Preventing LDAP Injection in Java EE6 Web Apps
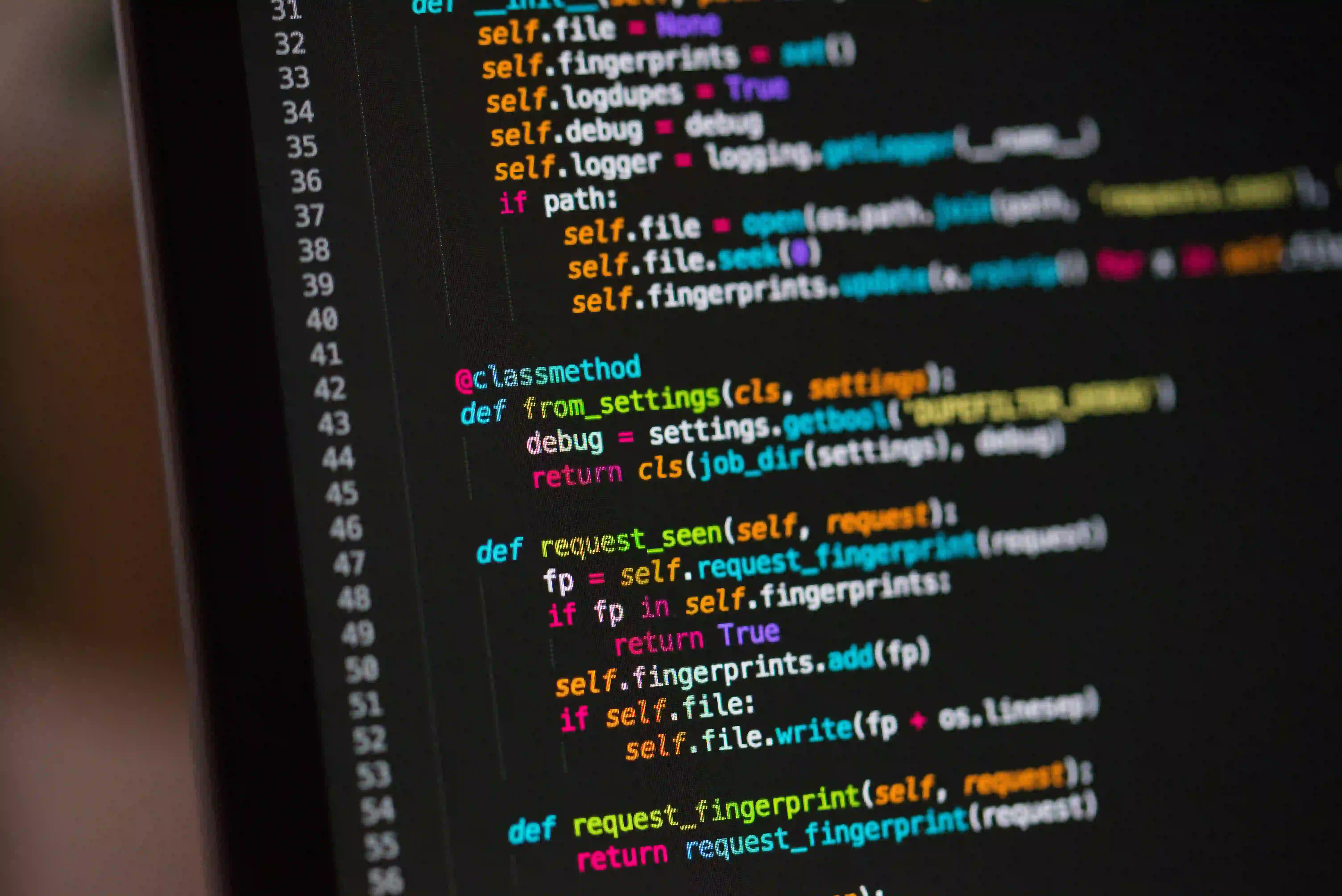
Preventing LDAP Injection in Java EE6 Web Apps
When it comes to building secure Java EE6 web applications, preventing LDAP injection is a critical aspect. LDAP injection attacks occur when untrusted data is inserted into an LDAP query, leading to potential security vulnerabilities. In this article, we'll explore the concept of LDAP injection and learn how to prevent it in Java EE6 web applications.
