Optimizing Continuous Testing in DevOps: Overcoming Test Environment Bottlenecks
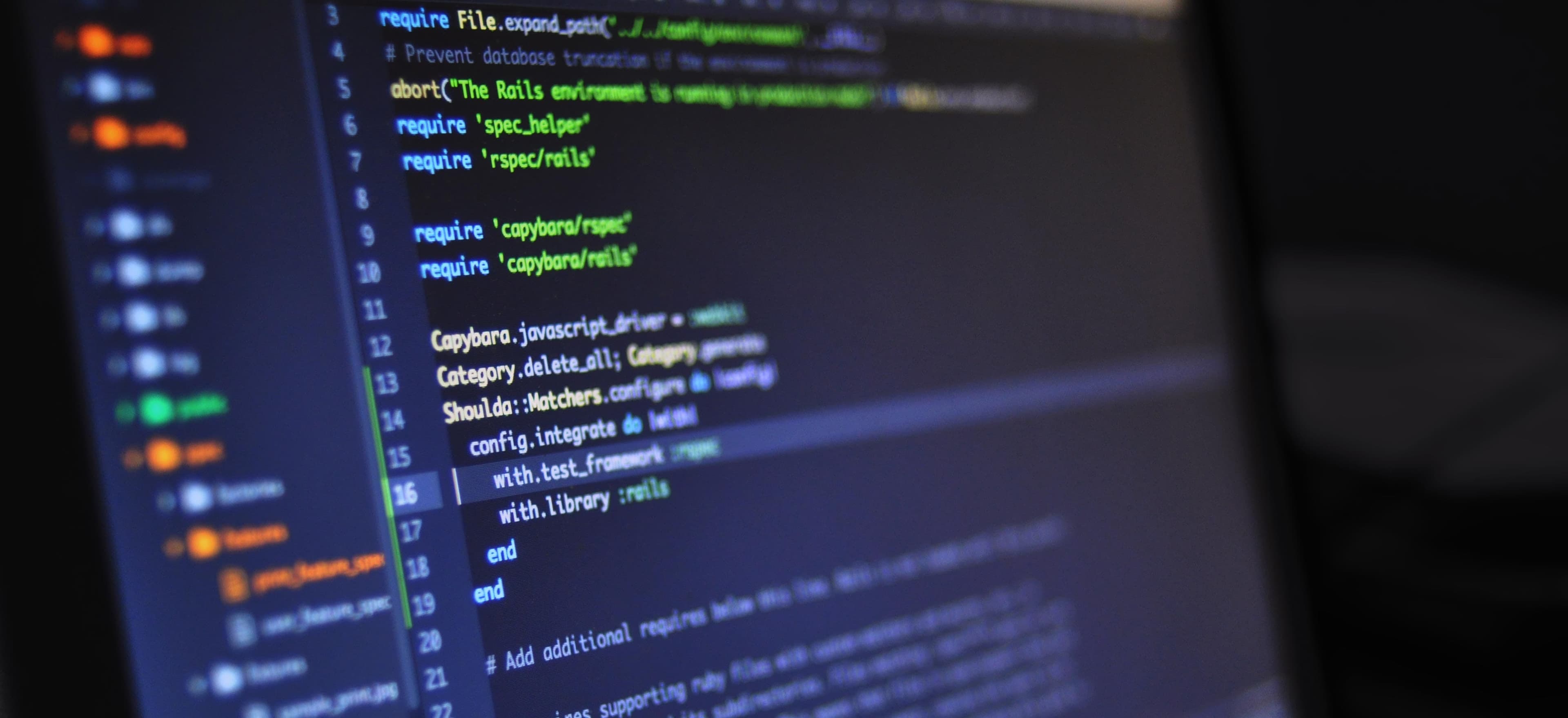
- Published on
Optimizing Continuous Testing in DevOps: Overcoming Test Environment Bottlenecks
Software development in today's fast-paced world demands rapid and continuous testing to ensure high-quality, reliable software. DevOps has gained prominence as a methodology for accelerating software development and delivery, emphasizing collaboration, automation, and integration between software development and IT operations teams. Continuous Testing is a fundamental practice in DevOps, striving to provide immediate feedback on the business risks associated with a software release candidate.
However, implementing Continuous Testing in a DevOps environment often introduces challenges related to test environment bottlenecks. These bottlenecks can significantly hamper the speed and efficiency of the testing process, leading to delays in delivering software. In this article, we will explore strategies for overcoming test environment bottlenecks and optimizing Continuous Testing in a DevOps ecosystem, with a focus on Java-based applications.
Understanding Test Environment Bottlenecks
Test environment bottlenecks arise when there are limitations or inefficiencies in the availability, setup, and maintenance of the test environments required for executing automated tests. These bottlenecks can manifest in various forms, such as:
- Limited Availability: Insufficient availability of test environments due to resource constraints or contention among development, testing, and staging teams.
- Slow Provisioning: Time-consuming process of setting up and configuring test environments, leading to delays in executing tests.
- Data Dependencies: Complex data dependencies that hinder the creation of isolated and deterministic test environments.
- Infrastructure Configurations: Challenges in replicating production-like infrastructure configurations for comprehensive testing.
- Environment Stability: Unstable test environments that introduce unpredictability and flakiness in test results.
