Maximizing Production Efficiency with Selenium Automation Testing
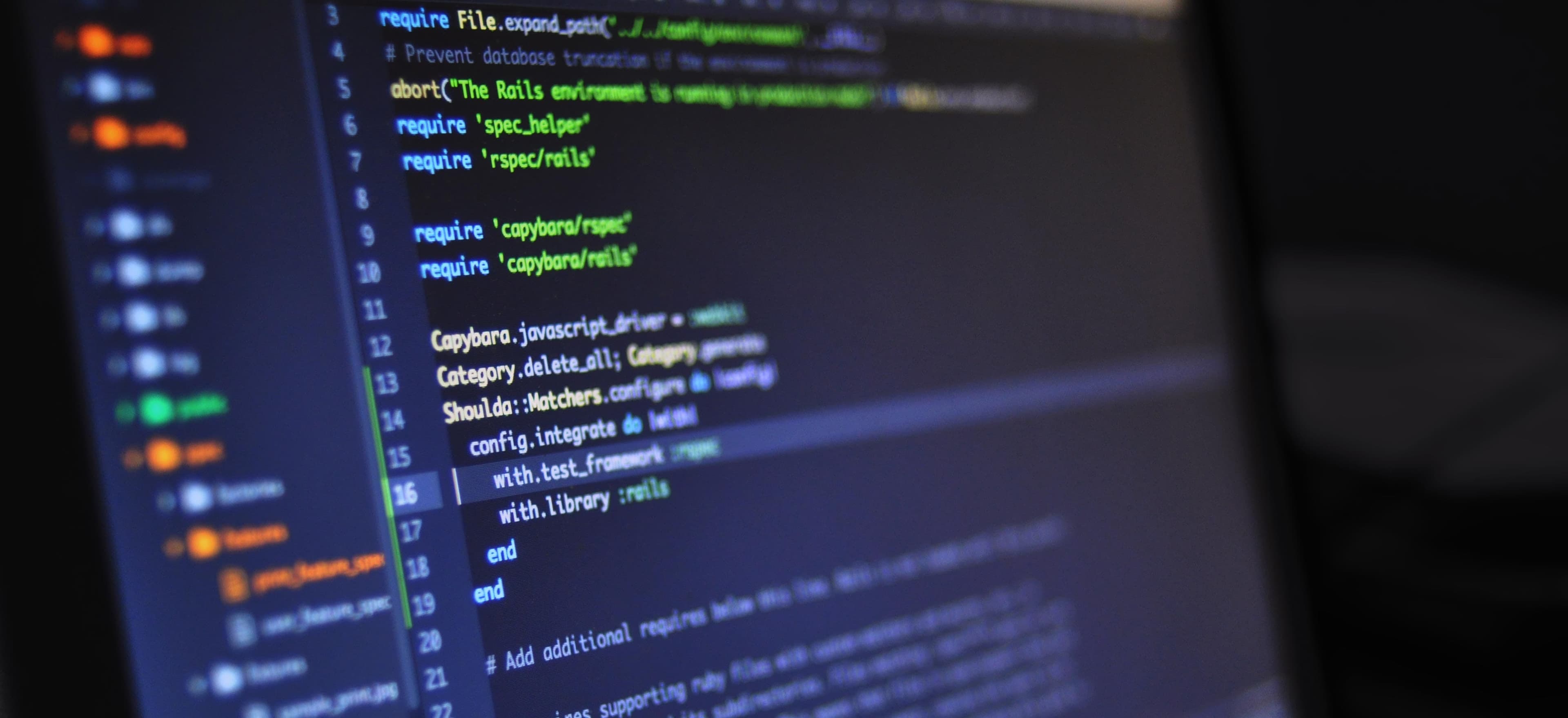
- Published on
Maximizing Production Efficiency with Selenium Automation Testing
In today's fast-paced software development industry, ensuring the efficiency of the production process is crucial for meeting deadlines and delivering high-quality products. One essential aspect of achieving efficiency is implementing robust and reliable testing procedures. This is where Selenium, an open-source automation tool, plays a significant role in streamlining the testing process.
