Maven and Ivy Integration Best Practices
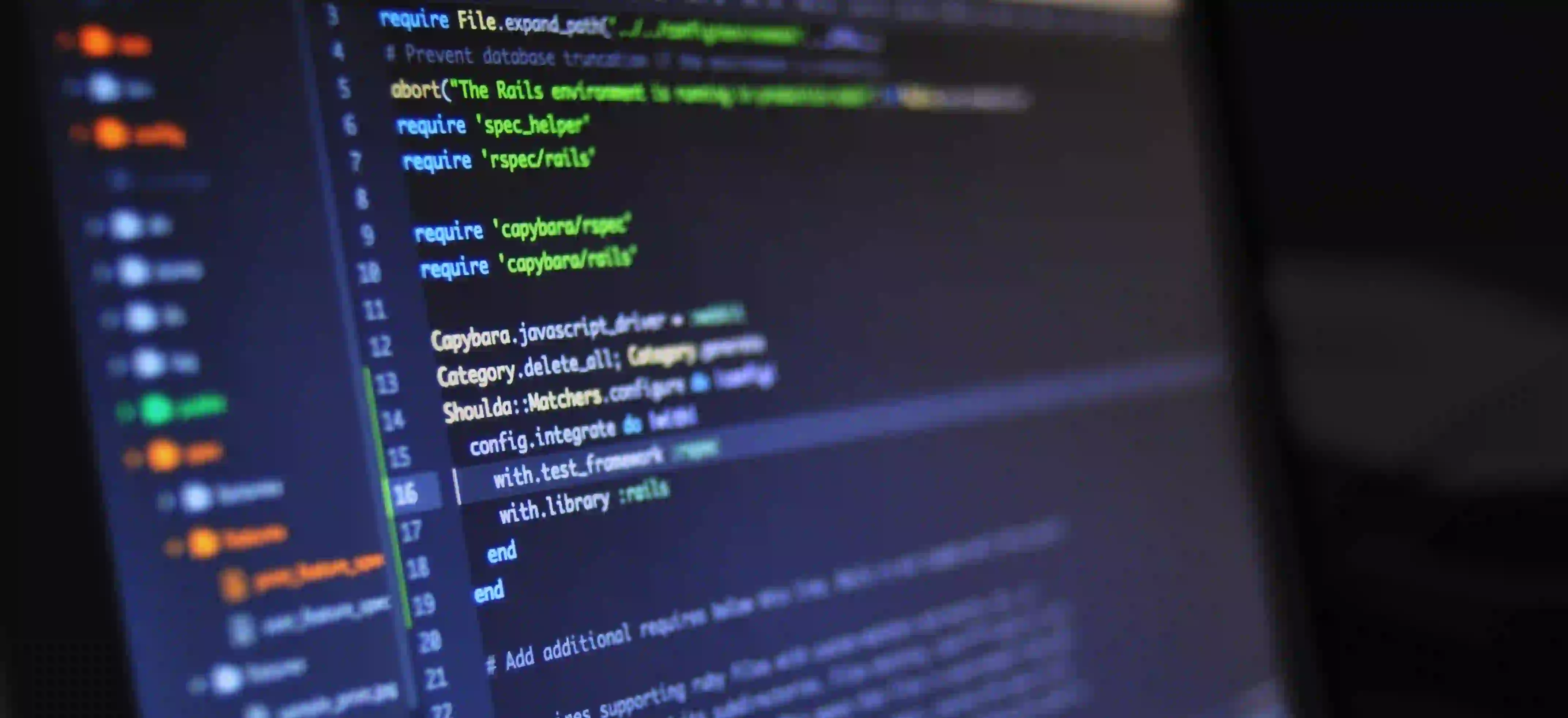
Integrating Maven and Ivy for Efficient Java Dependency Management
Managing dependencies is a crucial aspect of Java development, and build automation tools like Maven and Ivy play a vital role in simplifying this process. In this post, we'll explore the best practices for integrating Maven and Ivy to manage dependencies effectively in Java projects.
Why Integrate Maven and Ivy?
