Overcoming Common Research Challenges in Agile Transition
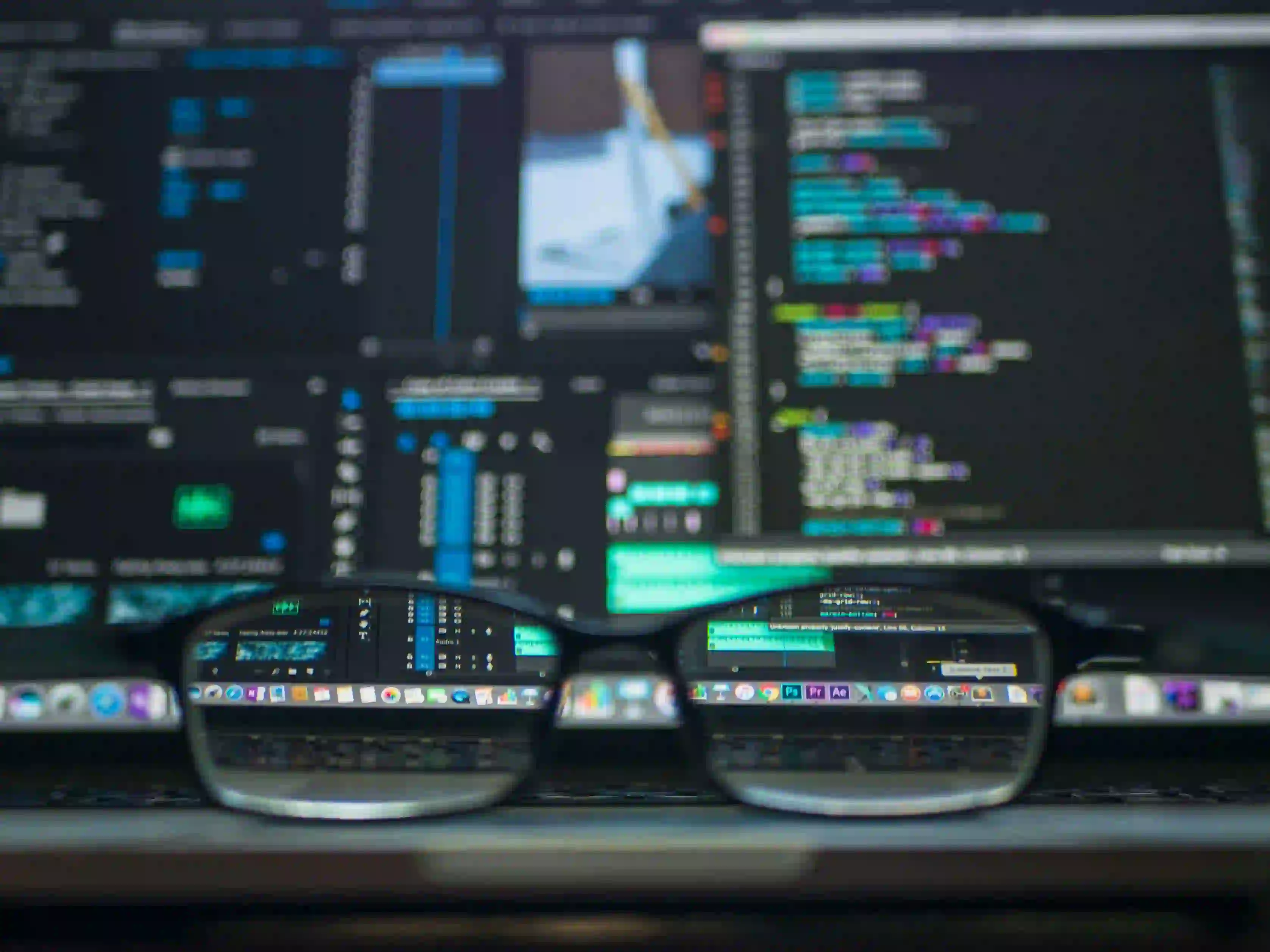
Overcoming Common Research Challenges in Agile Transition
In the world of software development, Agile has become the go-to methodology for businesses looking to develop high-quality software in a more efficient and flexible manner. Transitioning to Agile, however, is not without its challenges. In this article, we will discuss some of the common research challenges encountered when transitioning to Agile and how to overcome them using Java.
