Integrating Java and Scala for Event Calendars
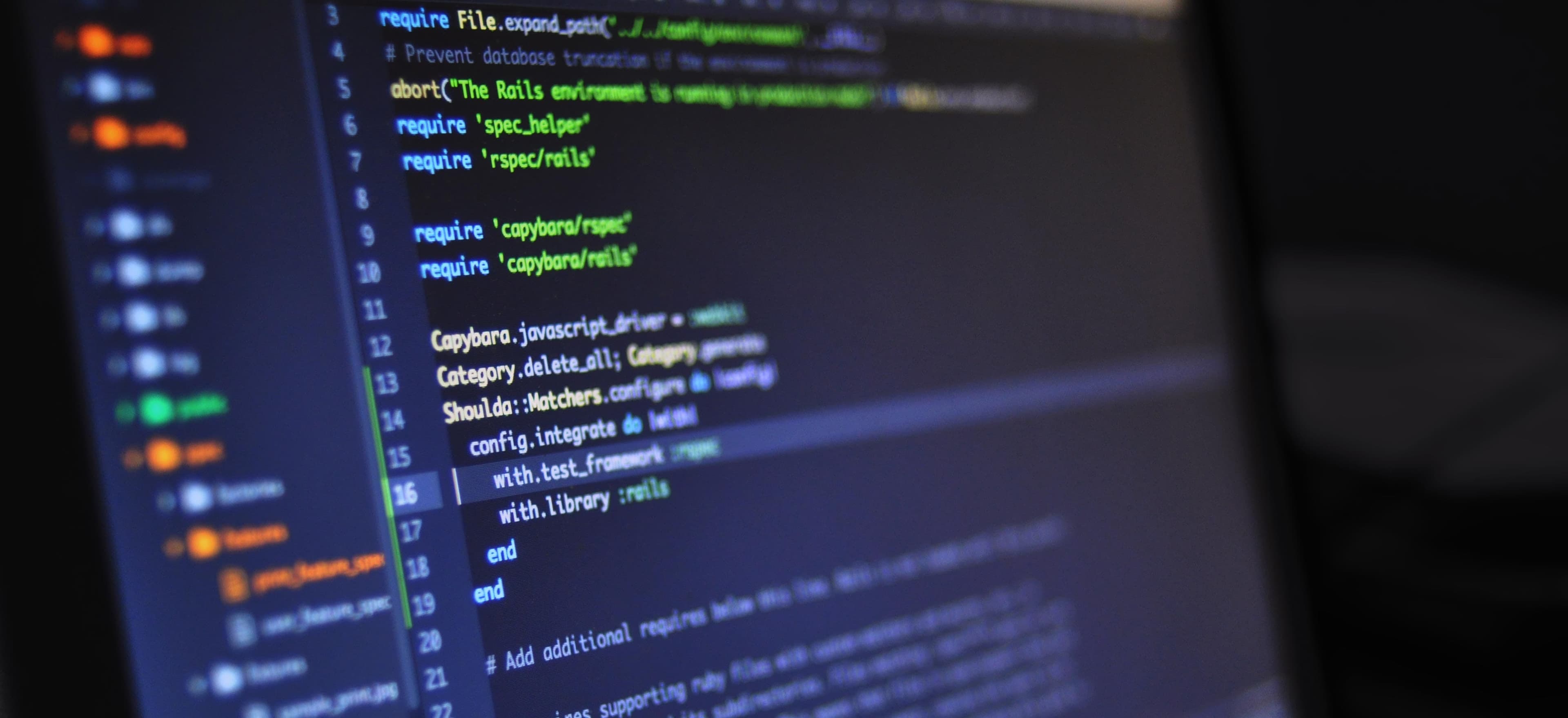
- Published on
Leveraging the Power of Java and Scala for Event Calendars
Event calendars are an essential aspect of many applications, whether it's for scheduling events, managing appointments, or organizing tasks. In this blog post, we'll explore how to leverage the power of Java and Scala to build a robust and efficient event calendar system. By combining the strengths of both languages, we can create an event calendar that is both scalable and high-performing.
Why Java and Scala?