Understanding LDAP Injection
LDAP (Lightweight Directory Access Protocol) is commonly used for accessing and maintaining distributed directory information services. In the context of a web application, LDAP is often used for authentication and authorization purposes. When constructing an LDAP query, it's important to properly sanitize and validate user input to prevent LDAP injection attacks.
LDAP injection attacks are similar to SQL injection attacks, where malicious input is injected into a query to manipulate the application's behavior. An attacker can exploit LDAP injection to bypass authentication, access unauthorized information, or even execute arbitrary commands within the LDAP server.
Mitigating LDAP Injection in Java EE6
To mitigate LDAP injection in Java EE6 web applications, we can employ several best practices and secure coding techniques. Let's explore some of the most effective strategies for preventing LDAP injection.
1. Sanitize User Input
Always sanitize and validate user input before constructing an LDAP query. Input validation ensures that the user-supplied data adheres to the expected format and does not contain any malicious content. This can be achieved using regular expressions, input whitelisting, or input validation libraries such as Apache Commons Validator.
2. Parameterized Queries
Whenever possible, utilize parameterized queries or prepared statements to construct LDAP queries. Parameterized queries ensure that user input is treated as data rather than executable code, thereby preventing LDAP injection.
// Example of using parameterized query for LDAP search
String username = userInput;
String baseDn = "ou=users,dc=example,dc=com";
String filter = "(uid=?)";
String[] filterArgs = { username };
NamingEnumeration<SearchResult> results = ctx.search(baseDn, filter, filterArgs, searchCtls);
In the above example, the filterArgs
array holds the user-supplied input, ensuring that it's treated as a parameter rather than being directly concatenated into the LDAP filter.
3. Principle of Least Privilege
Adhere to the principle of least privilege when configuring LDAP access controls. Only grant necessary permissions to the LDAP user used by the Java EE6 application. Limit the scope of operations that the application can perform within the LDAP directory.
4. Escaping Special Characters
If it's necessary to include user input directly within an LDAP filter, ensure that special characters are properly escaped. Use LDAP-specific escape functions to sanitize user input, preventing the interpretation of input as LDAP control characters.
// Example of escaping special characters in LDAP filter
String sanitizedInput = LdapName.escapeFilter(userInput);
String filter = "(cn=" + sanitizedInput + ")";
5. Input Encoding
Apply proper input encoding to user-supplied data before using it in LDAP queries. This helps mitigate potential encoding-related LDAP injection vulnerabilities.
6. Error Handling
Implement robust error handling and logging mechanisms to capture any LDAP-related exceptions and potential injection attempts. This facilitates the detection and mitigation of LDAP injection attacks.
Final Thoughts
Preventing LDAP injection in Java EE6 web applications is paramount for safeguarding sensitive directory information and ensuring the overall security of the application. By incorporating input validation, parameterized queries, least privilege principles, character escaping, and error handling, developers can effectively mitigate the risk of LDAP injection attacks.
By following these best practices, Java EE6 developers can build resilient and secure web applications that safely interact with LDAP directories. Additionally, staying informed about emerging security threats and regularly updating the application's dependencies and libraries is crucial for maintaining a robust defense against LDAP injection and other security vulnerabilities.
In conclusion, mitigating LDAP injection in Java EE6 web applications requires a proactive approach to secure coding, thorough validation of user input, and adherence to the principle of least privilege. By integrating these strategies into the development process, developers can fortify their applications against LDAP injection attacks and uphold the integrity of their LDAP-based authentication and authorization mechanisms.
Remember, security is an ongoing process, and staying vigilant is key to ensuring the resiliency of Java EE6 web applications against evolving security threats.
For further reading on secure coding practices in Java, refer to the OWASP Secure Coding Practices and the Oracle Java EE 6 Documentation.