Addressing these bottlenecks is crucial for achieving the rapid feedback and quality assurance objectives of Continuous Testing within a DevOps workflow.
Strategies for Overcoming Test Environment Bottlenecks
Infrastructure as Code (IaC)
One of the foundational strategies for overcoming test environment bottlenecks is the adoption of Infrastructure as Code (IaC). IaC involves managing and provisioning computing infrastructure through machine-readable definition files, rather than physical hardware configuration or interactive configuration tools. In the context of Java applications, tools like Terraform and CloudFormation enable the automated provisioning of test environments, offering consistency and reproducibility.
// Example Terraform configuration for provisioning test environment on AWS
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
In the above Terraform snippet, we define an AWS EC2 instance as code. By codifying infrastructure, teams can efficiently manage and replicate test environments while minimizing manual errors and accelerating the setup process.
Containerization with Docker
Containerization, particularly with Docker, presents a compelling solution to test environment bottlenecks. Docker allows packaging an application and its dependencies into a standardized unit for software development, ensuring consistency across different environments. Java applications can be seamlessly containerized, enabling the creation of lightweight, isolated test environments that can be spun up quickly.
// Dockerfile for containerizing a Java application
FROM openjdk:11-jre-slim
COPY ./target/app.jar /app/
CMD ["java", "-jar", "/app/app.jar"]
The Dockerfile above demonstrates a simple configuration to package a Java application into a Docker container. By leveraging Docker Compose or orchestration tools like Kubernetes, teams can orchestrate complex test environments with ease, addressing the challenges of environment provisioning and configuration.
Test Data Management
Effective test data management is pivotal in mitigating data dependencies that impede test environment setup. Tools such as Test Data Management (TDM) platforms or libraries like Mockito can aid in creating synthetic or masked test data, ensuring the independence and repeatability of automated tests.
// Using Mockito to create test data for a Java unit test
@Test
public void testCalculateTotalRevenue() {
List<Order> mockOrders = Arrays.asList(
new Order(1, "Product A", 100.00),
new Order(2, "Product B", 150.00)
);
when(orderService.getOrders()).thenReturn(mockOrders);
// Assert the calculated total revenue
assertEquals(250.00, revenueCalculator.calculateTotalRevenue());
}
In the above JUnit test case, Mockito is utilized to mock the Order data, eliminating the reliance on external data sources and ensuring consistent test outcomes.
Parallel Test Execution
Parallelizing test execution across distributed environments can significantly mitigate the impact of limited availability and slow provisioning of test environments. Test frameworks like TestNG or JUnit 5 offer built-in support for parallel test execution, allowing Java tests to run concurrently across multiple nodes or containers.
// TestNG configuration for parallel test execution
<suite name="TestSuite" parallel="tests" thread-count="5">
<test name="RegressionTests">
<classes>
<class name="com.example.RegressionTest1" />
<class name="com.example.RegressionTest2" />
<!-- More test classes -->
</classes>
</test>
</suite>
By harnessing the power of parallelism, teams can expedite test cycles and maximize resource utilization, effectively overcoming the constraints posed by limited test environment availability.
Environment Monitoring and Maintenance
Continuous monitoring and proactive maintenance of test environments are essential for ensuring their stability and availability. Tools such as Prometheus and Grafana can be employed to monitor the health and performance of test environments, enabling teams to identify and address issues before they impact the testing process.
// Using Micrometer to instrument Java application for monitoring
import io.micrometer.core.instrument.Counter;
import io.micrometer.core.instrument.MeterRegistry;
public class OrderService {
private final Counter ordersCounter;
public OrderService(MeterRegistry registry) {
this.ordersCounter = Counter.builder("orders.processed")
.description("Total processed orders")
.register(registry);
}
public void processOrder(Order order) {
// Process the order
// Increment the counter
ordersCounter.increment();
}
}
In the above Java class, Micrometer is utilized to instrument the OrderService, capturing metrics such as the total processed orders. By integrating such monitoring capabilities, teams can ensure the reliability and performance of test environments, preempting potential bottlenecks.
Bringing It All Together
Continuous Testing in a DevOps environment necessitates a concerted effort to overcome test environment bottlenecks, enabling the seamless and rapid execution of automated tests. Through the adoption of Infrastructure as Code, containerization, effective test data management, parallel test execution, and proactive environment monitoring, organizations can optimize their Continuous Testing practices and accelerate the delivery of high-quality software.
By embracing these strategies and leveraging Java's flexibility and robust ecosystem, teams can cultivate a resilient testing infrastructure that aligns with the principles of DevOps, fostering a culture of collaboration, automation, and continuous improvement.
In conclusion, the journey to optimizing Continuous Testing in DevOps is not without its challenges, but with the right strategies and technologies at hand, organizations can surmount test environment bottlenecks and embark on a path towards streamlined, efficient, and reliable testing processes.
Remember, efficient Continuous Testing is not just a goal; it is a journey of continuous refinement and optimization.
For further reading and practical insights on DevOps and Java testing:
- The Twelve-Factor App - A methodology for building modern, scalable, and maintainable software-as-a-service applications.
- JUnit 5 User Guide - Comprehensive documentation for leveraging the power of JUnit 5 for Java testing.
- Docker Documentation - Official Docker documentation for understanding and mastering containerization with Docker.
Thank you for reading, and happy testing!