Understanding Hazelcast: Handling Data Distribution
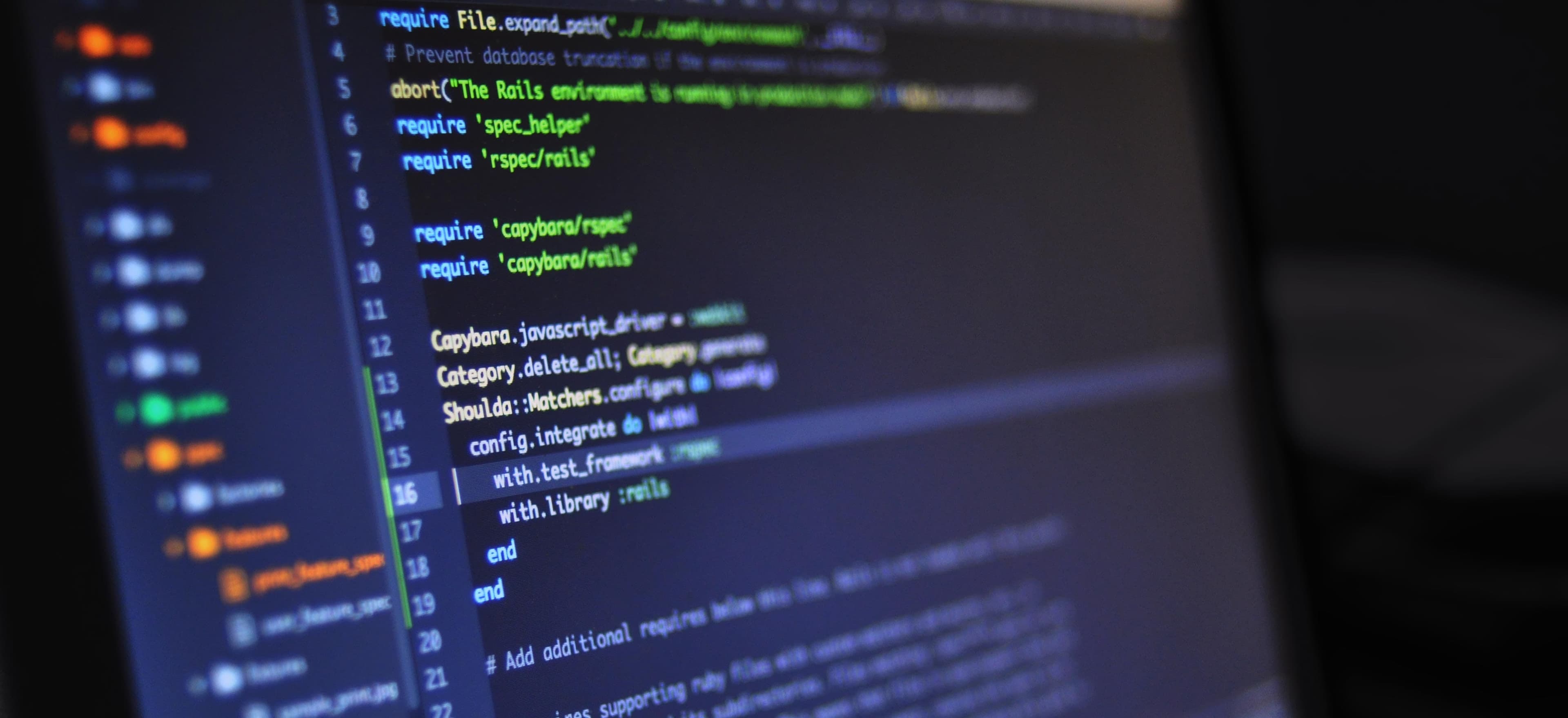
- Published on
Understanding Hazelcast: Handling Data Distribution
When it comes to building distributed systems in Java, one of the key challenges is effectively managing data distribution across multiple nodes. This is where Hazelcast, an open-source in-memory data grid, comes into play. In this article, we'll dive into how Hazelcast handles data distribution and explore its key features and best practices for managing distributed data effectively.
What is Hazelcast?
Hazelcast is a distributed computing platform that provides a set of in-memory data management tools. It allows you to distribute data across a cluster of nodes, providing high availability, fault tolerance, and scalability. With its simple and easy-to-use APIs, Hazelcast is an ideal choice for building distributed systems in Java.
