Optimizing SaaS Pricing Strategies for Business Transactions
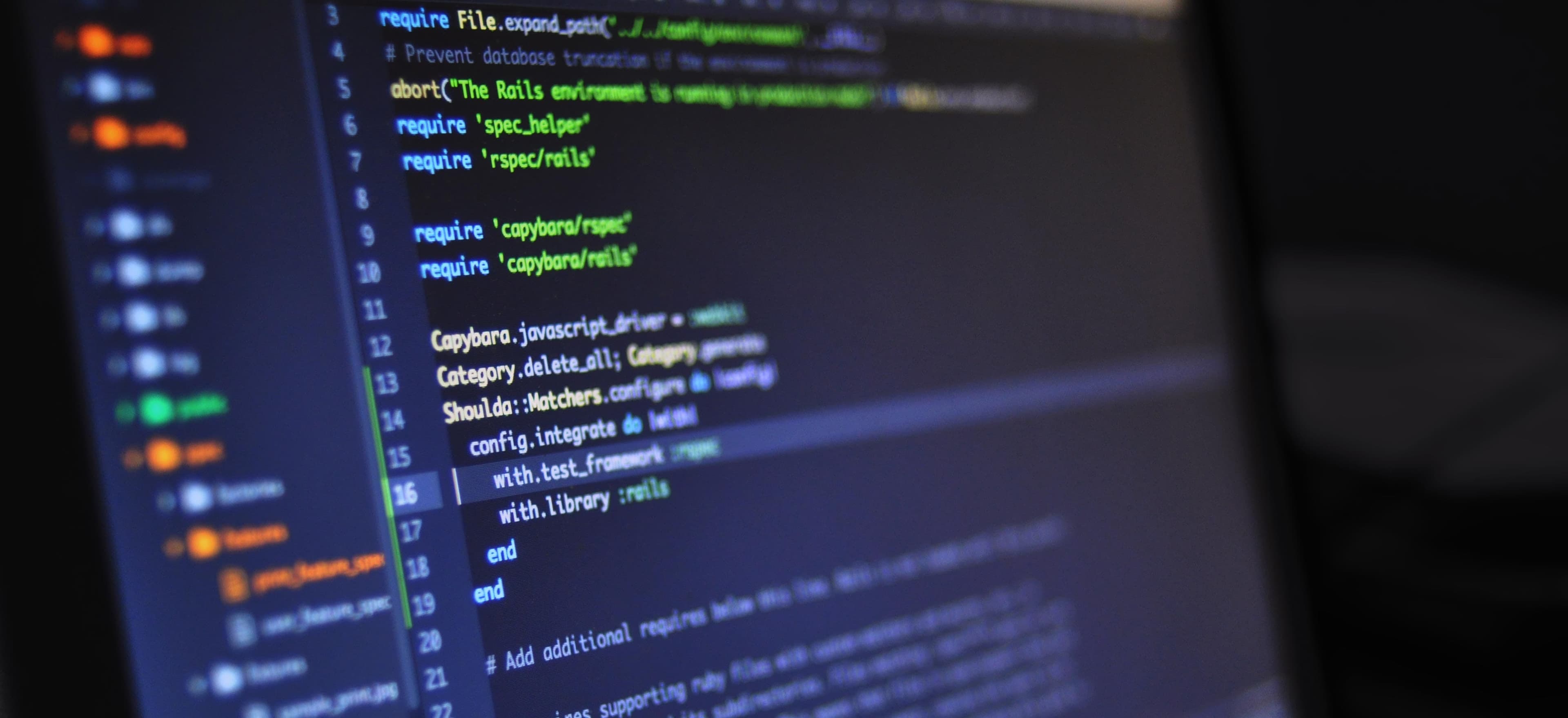
- Published on
Strategies for Optimizing SaaS Pricing in Java
As the Software as a Service (SaaS) industry continues to grow rapidly, optimizing pricing strategies has become essential for SaaS businesses. With Java being a popular language for building SaaS products, it's crucial to understand how Java can be utilized to optimize pricing strategies. In this article, we'll explore various strategies for optimizing SaaS pricing using Java and how these strategies can impact business transactions.
