Improving Customer Satisfaction Through Product Excellence
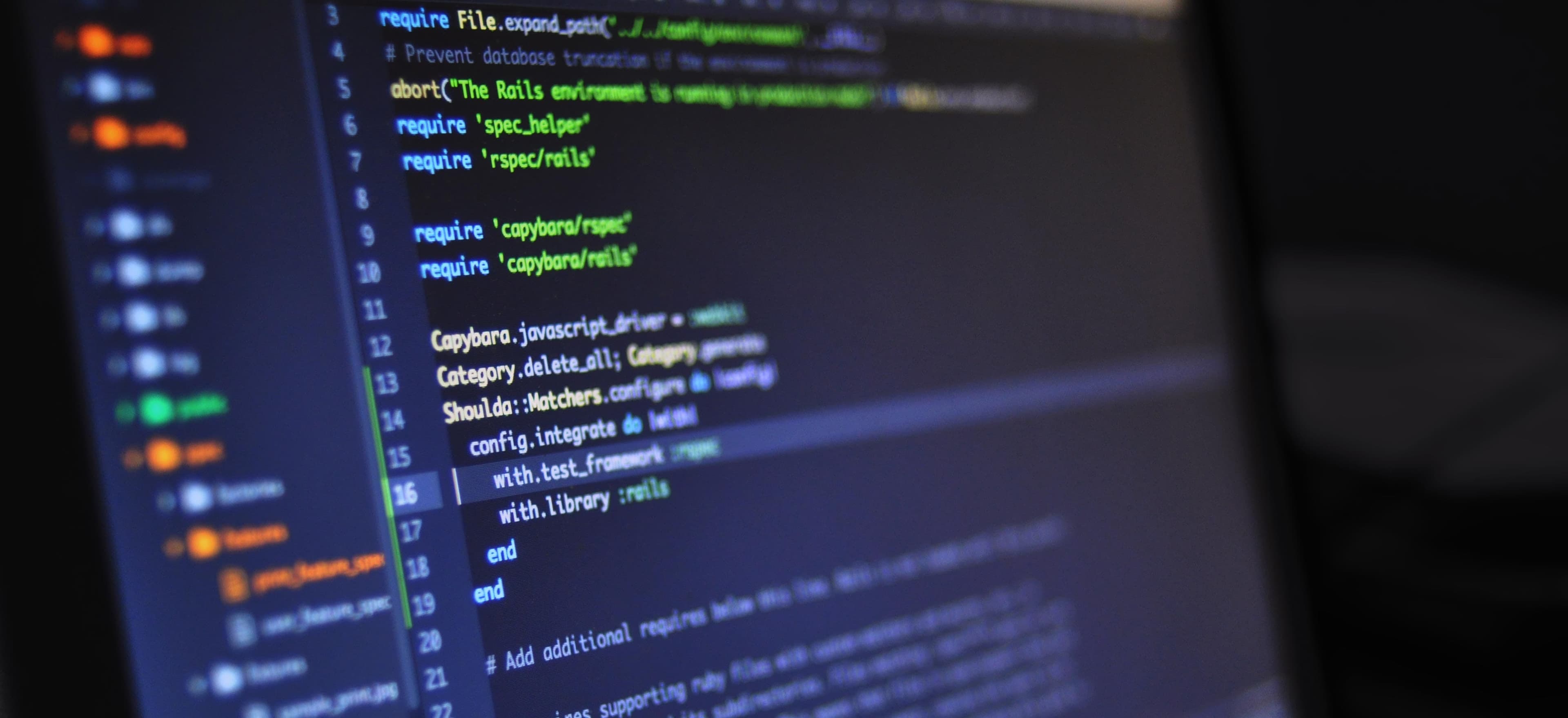
- Published on
Elevating Customer Satisfaction with Java 101
In an ever-evolving digital landscape, the bar for quality software is continually rising, and customer expectations of flawless user experiences are at an all-time high. Java, as a versatile and powerful programming language, plays a pivotal role in creating robust and high-performing applications. In this post, we'll explore how Java's diverse features and best practices can be leveraged to enhance product excellence, hence elevating customer satisfaction.
