The Diamond Problem in Java
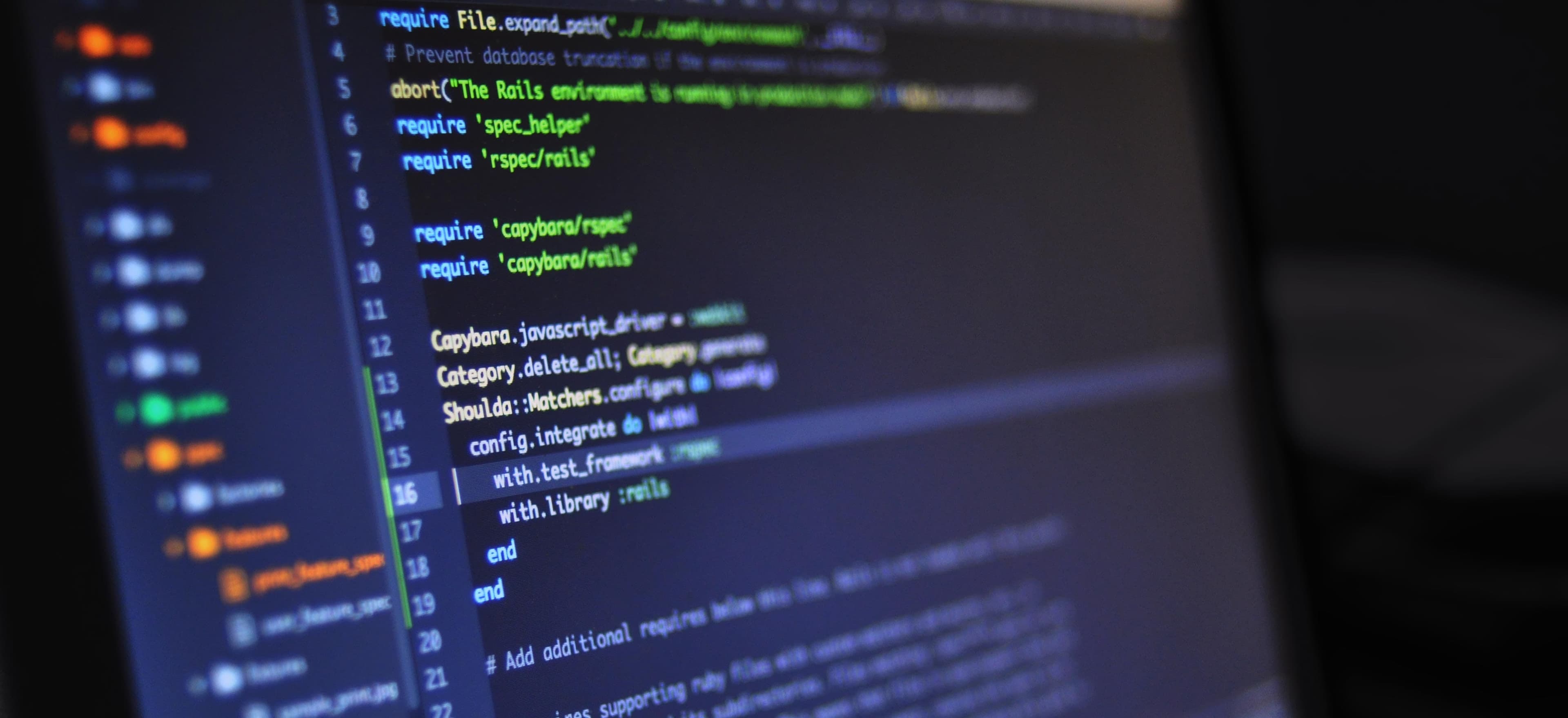
- Published on
Understanding the Diamond Problem in Java
In Java, the diamond problem refers to the complication that arises when a class inherits from two classes that have a common ancestor. This situation can lead to ambiguity and conflicts in the inheritance hierarchy. Let’s delve deeper into the diamond problem, explore its causes, and discuss how to mitigate it in Java.
The Diamond Problem Explained
Consider a scenario where ClassA and ClassB both inherit from ClassC. Now, if a new class, ClassD, inherits from both ClassA and ClassB, the diamond problem arises. This is because ClassD is now inheriting from ClassC through multiple paths, which can lead to ambiguity and conflicts during method resolution.
Example of the Diamond Problem
class ClassC {
public void doSomething() {
System.out.println("I'm from ClassC");
}
}
class ClassA extends ClassC {
}
class ClassB extends ClassC {
}
class ClassD extends ClassA, ClassB {
}
In this example, ClassD is inheriting from both ClassA and ClassB, both of which ultimately inherit from ClassC. When we create an instance of ClassD and call the doSomething()
method, the ambiguity arises as to which doSomething()
method should be invoked - the one from ClassA or from ClassB.
Resolving the Diamond Problem with Interfaces
In Java, the diamond problem can be resolved using interfaces. By making ClassA and ClassB interfaces instead of classes, we can implement them in ClassD, thus avoiding the diamond problem altogether.
interface InterfaceA {
void doSomething();
}
interface InterfaceB {
void doSomething();
}
class ClassC implements InterfaceA, InterfaceB {
public void doSomething() {
System.out.println("I'm from ClassC");
}
}
class ClassD implements InterfaceA, InterfaceB {
public void doSomething() {
// Resolve the ambiguity here
}
}
By implementing InterfaceA
and InterfaceB
in ClassD, we specifically define how the doSomething()
method should be implemented, effectively avoiding the diamond problem.
Using Default Methods in Interfaces
With the introduction of Java 8, interfaces can have concrete methods through default methods. This feature can also be leveraged to mitigate the diamond problem. By providing default method implementations in interfaces, we can ensure that the implementing class (in this case, ClassD) resolves the conflict explicitly.
interface InterfaceA {
default void doSomething() {
System.out.println("I'm from InterfaceA");
}
}
interface InterfaceB {
default void doSomething() {
System.out.println("I'm from InterfaceB");
}
}
class ClassD implements InterfaceA, InterfaceB {
// Explicitly resolve the conflict by overriding the default method
public void doSomething() {
InterfaceA.super.doSomething(); // Explicitly call the desired implementation
}
}
In this example, both InterfaceA
and InterfaceB
have a default implementation for the doSomething()
method. By implementing ClassD from these interfaces and explicitly overriding the doSomething()
method, we can resolve the conflict and specify which implementation should be used.
The Last Word
The diamond problem in Java inheritance can lead to ambiguity and conflicts, but it can be effectively resolved using interfaces and default methods. By leveraging interfaces and their default method features, we can avoid the diamond problem and ensure a clear and unambiguous inheritance hierarchy.
Understanding the diamond problem and its resolutions is crucial for Java developers to design robust and maintainable codebases.
In summary, the diamond problem can be mitigated in Java through:
- Implementing classes from interfaces instead of multiple classes
- Using default methods in interfaces to provide a clear resolution for method conflicts
By incorporating these techniques, Java developers can navigate around the diamond problem and ensure a smooth inheritance hierarchy.
For further understanding of the diamond problem and Java inheritance, refer to the official Java documentation.
With a clear grasp of the diamond problem and its solutions, Java developers can architect inheritance structures that are free from ambiguity and conflicts, contributing to more maintainable and scalable codebases.