Overcoming the Downsides of Oversized Code Components
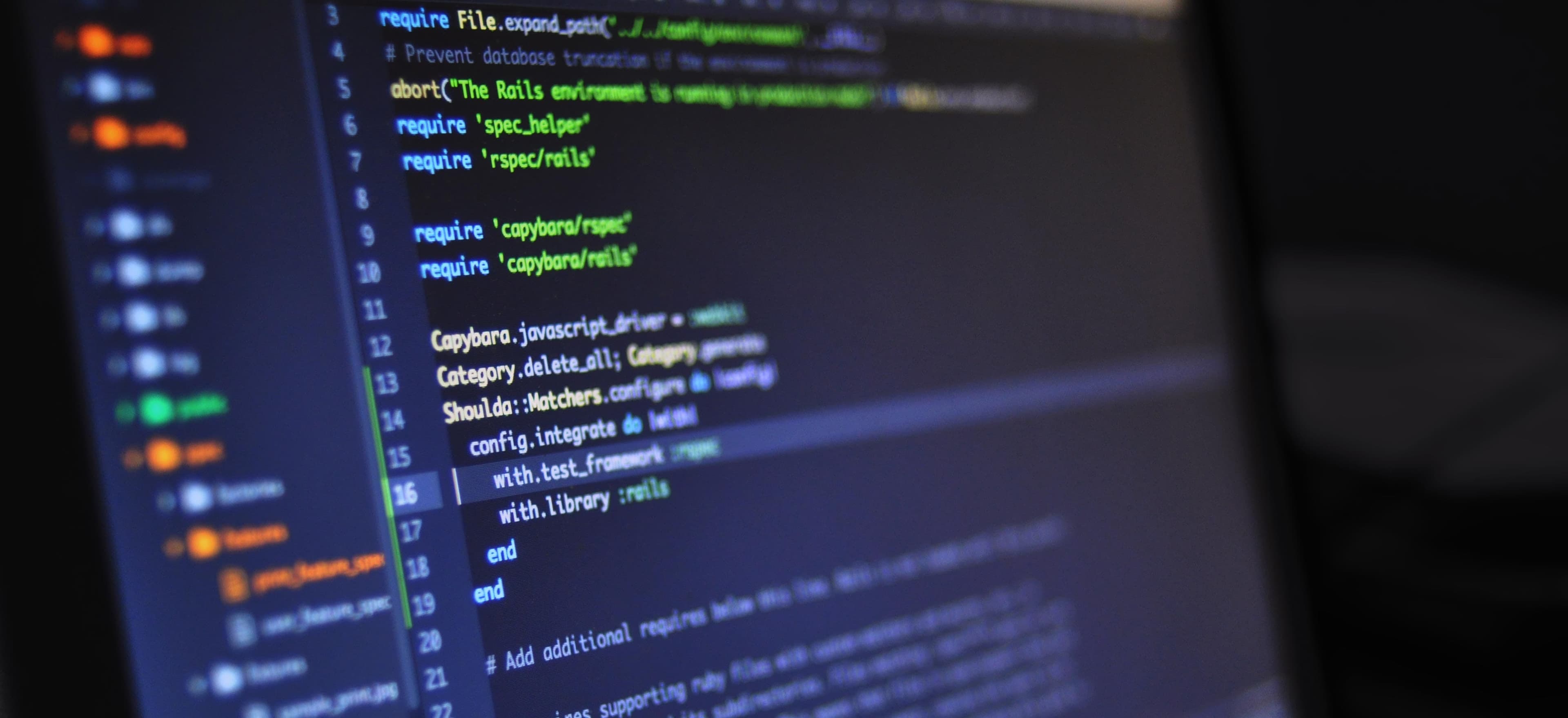
- Published on
Overcoming the Downsides of Oversized Code Components
In the world of Java development, the size of code components can significantly impact the overall quality and maintainability of a software project. While it may be tempting to cram a multitude of functionalities into a single codebase, this approach often leads to bloated, convoluted, and hard-to-maintain code. In this blog post, we will explore the downside of oversized code components and discuss strategies for overcoming these challenges.
The Downside of Oversized Code Components
1. Complexity
Large code components tend to be more complex, making it difficult for developers to understand, modify, and extend the functionality. This complexity can lead to an increase in bugs and make it challenging to onboard new team members.
2. Maintenance
Maintaining oversized code components becomes exponentially harder as the size of the codebase increases. Small changes can have unintended consequences, and the risk of introducing bugs skyrockets.
3. Testing
Testing becomes more cumbersome when dealing with oversized code components. Unit testing, integration testing, and regression testing all become more time-consuming and resource-intensive.
4. Scalability
Large code components often lack scalability. As the software grows, adding new features or refactoring existing ones becomes a daunting task, leading to stagnation and technical debt.
Strategies to Overcome the Downsides
Now that we've examined the challenges posed by oversized code components, let's delve into strategies for mitigating these issues.
1. Single Responsibility Principle (SRP)
Adhering to the SRP, as defined by Robert C. Martin, can be a powerful tool in combating oversized code components. By ensuring that each class or module has only one reason to change, we can keep the codebase lean and focused.
2. Modularization
Breaking down large components into smaller, self-contained modules can significantly reduce complexity and improve maintainability. This allows for better organization and makes it easier to reason about the code.
// Before Modularization
public class MonolithicComponent {
// ... Many methods and fields
}
// After Modularization
public class SmallComponent1 {
// ... Specific functionality
}
public class SmallComponent2 {
// ... Specific functionality
}
3. Dependency Injection
Applying dependency injection can help decouple components and reduce their size. By injecting dependencies rather than hard-coding them within the component, we can promote reusability and testability.
4. Encapsulation
Encapsulation ensures that each component exposes only the necessary functionality, hiding its internal complexities. This not only reduces the cognitive load on developers but also prevents unintended misuse of the component.
5. Code Reviews and Refactoring
Regular code reviews and refactoring sessions can help identify and rectify oversized code components. By continuously reviewing the codebase and refactoring where necessary, we can prevent the accumulation of technical debt.
6. Design Patterns
Employing design patterns such as the Strategy Pattern, Factory Pattern, and Observer Pattern can promote code reuse and maintainability, effectively preventing code components from growing out of control.
Key Takeaways
In the realm of Java development, the pitfalls of oversized code components are omnipresent. However, by adhering to fundamental principles such as the Single Responsibility Principle, embracing modularization, and leveraging design patterns, we can effectively overcome these challenges and foster a codebase that is maintainable, scalable, and robust.
Remember, the goal is not to eliminate complexity but to manage it effectively. By following these strategies and continuously reevaluating the codebase, we can ensure that our Java projects remain agile and adaptable in the face of evolving requirements.
So, let's strive for code components that are concise, focused, and resilient, ultimately leading to software that is a joy to develop, test, and maintain.
Happy coding!
Incorporate these links for additional reading: