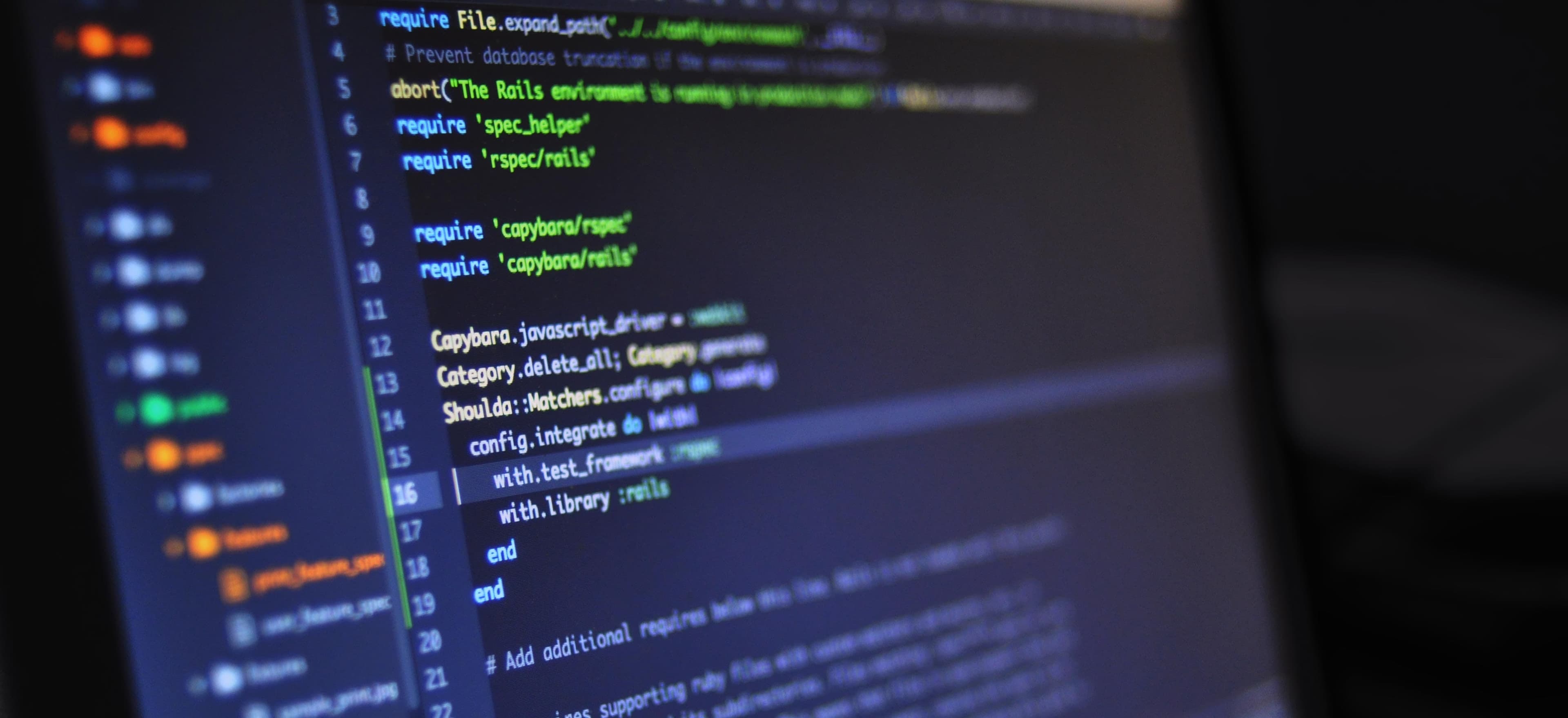
- Published on
Effective Strategies for Testing Data Access Code
When developing Java applications, testing data access code is crucial to ensure the stability and reliability of the overall system. In this article, we will explore effective strategies for testing data access code in Java, including unit testing with JUnit, integration testing with a database, and using mocking frameworks like Mockito.
Why Test Data Access Code?
Testing data access code is essential for several reasons:
- Reliability: Verifying that data access code functions as expected ensures the reliability of the application.
