Debugging System Tests: Containerization Challenges
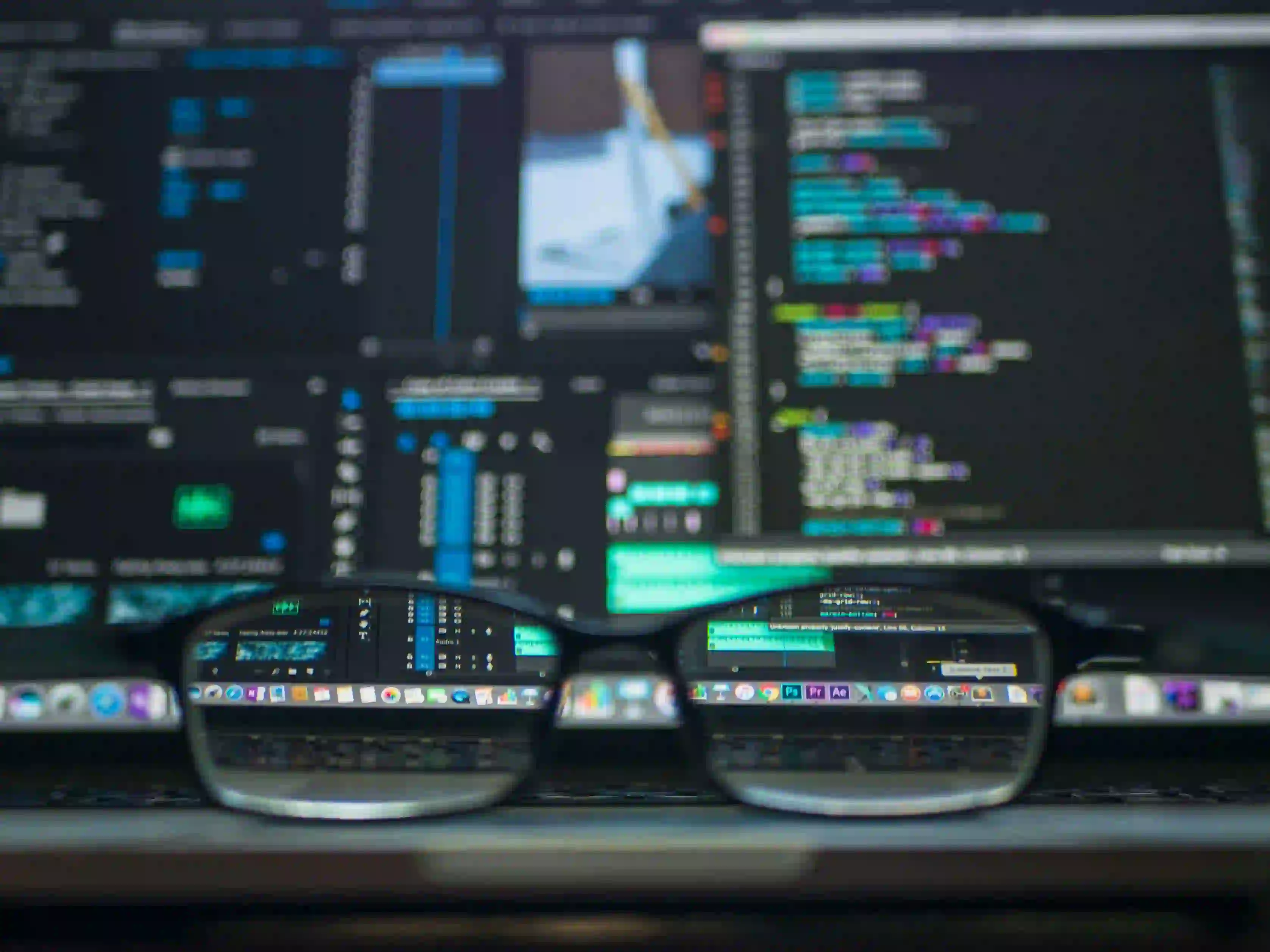
Debugging System Tests: Containerization Challenges
In the world of modern software development, containerization has become an integral part of building, shipping, and running applications. Containers have undeniably transformed the way applications are developed and deployed. However, with the rise of containerization, new challenges have emerged, particularly when it comes to debugging system tests within a containerized environment.