Maven is a popular build automation tool that provides a standard way to manage project dependencies and build processes. It uses a Project Object Model (POM) to manage projects and their dependencies. On the other hand, Ivy is a powerful dependency management tool that can be integrated with Apache Ant to manage project dependencies.
Integrating Maven and Ivy brings the best of both worlds – the robust build management of Maven and the flexible dependency resolution of Ivy. This combination allows for seamless dependency management and build automation in Java projects.
Setting Up Maven and Ivy Integration
Step 1: Configure Maven
In your Maven project, configure the pom.xml
file to include the Ivy plugin. This allows Maven to work in conjunction with Ivy for dependency resolution.
<plugin>
<groupId>org.apache.ivy</groupId>
<artifactId>ivy-maven-plugin</artifactId>
<version>2.4.0</version>
<executions>
<execution>
<phase>generate-resources</phase>
<goals>
<goal>init</goal>
<goal>resolve</goal>
</goals>
</execution>
</executions>
</plugin>
In this configuration, the ivy-maven-plugin
is included, and two goals, init
and resolve
, are specified to be executed during the generate-resources
phase of the Maven build lifecycle.
Step 2: Configure Ivy
Next, create an ivy.xml
file in the root of your project to define the dependencies managed by Ivy.
<ivy-module version="2.0">
<info organisation="com.example" module="my-project"/>
<dependencies>
<dependency org="commons-lang" name="commons-lang" rev="2.6"/>
<!-- Additional dependencies -->
</dependencies>
</ivy-module>
Here, the ivy.xml
file defines the project's dependencies, including the organization, module, and specific artifact versions required.
Step 3: Integrate Ivy with Ant
To make Ivy work alongside Maven, an Ant build file (build.xml
) should be created or updated to include Ivy tasks for dependency resolution.
<project name="my-project" default="resolve" basedir="." xmlns:ivy="antlib:org.apache.ivy.ant">
<property name="ivy.dir" value="${user.home}/.ivy2" />
<property name="ivy.settings.file" value="${ivy.dir}/settings.xml" />
<target name="resolve">
<ivy:resolve file="ivy.xml"/>
<ivy:retrieve pattern="lib/[conf]/[artifact]-[revision](-[classifier]).[ext]"/>
</target>
</project>
In this Ant build file, the ivy:resolve
task is used to resolve the project dependencies as specified in the ivy.xml
file, and the ivy:retrieve
task is used to retrieve the resolved artifacts into the project's designated library directory.
Best Practices for Maven and Ivy Integration
1. Leverage Maven's Project Structure
When integrating Maven and Ivy, it's beneficial to follow Maven's standard project structure. This allows for seamless integration without the need for additional configuration.
2. Utilize Maven Profiles
Maven profiles can be used to centralize and manage different sets of project configurations. Utilize Maven profiles to define Ivy-related configurations and dependencies, ensuring a clean and organized approach to integration.
3. Define Exclusions Appropriately
In scenarios where conflicts arise between dependencies managed by Maven and Ivy, define exclusions in the POM file to avoid version clashes and ensure that the desired version is used.
4. Automate Ivy Resolution in Continuous Integration
Integrate Ivy dependency resolution into your continuous integration (CI) process to ensure that all dependencies are resolved consistently across different build environments.
5. Version Alignment
Ensure that the versions of dependencies managed by Ivy align with those specified in the POM file to maintain consistency and avoid conflicts during the build process.
Bringing It All Together
Integrating Maven and Ivy provides a powerful solution for managing dependencies in Java projects. By following best practices and leveraging the strengths of both tools, developers can ensure efficient and streamlined dependency management. This integration allows for flexibility, control over dependency resolution, and seamless build automation in Java projects.
Incorporating Maven and Ivy integration best practices not only simplifies the management of project dependencies but also contributes to the overall efficiency and reliability of the build process.
In conclusion, combining Maven and Ivy's capabilities leads to a robust dependency management system that supports the development of scalable and reliable Java applications.
Integrate Maven and Ivy today to streamline your Java dependency management process and experience the benefits of this powerful combination!