Accessing Request Parameters in Spring MVC Binding Without Setters
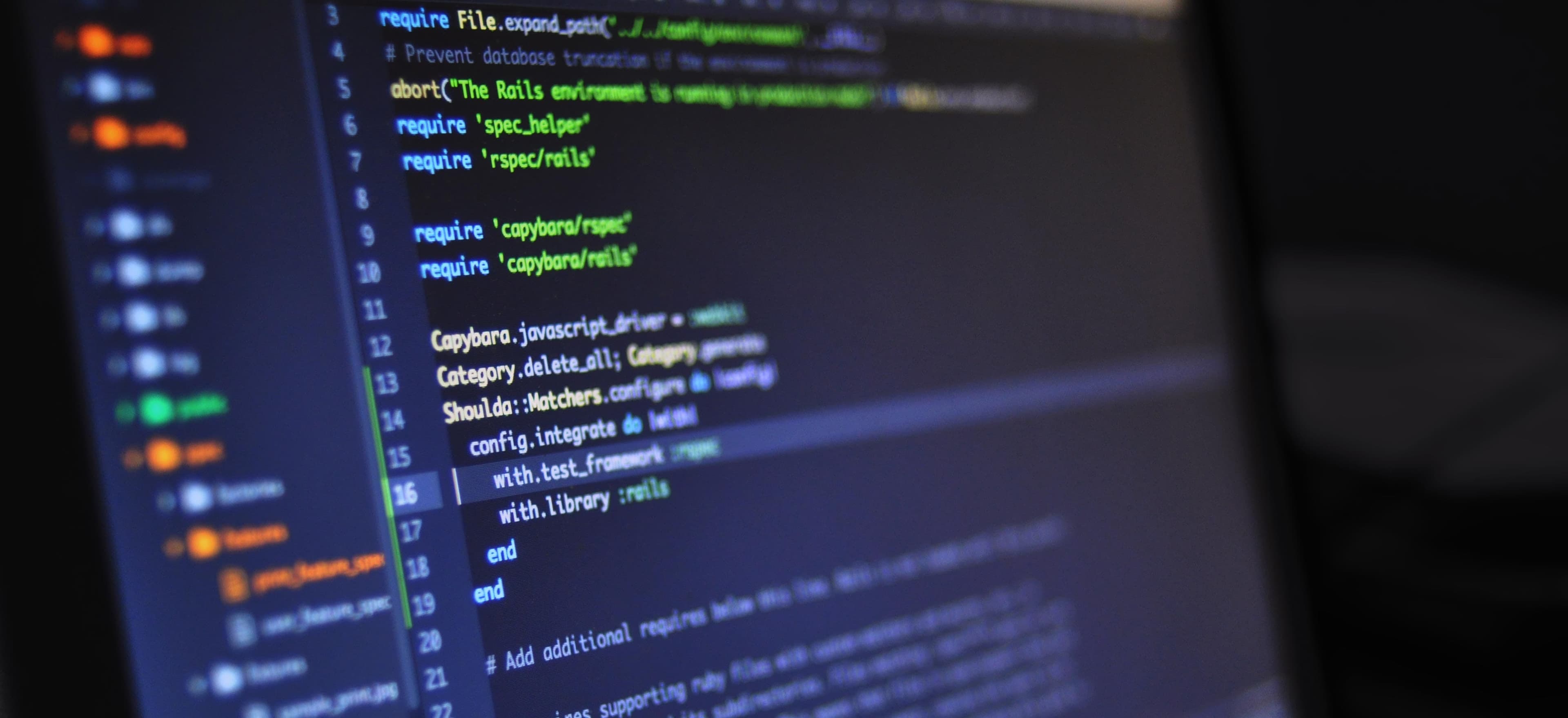
- Published on
Accessing Request Parameters in Spring MVC Binding Without Setters
In a Spring MVC application, it's a common requirement to bind request parameters to a model or controller without explicitly defining setters for each parameter. There are times when you want to have immutable objects or when you want to enforce that a parameter should not be changed after it's initialized.
In this blog post, we will explore how to bind request parameters to a model in Spring MVC without using setters, leveraging the @ModelAttribute
annotation and constructor binding.
Using @ModelAttribute Without Setters
When using the @ModelAttribute
annotation in Spring MVC, by default, Spring tries to set the request parameters using setter methods. However, we can achieve parameter binding without setters by using constructor injection and the @ModelAttribute
annotation.
Let's consider a simple example where we have a User
class with immutable fields.
public class User {
private final String username;
private final int age;
public User(String username, int age) {
this.username = username;
this.age = age;
}
public String getUsername() {
return username;
}
public int getAge() {
return age;
}
}
In the controller, we can use the @ModelAttribute
annotation to bind the request parameters to the User
object without the need for setters.
@Controller
public class UserController {
@PostMapping("/saveUser")
public String saveUser(@ModelAttribute User user) {
// Process the user object
return "success";
}
}
In the above example, Spring MVC will automatically perform request parameter binding to the User
object using the constructor.
Why Use Constructor Binding?
Using constructors for binding request parameters offers several benefits:
- Immutable Objects: It enforces immutability, ensuring that the object state cannot be altered after initialization.
- Clear Intent: It makes the intent of the object clear by explicitly defining the required parameters for creating an instance.
- Thread Safety: Immutable objects are inherently thread-safe, which can be crucial in multi-threaded environments.
Handling Complex Objects
When dealing with more complex objects or objects with nested properties, we can still utilize constructor binding by employing custom converters or formatters. These can handle the conversion of request parameters to complex object types.
Let's consider an example where we have a Address
class as part of the User
object.
public class Address {
private final String street;
private final String city;
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public String getCity() {
return city;
}
}
Now, we can modify the User
class to include the Address
object and set up a constructor that receives the Address
object.
public class User {
private final String username;
private final int age;
private final Address address;
public User(String username, int age, Address address) {
this.username = username;
this.age = age;
this.address = address;
}
// Getters for username, age, and address
}
In the controller, the @ModelAttribute
annotation can still be used for parameter binding without setters, even for the User
class containing the Address
object.
@Controller
public class UserController {
@PostMapping("/saveUser")
public String saveUser(@ModelAttribute User user) {
// Process the user object
return "success";
}
}
By restructuring the User
class and using constructor binding, we maintain immutability while handling complex objects within the request parameters.
To Wrap Things Up
In Spring MVC, we can achieve request parameter binding without setters using constructor injection and the @ModelAttribute
annotation. This approach allows us to work with immutable objects, promotes clear intent, and ensures thread safety. Additionally, when dealing with complex objects or nested properties, we can still apply constructor binding while maintaining immutability and clarity in our code.
By understanding and leveraging these techniques, we can enhance the design and maintainability of our Spring MVC applications while adhering to best practices in object-oriented programming.
For more in-depth insights into Spring MVC and Java development, I recommend checking out the official Spring documentation and other reputable resources in the Java ecosystem.
I hope this blog post has provided valuable insights into handling request parameters in Spring MVC without setters. Thank you for reading!