Understanding Dynamic Pricing
Dynamic pricing is a strategy where businesses adjust the price of their products or services in real-time based on market demand, competitor pricing, and other external factors. In the context of SaaS, dynamic pricing can be particularly effective as it allows businesses to capture the value they deliver to each customer.
Java's flexibility and robustness make it an ideal choice for implementing dynamic pricing algorithms. Let's consider an example of how dynamic pricing logic can be implemented in Java:
public class DynamicPricing {
public double calculateDynamicPrice(double basePrice, int demandLevel, double competitorPrice) {
double dynamicPrice = basePrice;
if (demandLevel > 8) {
dynamicPrice *= 1.2; // Increase price by 20% for high demand
} else {
dynamicPrice *= 0.8; // Decrease price by 20% for low demand
}
if (competitorPrice < dynamicPrice) {
dynamicPrice = competitorPrice * 0.95; // Set price 5% lower than the competitor
}
return dynamicPrice;
}
}
In this example, the calculateDynamicPrice
method takes the base price, demand level, and competitor price as inputs, and applies dynamic pricing logic to determine the optimal price. This is just a simplified illustration of dynamic pricing, and the actual implementation would be more intricate.
Leveraging Data Analysis with Java
Data analysis plays a crucial role in optimizing SaaS pricing strategies. By analyzing customer usage patterns, market trends, and other relevant data, businesses can make informed decisions about pricing adjustments.
Java's rich ecosystem of libraries and frameworks, such as Apache Hadoop and Apache Spark, makes it well-suited for data analysis tasks. Let's consider how Java can be used to process and analyze customer usage data:
public class CustomerUsageAnalyzer {
public void analyzeUsageData(List<CustomerData> customerDataList) {
// Perform data analysis to identify usage patterns
// Make pricing recommendations based on the analysis
}
}
In this example, the analyzeUsageData
method takes a list of CustomerData
objects as input and processes the data to identify usage patterns. This analysis can then inform pricing recommendations, such as offering tailored pricing plans based on usage patterns.
Implementing A/B Testing and Experimentation
A/B testing is a method of comparing two versions of a product or pricing strategy to determine which one performs better. For SaaS businesses, A/B testing can be used to experiment with different pricing models, payment plans, and promotional offers.
Java can be utilized to implement A/B testing frameworks and conduct experimentation. Let's look at a simplified example of how A/B testing can be implemented in Java:
public class ABTestingService {
public void runPriceExperiment(PriceVariant variantA, PriceVariant variantB) {
// Randomly assign users to variant A or variant B
// Track user interactions and measure key metrics such as conversion rate and revenue
// Determine the better performing variant based on the experiment results
}
}
In this example, the runPriceExperiment
method runs an A/B test by assigning users to different price variants and analyzing key metrics to determine the better-performing variant.
Incorporating Behavioral Economics Principles
Behavioral economics principles, such as anchoring, framing, and cognitive biases, can significantly influence consumers' perception of pricing. By incorporating these principles into pricing strategies, SaaS businesses can nudge customers towards making purchasing decisions that are favorable for the business.
Java can be used to implement pricing strategies that leverage behavioral economics principles. Let's consider an example of how anchoring can be implemented in Java:
public class AnchoringPricingStrategy {
public double applyAnchoring(double basePrice, double anchorPrice) {
// Display the anchor price to influence perception
return basePrice; // Return the actual base price for the product
}
}
In this example, the applyAnchoring
method applies the anchoring principle by displaying an anchor price to influence customers' perception of the actual base price.
The Last Word
Optimizing SaaS pricing strategies using Java involves leveraging dynamic pricing algorithms, conducting data analysis, implementing A/B testing, and incorporating behavioral economics principles. By utilizing Java's flexibility and robustness, businesses can implement sophisticated pricing strategies that impact the bottom line and drive business transactions.
In conclusion, Java serves as a powerful tool for optimizing SaaS pricing strategies, and businesses can benefit greatly from leveraging Java's capabilities in this domain.
Implementing these strategies in Java can lead to more effective pricing models and ultimately drive higher revenues and customer satisfaction for SaaS businesses.
By incorporating dynamic pricing algorithms, data analysis, A/B testing, and behavioral economics principles, SaaS businesses can optimize their pricing strategies to drive higher revenues and customer satisfaction.