Compactness Benefits of Java 8 Lambdas
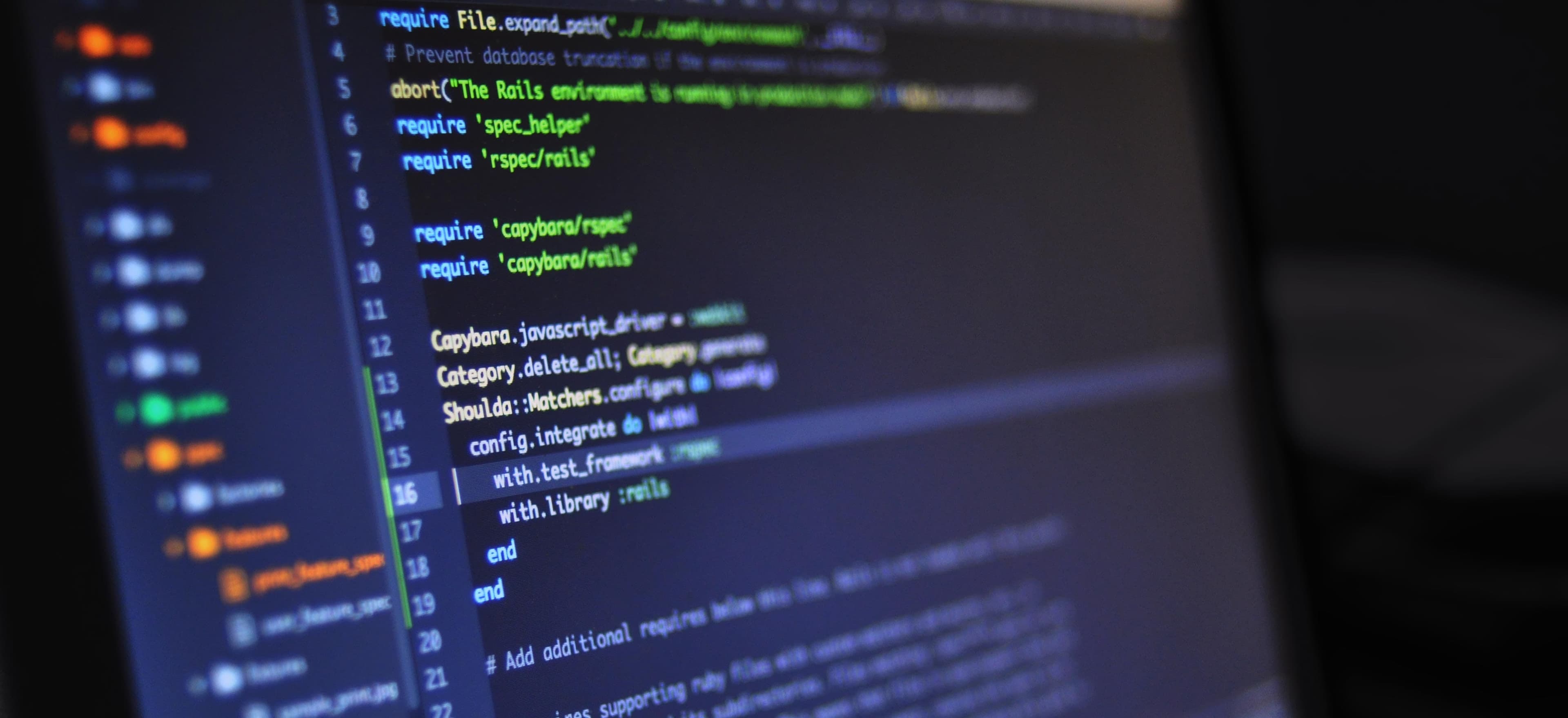
- Published on
Understanding the Compactness Benefits of Java 8 Lambdas
In the world of Java programming, the introduction of lambdas in Java 8 has been a game-changer. Lambdas provide a concise way to represent a piece of functionality to be executed later, enabling developers to write shorter and more readable code.
In this article, we'll explore the compactness benefits of Java 8 lambdas and how they can improve your code.
What are Java 8 Lambdas?
Java 8 introduced the concept of functional programming by adding support for lambda expressions, also known as closures. A lambda expression is an anonymous function that allows you to pass behavior as an argument to a method. Essentially, it's a way to write short blocks of code that can be passed around as data.
Let's dive into the benefits of using Java 8 lambdas in your code.
Compact and Readable Code
One of the primary benefits of using Java 8 lambdas is the ability to write more compact and readable code. Traditionally, implementing functional interfaces (interfaces with a single abstract method) required verbose anonymous inner classes. Lambdas provide a much more concise syntax for achieving the same result.
Consider the following example where we want to sort a list of strings in alphabetical order using the Comparator
interface:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Prior to Java 8
Collections.sort(names, new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
});
// Using Java 8 lambda
Collections.sort(names, (s1, s2) -> s1.compareTo(s2));
In this example, the lambda expression (s1, s2) -> s1.compareTo(s2)
is much more compact and easier to read than the anonymous inner class version. This results in cleaner and more maintainable code.
Improved API Libraries
Java 8 introduced a variety of new methods in the JDK that accept functional interfaces. The Streams API, for example, is designed to work seamlessly with lambdas, enabling developers to process collections in a more concise and expressive manner.
Consider the following code snippet, where we filter a list of numbers to get only the even ones using the Streams API:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
In this example, the lambda expression n -> n % 2 == 0
passed to the filter
method succinctly captures the logic for filtering even numbers. This leads to more expressive and elegant code that is easier to understand.
Enhanced Event Handling
Lambdas have greatly simplified event handling in graphical user interfaces (GUI) and other interactive applications. Instead of implementing numerous event listener interfaces, you can now use lambdas to directly specify the behavior in response to events.
For instance, when handling button clicks in a JavaFX application, using lambdas can significantly reduce the boilerplate code required:
Button button = new Button("Click Me");
// Prior to Java 8
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
System.out.println("Button clicked");
}
});
// Using Java 8 lambda
button.setOnAction(event -> System.out.println("Button clicked"));
The lambda expression event -> System.out.println("Button clicked")
offers a much more succinct and clear representation of the event handling behavior.
Deferred Execution
Lambdas support deferred execution, allowing you to represent a block of code that can be executed later. This flexibility is particularly useful for asynchronous programming and executing code in response to certain conditions.
For example, lambdas are commonly used with the java.util.concurrent
package to specify tasks to be executed by worker threads. This allows for cleaner and more compact code when dealing with concurrent operations.
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.submit(() -> System.out.println("Task running in a separate thread"));
The lambda expression () -> System.out.println("Task running in a separate thread")
captures the task to be executed by the thread pool, providing a clear and concise representation of the code to be run asynchronously.
The Bottom Line
In conclusion, Java 8 lambdas offer significant benefits in terms of code compactness, readability, and expressiveness. They enable developers to write more concise and maintainable code, leverage enhanced API libraries, simplify event handling, and support deferred execution for asynchronous programming.
By embracing lambdas, you can take advantage of the modern functional programming capabilities in Java and write more elegant and efficient code.
Now that you understand the compactness benefits of Java 8 lambdas, consider integrating them into your Java projects to reap the advantages they offer.
Continue honing your skills with Java 8 lambdas, make your code more readable and concise, and explore the possibilities they unlock for your development projects.
Happy coding!
References:
Checkout our other articles