- Isolation: Testing data access code in isolation from the rest of the application helps identify and fix issues more effectively.
- Continuous Integration: Automated tests for data access code facilitate continuous integration and deployment processes.
Now, let's delve into the strategies for testing data access code effectively.
Unit Testing with JUnit
Unit testing focuses on testing individual units of code in isolation. When testing data access code, we want to ensure that each method or function works as intended.
Example: Unit Testing a Data Access Class with JUnit
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.*;
@RunWith(MockitoJUnitRunner.class)
public class UserDaoTest {
@Mock
private Connection mockConnection;
@InjectMocks
private UserDao userDao;
@Test
public void testGetUserName() throws SQLException {
// Mocking the PreparedStatement
PreparedStatement mockPreparedStatement = mock(PreparedStatement.class);
when(mockConnection.prepareStatement(anyString())).thenReturn(mockPreparedStatement);
// Creating a ResultSet with expected data
ResultSet mockResultSet = mock(ResultSet.class);
when(mockPreparedStatement.executeQuery()).thenReturn(mockResultSet);
when(mockResultSet.next()).thenReturn(true);
when(mockResultSet.getString("name")).thenReturn("John Doe");
String userName = userDao.getUserName(123);
assertEquals("John Doe", userName);
}
}
In this example, we use JUnit and Mockito to test a UserDao
class. We mock the database connection and prepare statements to isolate the testing of the getUserName
method. This allows us to verify the behavior of the data access code without actually hitting the database.
Integration Testing with a Database
While unit testing is effective for isolating and testing individual units of code, it's also crucial to perform integration testing to validate the interaction between the application and the database.
Example: Integration Testing with H2 Database
Integration testing with a real database can be achieved using an in-memory database like H2. The following is an example configuration for using H2 for integration testing with Spring Boot.
@Profile("test")
@Configuration
public class TestDatabaseConfig {
@Bean
public DataSource dataSource() {
return new EmbeddedDatabaseBuilder()
.setType(EmbeddedDatabaseType.H2)
.addScript("classpath:schema.sql")
.build();
}
}
In this example, we configure a test-specific DataSource
using H2 that initializes the database schema from a schema.sql
file. This allows us to perform integration tests against a real database without affecting the development or production databases.
Using Mocking Frameworks like Mockito
Mocking frameworks like Mockito are valuable tools for simulating the behavior of dependencies, such as database connections or external services, during testing.
Example: Mocking a Database Connection with Mockito
public class UserDatabaseServiceTest {
@Test
public void testGetUserName() {
Connection mockConnection = mock(Connection.class);
PreparedStatement mockPreparedStatement = mock(PreparedStatement.class);
ResultSet mockResultSet = mock(ResultSet.class);
when(mockConnection.prepareStatement(anyString())).thenReturn(mockPreparedStatement);
when(mockPreparedStatement.executeQuery()).thenReturn(mockResultSet);
when(mockResultSet.next()).thenReturn(true);
when(mockResultSet.getString("name")).thenReturn("Jane Smith");
UserDatabaseService userDatabaseService = new UserDatabaseService(mockConnection);
String userName = userDatabaseService.getUserName(456);
assertEquals("Jane Smith", userName);
}
}
In this example, we use Mockito to mock the database connection and simulate the behavior of preparing a statement and executing a query. This allows us to test the UserDatabaseService
class in isolation and verify its interaction with the database without actually connecting to a real database.
Closing the Chapter
Testing data access code is critical for ensuring the stability and reliability of Java applications. By leveraging strategies such as unit testing with JUnit, integration testing with a database, and using mocking frameworks like Mockito, developers can effectively test data access code and build robust, maintainable systems.
Testing data access code not only improves the quality of the application but also provides confidence in making changes and refactoring, ultimately leading to a more maintainable and scalable codebase.
By adopting these effective testing strategies, developers can enhance the overall quality and robustness of their Java applications, setting the stage for successful deployment and maintenance.
To delve deeper into testing data access code using JUnit, Mockito, and testing frameworks like H2, check out the following resources:
Incorporate these testing strategies into your Java development workflow to build resilient and reliable data access code for your applications. Happy testing!