Optimizing Queue Processing for Efficient Workflows
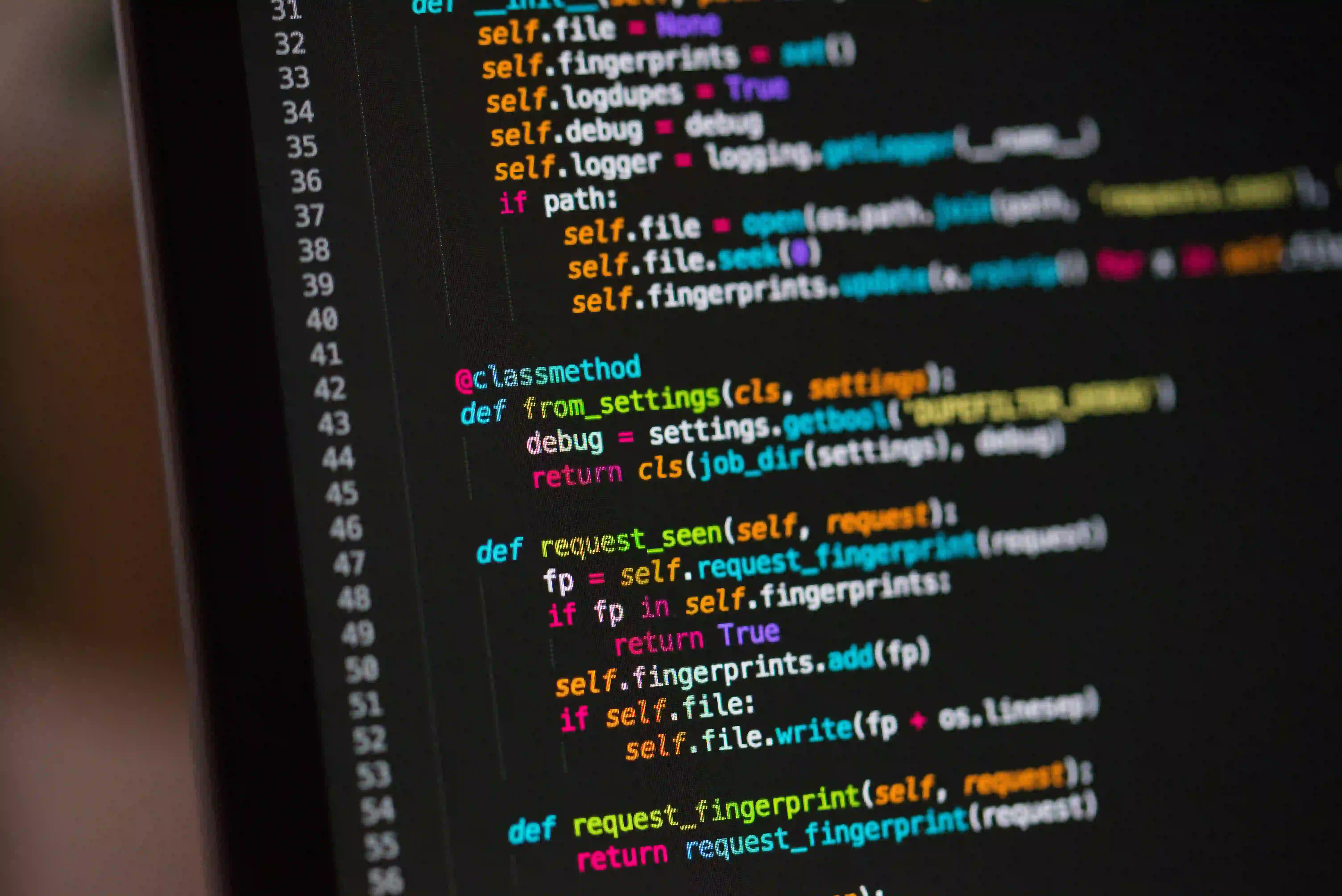
Understanding the Need for Optimized Queue Processing in Java
In the world of software development, complex workflows and tasks often need to be processed efficiently. When dealing with large volumes of data or numerous concurrent requests, it becomes crucial to optimize the processing to ensure smooth and efficient execution. This is where queue processing comes into play.
Queue processing involves managing a queue of tasks or messages, where each task is executed in a sequential or parallel manner. Java, being a popular programming language for building enterprise-level applications, provides robust support for implementing queue processing.