Java is a widely-used, versatile programming language with a strong ecosystem and excellent support for building enterprise-level applications. On the other hand, Scala, as a language that runs on the Java Virtual Machine (JVM), provides functional programming capabilities, concise syntax, and seamless interoperability with Java. When used in conjunction, Java and Scala offer a powerful combination for building complex systems.
Setting Up the Project
To begin, let's set up a new project using Maven, a popular build automation tool primarily used for Java projects. We'll utilize Maven to manage our project dependencies and build process, ensuring a smooth integration of Java and Scala.
First, create a new Maven project and add the necessary dependencies for Scala using the following pom.xml
configuration:
<dependencies>
<dependency>
<groupId>org.scala-lang</groupId>
<artifactId>scala-library</artifactId>
<version>2.12.8</version>
</dependency>
</dependencies>
With our project structure in place, we can now start integrating Java and Scala to build our event calendar system.
Integrating Java and Scala
One of the key advantages of using Scala in conjunction with Java is its seamless interoperability. This means that we can make use of Scala's features and functional programming capabilities while still being able to interact with Java code.
Let's consider a scenario where we want to define the structure of an event in our calendar. We can create a Java class Event
with the following properties:
public class Event {
private String title;
private LocalDateTime startTime;
private LocalDateTime endTime;
// Constructor, getters, and setters
}
In Scala, we can then create a companion object for the Event
class, providing additional functionality using Scala's features. For example, we can define a method that calculates the duration of the event:
object Event {
def calculateDuration(event: Event): Duration = {
Duration.between(event.startTime, event.endTime)
}
}
Here, we've leveraged Scala's concise syntax and functional programming capabilities to define a method for calculating the duration of an event. This is just one example of how we can harness the strengths of both Java and Scala within our event calendar system.
Leveraging Scala's Pattern Matching
Scala's pattern matching is a powerful feature that allows for concise and expressive code. We can leverage pattern matching to handle different types of events within our calendar. For instance, we may want to distinguish between different categories of events, such as meetings, appointments, and deadlines.
In our Scala code, we can define a sealed trait CalendarEvent
to represent different types of events:
sealed trait CalendarEvent
case class Meeting(title: String, startTime: LocalDateTime, endTime: LocalDateTime, attendees: List[String]) extends CalendarEvent
case class Appointment(title: String, startTime: LocalDateTime, endTime: LocalDateTime, location: String) extends CalendarEvent
case class Deadline(title: String, dueTime: LocalDateTime) extends CalendarEvent
By using pattern matching, we can easily handle each type of event and perform specific actions based on its category. This not only improves the readability of our code but also allows for comprehensive event handling within our calendar system.
Consuming External APIs
In real-world applications, event calendar systems often need to interact with external APIs for functionalities such as retrieving weather information for event locations, sending notifications, or integrating with third-party services. Scala's support for asynchronous and non-blocking I/O makes it well-suited for consuming external APIs and handling concurrent operations efficiently.
We can use Scala's Future
to perform asynchronous operations and seamlessly integrate them into our Java-based event calendar system. For example, let's consider a scenario where we want to retrieve weather information for a given location when scheduling an event.
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
object WeatherAPI {
def getWeather(location: String): Future[Weather] = {
// Asynchronous operation to retrieve weather information
}
}
Here, we've defined a WeatherAPI
object that uses Scala's Future
to perform an asynchronous operation for retrieving weather information. By leveraging Scala's concurrency features, we can seamlessly integrate such operations into our event calendar system while ensuring optimal performance.
Closing Remarks
In this blog post, we've explored the seamless integration of Java and Scala for building an efficient and robust event calendar system. By combining the strengths of both languages, we can leverage Java's ecosystem and Scala's functional programming capabilities to create a high-performing solution.
Throughout our exploration, we've seen how Scala's features such as pattern matching and asynchronous I/O can enhance the functionality and maintainability of our event calendar system. By harnessing the power of Java and Scala, we can build event calendars that are not only feature-rich but also scalable and reliable.
In conclusion, the combined use of Java and Scala provides a compelling approach for developing event calendars and similar systems, offering a harmonious blend of productivity, performance, and expressive power.
Additional Resources:
Remember, the right tools and the appropriate language goes a long way in crafting the perfect solution!