Building Java Code Models: Overcoming Source and JAR File Challenges
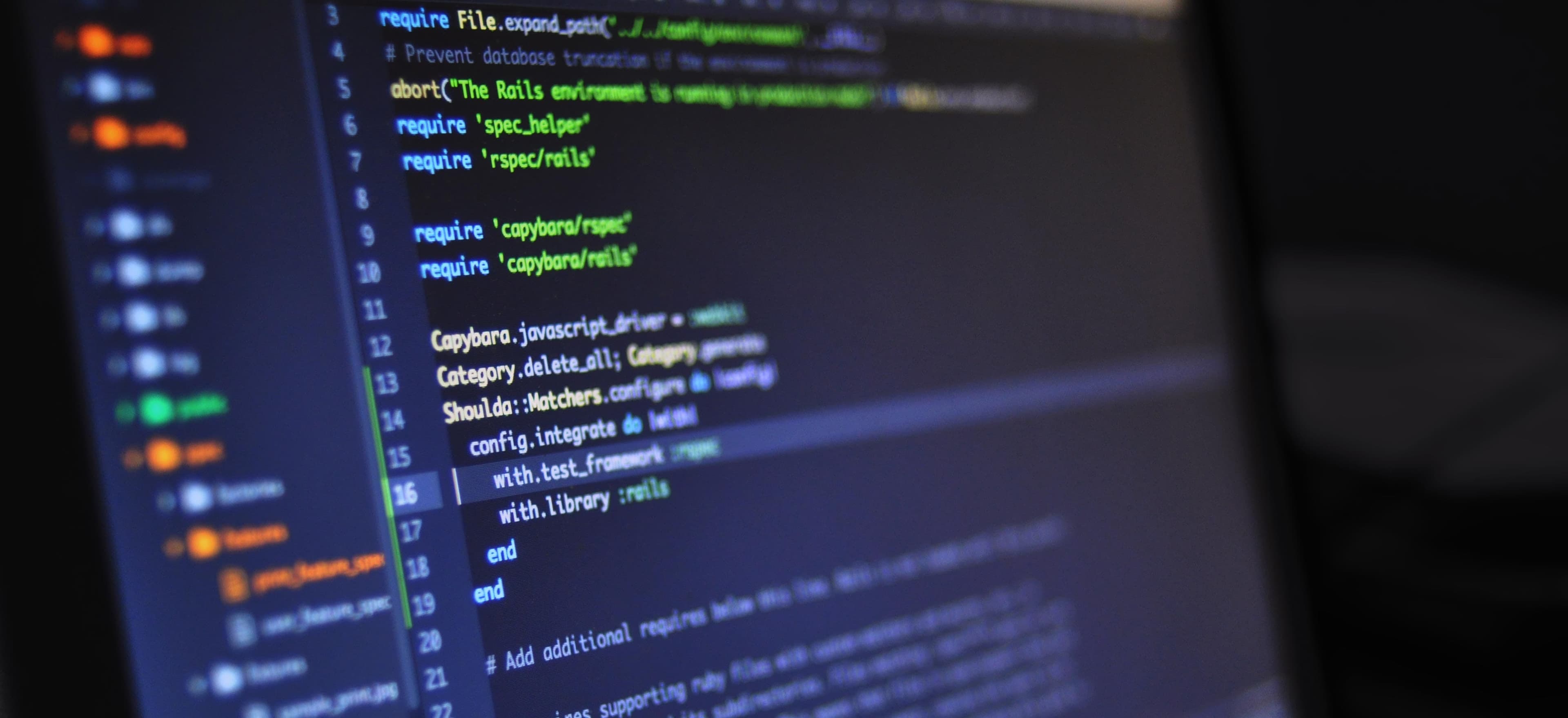
- Published on
Building Java Code Models: Overcoming Source and JAR File Challenges
Java is a powerful and widely-used programming language that allows for the development of robust and efficient applications. However, when working with Java codebases, developers often encounter challenges related to handling source code and JAR files. In this article, we will explore these challenges and discuss strategies for overcoming them when building Java code models.
Understanding the Challenges
Source Code vs. JAR Files
When analyzing Java code, developers may have to work with both the source code and compiled JAR files. Source code provides insights into the implementation details and allows for easy navigation and search. On the other hand, JAR files contain compiled class files and are essential for building and executing applications.
Lack of Documentation
Many Java codebases suffer from a lack of adequate documentation. This makes it difficult for developers to understand the code and its dependencies, leading to challenges in building accurate code models.
Overcoming Source Code and JAR File Challenges
Using Java Parser Libraries
Java parser libraries such as JavaParser and Eclipse JDT provide powerful tools for parsing and analyzing Java source code. These libraries enable developers to programmatically traverse the abstract syntax tree (AST) of Java code, extract relevant information, and build code models.
// Example of using JavaParser to parse a Java source file
CompilationUnit cu = JavaParser.parse(new File("Example.java"));
Generating JAR File Indexes
To overcome the challenges associated with JAR files, developers can generate indexes of classes and their dependencies using tools like JarAnalyzer and JarIndexer. These tools can create searchable indexes of classes within JAR files, making it easier to extract information about dependencies and build comprehensive code models.
Leveraging Dependency Management Tools
Dependency management tools such as Maven and Gradle play a crucial role in Java development. By utilizing these tools, developers can automatically resolve and download dependencies, making it easier to access the source code of libraries and build accurate code models.
<!-- Example of defining a dependency in Maven -->
<dependency>
<groupId>com.example</groupId>
<artifactId>library</artifactId>
<version>1.0.0</version>
</dependency>
Integrating with IDE APIs
Integrated Development Environments (IDEs) such as IntelliJ IDEA and Eclipse provide robust APIs for interacting with Java code. By leveraging IDE APIs, developers can access features like code navigation, code completion, and refactoring, which can significantly aid in building comprehensive code models.
Best Practices for Building Java Code Models
Documenting Code
Maintaining comprehensive and up-to-date documentation is crucial for building accurate code models. By documenting the codebase using tools like Javadoc and Doxygen, developers can ensure that code models reflect the latest changes and include relevant context.
Version Control Integration
Integrating code model building processes with version control systems like Git enables developers to track changes and manage code versions effectively. This ensures that code models are synchronized with the latest codebase revisions.
Continuous Integration
Incorporating code model building into the continuous integration (CI) pipeline ensures that code models are updated regularly and reflect the current state of the codebase. Tools like Jenkins and Travis CI can be leveraged to automate code model generation as part of the CI/CD process.
Wrapping Up
Building Java code models involves overcoming challenges related to source code and JAR files. By utilizing Java parser libraries, generating JAR file indexes, leveraging dependency management tools, and integrating with IDE APIs, developers can effectively build comprehensive code models. Additionally, following best practices such as documenting code, integrating with version control, and incorporating code model building into CI processes, ensures that code models remain accurate and up-to-date.
With the strategies and best practices outlined in this article, developers can tackle the complexities of building Java code models and gain a deeper understanding of Java codebases.
For further reading on Java code analysis and modeling, check out the official documentation for JavaParser and Maven.
Remember, the key to successful code modeling lies in understanding the codebase, utilizing the right tools, and following best practices for maintaining accuracy and relevancy. Happy coding!