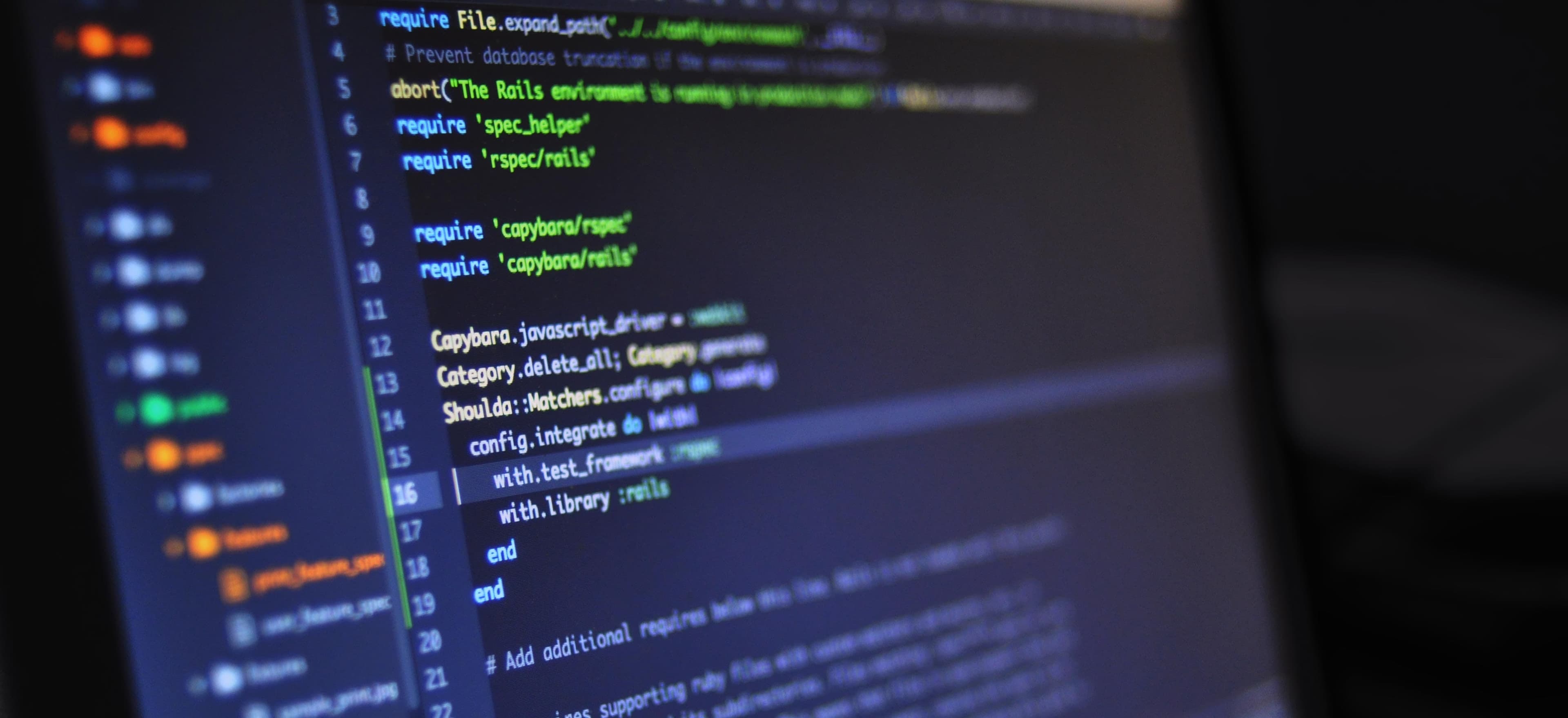
- Published on
Troubleshooting Micrometer Integration with Prometheus
Micrometer is a powerful Java library that provides a simple facade over the instrumentation clients for the most popular monitoring systems, including Prometheus. Integrating Micrometer with Prometheus allows developers to effortlessly collect and export application metrics, which are crucial for gaining insights into an application's performance and behavior. However, like any integration, issues can arise, and troubleshooting becomes essential. In this article, we will explore common problems when integrating Micrometer with Prometheus and how to troubleshoot them effectively.