In this blog post, we will explore the importance of optimized queue processing in Java and delve into techniques to achieve efficient workflows using queues.
Why Queue Processing is Crucial for Efficient Workflows
Before we dive into the technical aspects, let's understand why queue processing holds significant value in the realm of software development.
-
Asynchronous Processing: By using queues, tasks can be processed asynchronously, allowing the system to handle multiple tasks concurrently without blocking the main workflow.
-
Load Balancing: Queue processing facilitates load balancing by distributing tasks evenly across different processing units, thereby optimizing resource utilization.
-
Fault Tolerance: In scenarios where a task fails, queue processing allows for easy retry mechanisms and error handling, ensuring fault tolerance in the system.
-
Scalability: Queues enable horizontal scalability by adding more processing units to handle an increased workload, making it easier to scale applications.
Given these advantages, it's evident that optimized queue processing is crucial for achieving efficient and robust workflows in Java applications.
Leveraging Java's Queue Interface for Efficient Processing
Java provides a comprehensive Queue interface as part of the Collections framework, offering a range of implementations such as LinkedList, PriorityQueue, and ArrayDeque. These implementations serve as powerful tools for managing queues and processing tasks efficiently.
Let's take a look at a simple example of utilizing the Queue interface for task processing:
import java.util.LinkedList;
import java.util.Queue;
public class TaskProcessor {
public static void main(String[] args) {
Queue<String> taskQueue = new LinkedList<>();
// Enqueue tasks
taskQueue.add("Task1");
taskQueue.add("Task2");
taskQueue.add("Task3");
// Process tasks
while (!taskQueue.isEmpty()) {
String task = taskQueue.poll();
System.out.println("Processing task: " + task);
// Perform task processing logic
}
}
}
In this example, we create a simple queue of tasks using the LinkedList implementation. Tasks are enqueued using the add
method, and then processed sequentially using the poll
method within a loop.
Implementing Concurrent Queue Processing with Java Executor Framework
While the standard Queue interface serves well for basic task processing, Java provides a more advanced mechanism for concurrent processing through the Executor framework. The Executor framework, introduced in Java 5, manages thread execution and task scheduling, making it ideal for concurrent queue processing.
Here's a demonstration of concurrent queue processing using the Executor framework:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ConcurrentTaskProcessor {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3); // Create a fixed thread pool with 3 threads
Runnable task1 = () -> {
System.out.println("Processing task 1");
// Task 1 processing logic
};
Runnable task2 = () -> {
System.out.println("Processing task 2");
// Task 2 processing logic
};
Runnable task3 = () -> {
System.out.println("Processing task 3");
// Task 3 processing logic
};
// Submit tasks to the executor
executor.submit(task1);
executor.submit(task2);
executor.submit(task3);
executor.shutdown(); // Shutdown the executor after task completion
}
}
In this example, we create a fixed thread pool using Executors.newFixedThreadPool
, which allows concurrent processing of tasks. Each task is represented as a Runnable
and submitted to the executor using the submit
method. Once all tasks are submitted, the executor is shut down to release resources.
Improving Queue Processing Performance with Java Stream API
Java 8 introduced the Stream API, which provides functional-style operations for processing sequences of elements. When it comes to queue processing, the Stream API offers a concise and efficient way to perform operations on queues and their elements.
Let's see how we can leverage the Stream API for queue processing:
import java.util.Arrays;
import java.util.List;
public class QueueStreamProcessor {
public static void main(String[] args) {
List<String> taskList = Arrays.asList("Task1", "Task2", "Task3");
// Process tasks using Stream API
taskList.stream()
.forEach(task -> {
System.out.println("Processing task: " + task);
// Task processing logic
});
}
}
In this example, we use the stream
method to obtain a stream from the task list, and then we use the forEach
method to process each task in the queue. The Stream API simplifies the processing logic and offers a more declarative approach to working with queues.
To Wrap Things Up
Optimizing queue processing is pivotal for delivering efficient and scalable workflows in Java applications. By leveraging Java's Queue interface, Executor framework, and Stream API, developers can design robust and performant systems capable of handling diverse workloads.
In conclusion, understanding the nuances of queue processing and applying the right techniques can significantly enhance the performance and reliability of Java applications, ensuring a seamless workflow for both developers and end-users.
For further insights into efficient queue processing techniques, be sure to explore Java's official documentation and Concurrent Programming in Java.