Data Distribution in Hazelcast
At the heart of Hazelcast is its ability to distribute data across multiple nodes in a cluster. This enables parallel processing, fault tolerance, and efficient utilization of resources. Let's explore how Hazelcast achieves this:
Partitioning and Data Distribution
Hazelcast partitions the data across the cluster using a technique known as partitioning. It divides the data into partitions, and each partition is then assigned to a specific node in the cluster. This allows for parallel processing and efficient data access.
Data Replication
To ensure fault tolerance and high availability, Hazelcast provides support for data replication. It replicates data across multiple nodes, so that in case of a node failure, the data can still be accessed from other nodes in the cluster. This eliminates single points of failure and ensures data reliability.
Consistency and Eventual Consistency
Hazelcast offers strong consistency guarantees for data access and updates. However, in distributed systems, achieving strong consistency across all nodes can impact performance. To address this, Hazelcast also supports eventual consistency, allowing for faster data access at the cost of immediate consistency.
Key Features for Managing Distributed Data
Hazelcast offers a range of features and tools for managing distributed data effectively. Let's take a look at some of the key features:
Distributed Maps
Hazelcast provides a distributed implementation of the java.util.Map
interface, allowing you to store key-value pairs across the cluster. This provides a simple and intuitive way to distribute and access data.
Distributed Executors
With distributed executors, you can execute tasks and processes across the cluster in a parallel and distributed manner. This allows for efficient utilization of resources and faster processing of tasks.
Near Cache
Hazelcast's Near Cache feature allows for caching commonly accessed data near the client, reducing the need to access data from the cluster. This improves overall performance and reduces network overhead.
Entry Processors
Entry processors in Hazelcast allow you to perform operations on entries within a map in a distributed manner. This enables efficient data processing and manipulation across the cluster.
Eventual Consistency
As mentioned earlier, Hazelcast supports eventual consistency, allowing for faster data access at the cost of immediate consistency. This provides a balance between performance and data reliability.
Best Practices for Handling Data Distribution in Hazelcast
To effectively manage data distribution in Hazelcast, it's important to follow best practices and guidelines. Here are some best practices to consider:
Use Partition Awareness
When deploying Hazelcast in a production environment, it's important to be partition-aware. This involves understanding the partitioning strategy used by Hazelcast and designing your data distribution and access patterns accordingly.
Data Partitioning Strategies
Hazelcast provides different partitioning strategies, such as PARTITION
, CUSTOM
, and AUTO
. It's important to evaluate your data access patterns and choose the appropriate partitioning strategy to optimize data distribution and access.
Optimizing Data Replication
While data replication is crucial for fault tolerance, it's also important to optimize data replication to avoid unnecessary overhead. Evaluate the replication factor and configure it based on your fault tolerance requirements.
Monitoring and Maintenance
Regular monitoring and maintenance of the Hazelcast cluster are essential for ensuring optimal data distribution and performance. Utilize Hazelcast Management Center or other monitoring tools to keep an eye on data distribution and node health.
Code Example: Using Hazelcast Distributed Map
Let's take a look at a simple code example that demonstrates the use of a distributed map in Hazelcast:
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
import java.util.Map;
public class HazelcastMapExample {
public static void main(String[] args) {
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
Map<String, String> distributedMap = hazelcastInstance.getMap("my-distributed-map");
distributedMap.put("key1", "value1");
String value = distributedMap.get("key1");
System.out.println("Retrieved value: " + value);
}
}
In this example, we create a Hazelcast instance and obtain a distributed map named "my-distributed-map". We then put a key-value pair into the map and retrieve the value associated with "key1". This demonstrates how easy it is to work with distributed data using Hazelcast.
Final Thoughts
In conclusion, effectively handling data distribution is essential for building robust and scalable distributed systems. Hazelcast provides powerful tools and features for managing distributed data, including partitioning, replication, and eventual consistency. By following best practices and utilizing Hazelcast's capabilities, you can ensure efficient data distribution and utilization in your Java-based distributed systems.
Incorporate Hazelcast into your distributed Java applications and experience the benefits of efficient data distribution!
To learn more about Hazelcast and its features, check out the official documentation.