Issue 1: No Data in Prometheus
One common issue is when the Prometheus server does not receive any data from the Micrometer integration. This could be due to a misconfiguration or an issue with the data collection process.
Troubleshooting Steps:
-
Verify Prometheus Configuration: Ensure that the Prometheus configuration includes the correct endpoint for scraping metrics from the application. The
scrape_configs
section of theprometheus.yml
file should include the appropriate target with the/actuator/prometheus
endpoint configured. -
Check Micrometer Setup: Confirm that Micrometer is properly configured in the application. The Micrometer registry should be bound to the Prometheus registry, and the application should be producing and exposing metrics.
-
Verify Connectivity: Check the connectivity between the application and the Prometheus server. Verify that the application is accessible from the server and that there are no network issues preventing metric scraping.
Example Code:
// Micrometer setup with Prometheus registry
MeterRegistry registry = new PrometheusMeterRegistry(PrometheusConfig.DEFAULT);
registry.config().commonTags("application", "my-application");
In this example, we are creating a Micrometer registry with the Prometheus implementation and setting common tags for the metrics. This ensures that the metrics are exposed correctly for scraping by Prometheus.
Issue 2: Incorrect Metric Values
Another issue that may arise is when the metrics collected by Micrometer do not reflect the expected values. This could be due to misconfigured meters or incorrect usage of Micrometer's APIs.
Troubleshooting Steps:
-
Review Meter Registration: Verify that the meters are correctly registered and associated with the appropriate metric names and tags. Incorrect registration can lead to metrics not being captured or being mislabeled.
-
Check Metric Collection: Inspect the code responsible for metric collection. Ensure that the metrics are being captured at the right places in the application code and that the values being recorded are accurate representations of the application's behavior.
-
Debug Meter Usage: Use logging and debugging techniques to track the flow of metric recording in the application. This can help identify any discrepancies between the expected and actual values.
Example Code:
// Registering a custom gauge metric
Gauge.builder("custom.gauge", () -> customService.getData())
.description("Description of custom gauge")
.tags("custom_tag", "value")
.register(registry);
In this example, we are registering a custom gauge metric using Micrometer. The gauge function retrieves data from a custom service and exposes it as a metric. Verifying the correctness of such registrations is crucial in ensuring the accuracy of the collected metrics.
Issue 3: High Cardinality Metrics
High cardinality refers to a large number of unique label sets for a given metric, which can lead to increased memory usage and query load on the Prometheus server. Managing high cardinality metrics is a common challenge when using Micrometer with Prometheus.
Troubleshooting Steps:
-
Review Label Usage: Evaluate the usage of labels in metric registrations. Determine if there are excessive unique label combinations being used, leading to high cardinality. Consider consolidating or reducing the number of labels where possible.
-
Utilize Filters: Micrometer allows the use of filters to restrict the number of unique combinations reported to the monitoring system. Implementing filters can help control high cardinality by excluding less critical dimensions from the reported metrics.
-
Optimize Queries: When querying metrics in Prometheus, optimize the selection of labels and dimensions to avoid retrieving excessively detailed data. Refining the queries can help mitigate the impact of high cardinality metrics on the Prometheus server.
Example Code:
// Using a common tag for reducing cardinality
registry.config().commonTags("application", "my-application");
In this example, setting a common tag for the registry can help reduce the cardinality of the reported metrics. By including common tags, the number of unique label sets can be controlled, thus mitigating the impact of high cardinality.
Bringing It All Together
Integrating Micrometer with Prometheus provides valuable insights into an application's performance, but encountering issues during the integration is not uncommon. By following the troubleshooting steps outlined in this article and reviewing the example code provided, developers can effectively identify and resolve issues related to data collection, metric accuracy, and high cardinality. A robust monitoring and metrics system is essential for understanding the behavior of Java applications, and resolving integration issues ensures the reliability of the collected data for informed decision-making and performance optimization.
Make sure to check out the official Micrometer documentation for comprehensive guidance on integrating Micrometer with various monitoring systems, including Prometheus.
By addressing these common issues and understanding the principles behind Micrometer and Prometheus integration, developers can ensure a seamless and effective monitoring solution for their Java applications.