The Pitfalls of Implementing Java Singleton Pattern
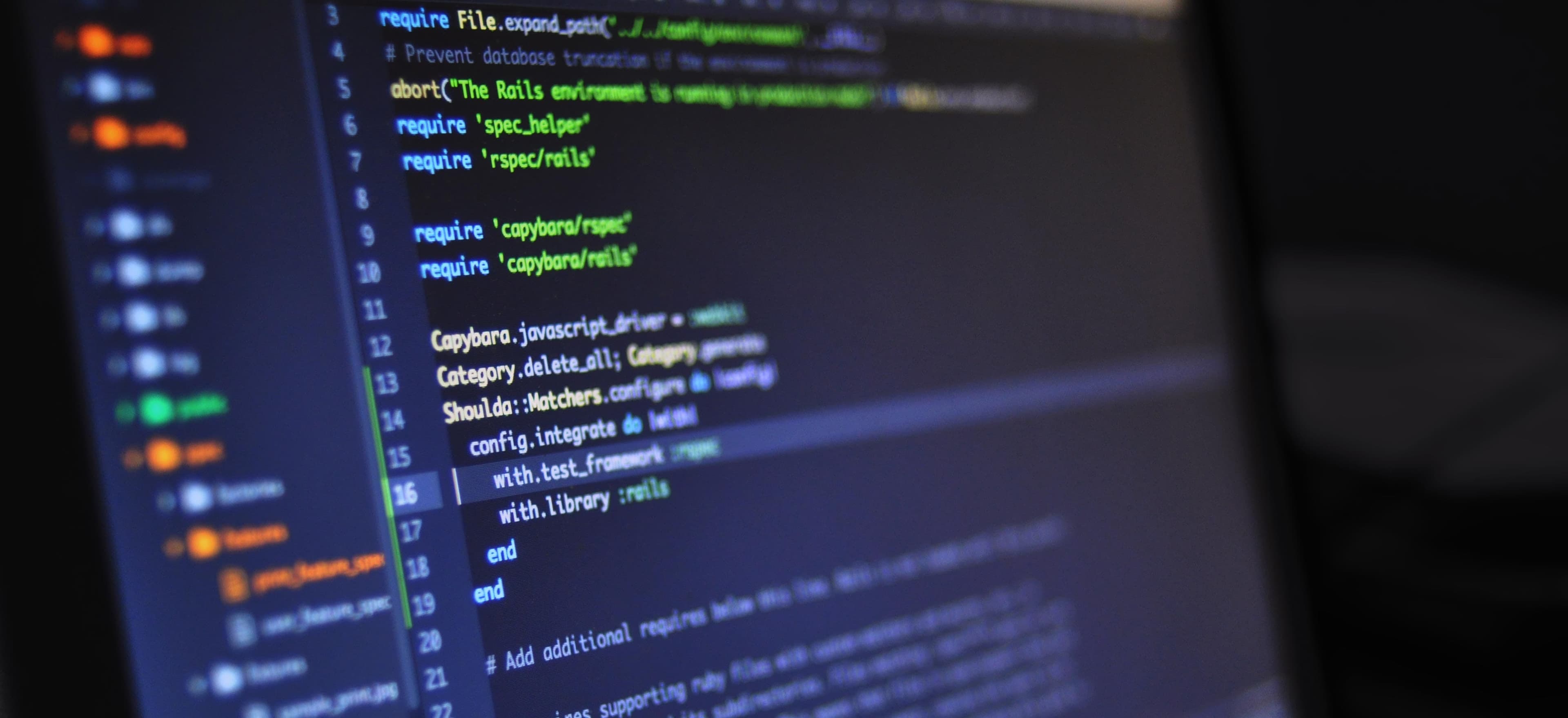
- Published on
The Pitfalls of Implementing Java Singleton Pattern
In Java, the Singleton pattern is a design pattern used to ensure that a class has only one instance and provides a global point of access to that instance. While it has its advantages, improper implementation can lead to pitfalls that may affect the performance, concurrency, and maintainability of the code. In this article, we will explore the common pitfalls of implementing the Singleton pattern in Java and discuss best practices to avoid them.
Understanding the Singleton Pattern
The Singleton pattern restricts the instantiation of a class to a single object. This is achieved by making the constructor private and providing a static method to access the instance of the class. The Singleton pattern is often used in scenarios where only one instance of a class is required, such as in logging, database connections, and configuration settings.
public class Singleton {
private static Singleton instance;
private Singleton() {
// private constructor to prevent instantiation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Pitfalls of Singleton Implementation
1. Multithreading Issues
The most common pitfall of implementing the Singleton pattern in Java is related to multithreading. In a multithreaded environment, multiple threads accessing the getInstance()
method concurrently may create multiple instances of the Singleton, violating the pattern's intent.
To mitigate this issue, we can use the double-checked locking mechanism or rely on the classloader to ensure thread-safety during the initialization of the Singleton instance.
public class ThreadSafeSingleton {
private static ThreadSafeSingleton instance;
private ThreadSafeSingleton() {
// private constructor to prevent instantiation
}
public static ThreadSafeSingleton getInstance() {
if (instance == null) {
synchronized (ThreadSafeSingleton.class) {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
2. Serialization Problem
Another pitfall is related to the serialization of Singleton objects. When a Singleton class is serialized and then deserialized, it creates a new instance, breaking the Singleton contract. To address this, we can implement the readResolve()
method to ensure that the deserialization process returns the existing Singleton instance.
public class SerializableSingleton implements Serializable {
private static final long serialVersionUID = 1L;
private static SerializableSingleton instance;
private SerializableSingleton() {
// private constructor to prevent instantiation
}
public static SerializableSingleton getInstance() {
if (instance == null) {
instance = new SerializableSingleton();
}
return instance;
}
protected Object readResolve() {
return instance;
}
}
3. Classloaders in a Distributed Environment
In a distributed environment with multiple classloaders, there is a risk of creating multiple instances of a Singleton if the class is loaded by different classloaders. To address this, we can use the Enum
approach for Singleton instantiation, as enums in Java are implicitly final
and static
.
public enum EnumSingleton {
INSTANCE;
public void someMethod() {
// perform some action
}
}
Best Practices for Singleton Implementation
To avoid the pitfalls mentioned above, here are some best practices for implementing the Singleton pattern in Java:
-
Use Enum for Singletons: As mentioned, using the enum approach for Singleton instantiation ensures that the Singleton is thread-safe, prevents multiple instantiations, and handles serialization and classloader issues.
-
Lazy Initialization: If the Singleton instance is resource-intensive and not always required, consider lazy initialization to create the instance only when needed.
-
Use Bill Pugh's Solution: The Bill Pugh Singleton implementation uses a static inner helper class to create the Singleton instance, providing lazy initialization and thread-safety without the need for synchronized blocks.
public class BillPughSingleton {
private BillPughSingleton() {
// private constructor to prevent instantiation
}
private static class SingletonHelper {
private static final BillPughSingleton INSTANCE = new BillPughSingleton();
}
public static BillPughSingleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
Final Considerations
While the Singleton pattern can be a powerful tool in Java development, implementing it improperly can lead to various pitfalls that may impact the application's performance and stability. By understanding the challenges associated with Singleton implementation and following best practices, developers can ensure the effective and reliable use of the Singleton pattern in their Java applications.