Let us Begin to Selenium
Selenium is a powerful framework that allows for the automation of web browsers. It provides a set of tools that support the automation of web applications for testing purposes, simplifying the validation of functionality and performance across different browsers and platforms.
Advantages of Selenium
-
Cross-browser Compatibility: Selenium allows tests to be executed across various browsers, ensuring the consistency of web application behavior.
-
Language Support: It offers support for multiple programming languages such as Java, Python, C#, and more, providing flexibility for developers to write automated tests in their preferred language.
-
Integration Capabilities: Selenium seamlessly integrates with other tools and frameworks, allowing for the creation of comprehensive test suites.
Using Selenium for Production Efficiency
1. Test Suite Automation
Code Sample:
@Test
public void loginTest() {
// Navigate to the login page
driver.get("https://example.com/login");
// Perform login actions
WebElement usernameField = driver.findElement(By.id("username"));
WebElement passwordField = driver.findElement(By.id("password"));
WebElement loginButton = driver.findElement(By.id("loginButton"));
usernameField.sendKeys("user123");
passwordField.sendKeys("pass456");
loginButton.click();
// Validate login success
assertTrue(driver.getCurrentUrl().equals("https://example.com/dashboard"));
}
Why: Automating test suites with Selenium reduces the manual effort required for regression testing, allowing developers to focus on coding and feature development. As a result, production efficiency is significantly enhanced.
2. Continuous Integration with Selenium
Continuous Integration (CI) enables the automation of the build and testing process whenever code changes are committed. By integrating Selenium tests into CI pipelines using tools like Jenkins or CircleCI, the testing process becomes an integral part of the development cycle, ensuring the early detection of issues and maintaining the production efficiency.
3. Parallel Testing
Code Sample:
@Test
public void parallelTestExecution() {
// Instantiate multiple driver instances
WebDriver driver1 = new ChromeDriver();
WebDriver driver2 = new FirefoxDriver();
// Execute tests in parallel
driver1.get("https://example.com");
driver2.get("https://example.com");
// Perform assertions and cleanup
// ...
// Quit drivers
driver1.quit();
driver2.quit();
}
Why: Parallel testing using Selenium allows for simultaneous execution of tests across different browsers, drastically reducing the overall test execution time and improving production efficiency.
Best Practices for Efficient Selenium Testing
1. Maintain a Clear Test Structure
Organizing test cases and suites with a clear and understandable structure ensures maintainability and scalability, contributing to the overall efficiency of the testing process.
2. Prioritize Test Coverage
Focusing on critical functionality and high-traffic areas of the application for automation ensures that the test suite delivers maximum value in terms of identifying issues that could impact production efficiency.
3. Implement Page Object Model
Code Sample:
public class LoginPage {
private WebDriver driver;
// Define page elements
private By usernameField = By.id("username");
private By passwordField = By.id("password");
private By loginButton = By.id("loginButton");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
// Reusable actions on the page
public void enterUsername(String username) {
driver.findElement(usernameField).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(passwordField).sendKeys(password);
}
public void clickLoginButton() {
driver.findElement(loginButton).click();
}
}
Why: By implementing the Page Object Model, test maintenance becomes more efficient as changes in the application's UI can be localized within the page objects, reducing the impact on the test code and improving overall productivity.
My Closing Thoughts on the Matter
Selenium automation testing serves as a pivotal tool in maximizing production efficiency by enabling the automation of repetitive tasks, integration with continuous integration pipelines, and facilitating parallel testing. By adhering to best practices and leveraging the capabilities of Selenium, development teams can streamline their testing processes, leading to accelerated delivery timelines and enhanced software quality.
Incorporating Selenium into the testing workflow not only benefits the development team but also contributes to the overall success of the product by ensuring a robust and reliable application that meets user expectations.
To explore more about Selenium and its features, check out the official Selenium website and Selenium WebDriver documentation. Happy testing!