Embracing Structure with Object-Oriented Programming (OOP)
Java's support for OOP empowers developers to create modular, maintainable, and scalable codebases. Through the implementation of classes, objects, and inheritance, developers can build intuitive and organized solutions that reflect real-world entities. Let's dive into a simple example:
// Define a class
public class Car {
// Define attributes
private String make;
private String model;
// Define behavior
public void startEngine() {
System.out.println("Engine started");
}
}
In this snippet, we establish a Car
class with attributes and behavior, allowing us to encapsulate related functionalities and data. This approach fosters code reusability, making it easier to maintain and extend our codebase—ultimately contributing to product excellence and reliability.
Leveraging Java's Performance Capabilities
Java, renowned for its performance, enables the creation of high-speed and efficient applications. With features like multithreading, developers can harness the full potential of modern hardware, ensuring responsive and seamless user experiences even under demanding workloads. Let's consider a multithreading example:
// Create and start a new thread
Thread thread = new Thread(() -> {
// Perform intensive task
});
thread.start();
By employing multithreading, tasks can be concurrently executed, amplifying performance and responsiveness. This not only enhances product excellence but also enriches customer satisfaction through snappy and lag-free applications.
Enforcing Code Quality and Reliability
Maintaining code quality is paramount in delivering exceptional products. Java offers a myriad of tools and frameworks—such as Checkstyle and FindBugs—that enable developers to uphold coding standards, detect potential bugs, and ensure robustness. Let's envision how Checkstyle can elevate code consistency:
// Sample Java code
public class Sample {
private String name;
public void setName(String name) {
this.name = name;
}
}
By integrating Checkstyle within the development workflow, styling inconsistencies and potential errors can be swiftly identified and rectified, fortifying the codebase and subsequently enhancing product reliability and excellence.
Harnessing Java's Extensive Ecosystem
Java's extensive ecosystem encompasses a wealth of libraries, frameworks, and tools that expedite development and augment product quality. Leveraging popular frameworks like Spring Boot allows for the rapid construction of robust and scalable applications, thereby enriching the overall product experience. Let's visualize a simple Spring Boot REST endpoint:
// Define a REST controller
@RestController
public class ProductController {
@GetMapping("/products/{id}")
public ResponseEntity<Product> getProduct(@PathVariable Long id) {
// Retrieve and return product
}
}
By leveraging the capabilities provided by Spring Boot, developers can swiftly build and deploy feature-rich applications, showcasing the prowess of Java in augmenting product excellence and customer satisfaction.
Embracing Strong Security Measures
In an era rife with cybersecurity threats, prioritizing security is imperative in fostering customer trust and satisfaction. Java's robust security features, including its cryptographic libraries and secure coding practices, facilitate the fortification of applications against vulnerabilities and breaches. Let's explore the utilization of Java's cryptography libraries:
// Generate a secure random number
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[20];
secureRandom.nextBytes(randomBytes);
By leveraging Java's security capabilities, developers can instill confidence in customers by delivering secure applications, thereby amplifying satisfaction and loyalty.
My Closing Thoughts on the Matter
Java, with its versatile features and robust capabilities, serves as a formidable ally in the quest for product excellence and customer satisfaction. By embracing OOP principles, leveraging performance optimizations, enforcing code quality, harnessing its extensive ecosystem, and prioritizing security, Java empowers developers to craft exceptional products that delight and retain customers. As businesses navigate the competitive digital landscape, it's essential to harness Java's prowess to elevate customer satisfaction—solidifying its status as a premier language for delivering unparalleled software experiences.
Embrace Java's potential and witness the transformative impact it can have on customer satisfaction by raising the bar for product excellence.