In this blog post, we'll delve into the complexities of debugging system tests in a containerized environment, explore the unique challenges posed by containerization, and discuss strategies to overcome these obstacles using Java.
Understanding the Containerized Environment
Containerization, popularized by platforms like Docker and Kubernetes, encapsulates an application along with its dependencies and runtime environment. While this approach offers numerous benefits such as consistency and portability, it also introduces complexities in the context of debugging system tests.
In a containerized environment, system tests are often executed within containers that mirror the production environment. This means that the test environment closely resembles the production setup, ensuring reliable results. However, this also implies that debugging issues within the containerized environment requires a different approach than traditional debugging methods.
Challenges of Debugging System Tests in Containers
Limited Visibility
When running system tests within containers, developers are often faced with restricted visibility into the test execution. The encapsulation provided by containers can make it challenging to gain insights into the inner workings of the system under test. Traditional logging and debugging tools may not provide the same level of visibility within a containerized environment.
Environment Discrepancies
Another common challenge is the variance between the development environment and the containerized test environment. Differences in configurations, dependency versions, or underlying infrastructure can lead to issues that only surface within the container, making debugging a complex task.
Isolation Constraints
Containers are designed to be isolated from each other, which can hinder the traditional debugging practices that rely on direct access to the system under test. This isolation can make it difficult to interactively troubleshoot issues or attach a debugger to the running system.
Strategies for Debugging System Tests in Containerized Environments
Leveraging Logging and Monitoring
In containerized environments, robust logging and monitoring play a crucial role in debugging system tests. Incorporating structured logging and utilizing monitoring tools such as Prometheus or Grafana can provide valuable insights into the behavior of the system under test within containers.
// Example of structured logging in Java using SLF4J
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExampleClass {
private static final Logger LOGGER = LoggerFactory.getLogger(ExampleClass.class);
public void performTask() {
LOGGER.info("Starting the task");
// Perform the task
LOGGER.info("Task completed successfully");
}
}
In the example above, structured logging using SLF4J enables clear and detailed logs, which can be invaluable when diagnosing issues within a containerized environment.
Embracing Container Orchestration Tools
Container orchestration platforms like Kubernetes offer features that aid in debugging system tests. Tools such as kubectl exec
allow developers to enter a running container and inspect its state, facilitating interactive debugging within the containerized environment.
Implementing Health Checks
Integrating health checks into the application can assist in identifying issues early on. Health checks can be used to verify the readiness and liveness of services within containers, enabling proactive detection of potential problems during system tests.
// Example of implementing a health check endpoint in a Spring Boot application
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.stereotype.Component;
@Component
public class ExampleHealthCheck implements HealthIndicator {
@Override
public Health health() {
// Check the health of the application
// Return Health.up() if healthy, else Health.down()
}
}
In the above Java code snippet, a custom health check is implemented using Spring Boot's HealthIndicator
, allowing the application to report its health status, which can be utilized during system tests.
Remote Debugging Capabilities
Some containers support remote debugging, enabling developers to attach a debugger to the running application within the container. This allows for real-time inspection and troubleshooting of issues, bridging the gap imposed by container isolation.
Closing the Chapter
Containerization has undoubtedly revolutionized the way applications are developed and deployed, but it has also brought about new challenges, particularly when it comes to debugging system tests. By leveraging logging, monitoring, container orchestration tools, health checks, and remote debugging capabilities, developers can navigate the complexities of debugging within containerized environments.
In the context of Java, adopting best practices for logging, implementing health checks, and exploring container orchestration features can significantly enhance the debugging process within containerized environments. As containerization continues to evolve, so too will the strategies and tools available for effective system test debugging.
In conclusion, while debugging system tests within containerized environments presents unique challenges, proactive implementation of appropriate strategies can empower developers to effectively diagnose and resolve issues, ensuring the reliability and robustness of containerized applications.