Understanding Agile Methodology
Before delving into the research challenges, let's first understand the basics of Agile methodology. Agile emphasizes iterative development, cross-functional teams, customer collaboration, and adaptability to change. It promotes a flexible and evolutionary approach to software development, allowing for continuous improvement and the ability to respond to feedback.
Common Research Challenges in Agile Transition
1. Lack of Clarity on Agile Practices
When transitioning to Agile, teams often struggle with a lack of clarity on Agile practices. This can lead to confusion and inefficiency in the development process. Researching and understanding Agile principles and practices is crucial for a successful transition.
2. Choosing the Right Tools and Frameworks
Selecting the appropriate tools and frameworks compatible with Agile practices is another common challenge. With the abundance of options available, it is essential to research and choose tools that seamlessly integrate with the Agile workflow.
3. Maintaining Code Quality
Maintaining code quality in Agile development is paramount. Without proper research and implementation of best practices, the codebase can quickly become unmanageable. Researching code quality tools and establishing coding standards is vital.
4. Balancing Flexibility and Stability
Agile promotes flexibility, but maintaining stability in the codebase is equally important. Striking a balance between the two can be challenging and requires thorough research into best practices and architectural patterns.
Overcoming Research Challenges with Java
Java, being one of the most prominent languages in Agile development, provides robust solutions to overcome these research challenges. Let's explore how Java can address each of the aforementioned challenges:
1. Lack of Clarity on Agile Practices
Java offers an extensive range of frameworks and libraries that align with Agile practices. For instance, Spring Framework facilitates building robust and scalable applications following Agile principles. By researching and leveraging such frameworks, teams can gain clarity on Agile practices.
2. Choosing the Right Tools and Frameworks
Java’s rich ecosystem includes a variety of tools and frameworks tailored for Agile development. Tools like Maven for build automation, JUnit for testing, and Jenkins for continuous integration offer comprehensive support for Agile methodologies. Through research and evaluation, teams can select the most suitable tools for their Agile projects.
3. Maintaining Code Quality
Java presents a plethora of tools to ensure code quality, such as Checkstyle for code style checking, PMD for source code analysis, and SonarQube for continuous inspection. By conducting thorough research and integrating these tools into the development process, teams can uphold high code quality standards in their Agile projects.
4. Balancing Flexibility and Stability
Java’s design principles, particularly its support for object-oriented programming and design patterns, contribute to striking a balance between flexibility and stability. Researching and applying design patterns like the Strategy Pattern and the Observer Pattern can aid in establishing a flexible yet stable codebase.
Implementing Java Solutions
Let's delve into some exemplary Java code snippets with commentary on how they address the research challenges:
Using Spring Framework for Agile Practices
@RestController
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
return ResponseEntity.ok(user);
}
}
In this code snippet, the use of Spring Framework facilitates the creation of a RESTful endpoint, aligning with Agile’s emphasis on iterative development and customer collaboration.
Leveraging Maven for Build Automation
<project>
...
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
...
</project>
The Maven configuration exemplifies how Java leverages build automation to adhere to Agile’s focus on efficient and consistent builds.
Integrating Checkstyle for Code Quality
<build>
...
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.1</version>
<dependencies>
<dependency>
<groupId>com.puppycrawl.tools</groupId>
<artifactId>checkstyle</artifactId>
<version>8.44</version>
</dependency>
</dependencies>
<configuration>
<configLocation>checkstyle.xml</configLocation>
</configuration>
</plugin>
</plugins>
...
</build>
This snippet showcases the integration of Checkstyle into the build process, emphasizing Java’s commitment to maintaining high code quality standards in Agile development.
Applying Design Patterns for Flexibility and Stability
public interface NotificationStrategy {
void sendNotification(String message);
}
By defining a NotificationStrategy interface, Java promotes the use of design patterns to achieve flexibility and stability, in line with Agile’s principles.
My Closing Thoughts on the Matter
Transitioning to Agile comes with its set of research challenges, but Java offers robust solutions to overcome them. By understanding and leveraging Java’s rich ecosystem of tools, frameworks, and best practices, teams can effectively navigate the complexities of Agile development. Research, experimentation, and continuous learning form the cornerstone of a successful Agile transition with Java at the helm.