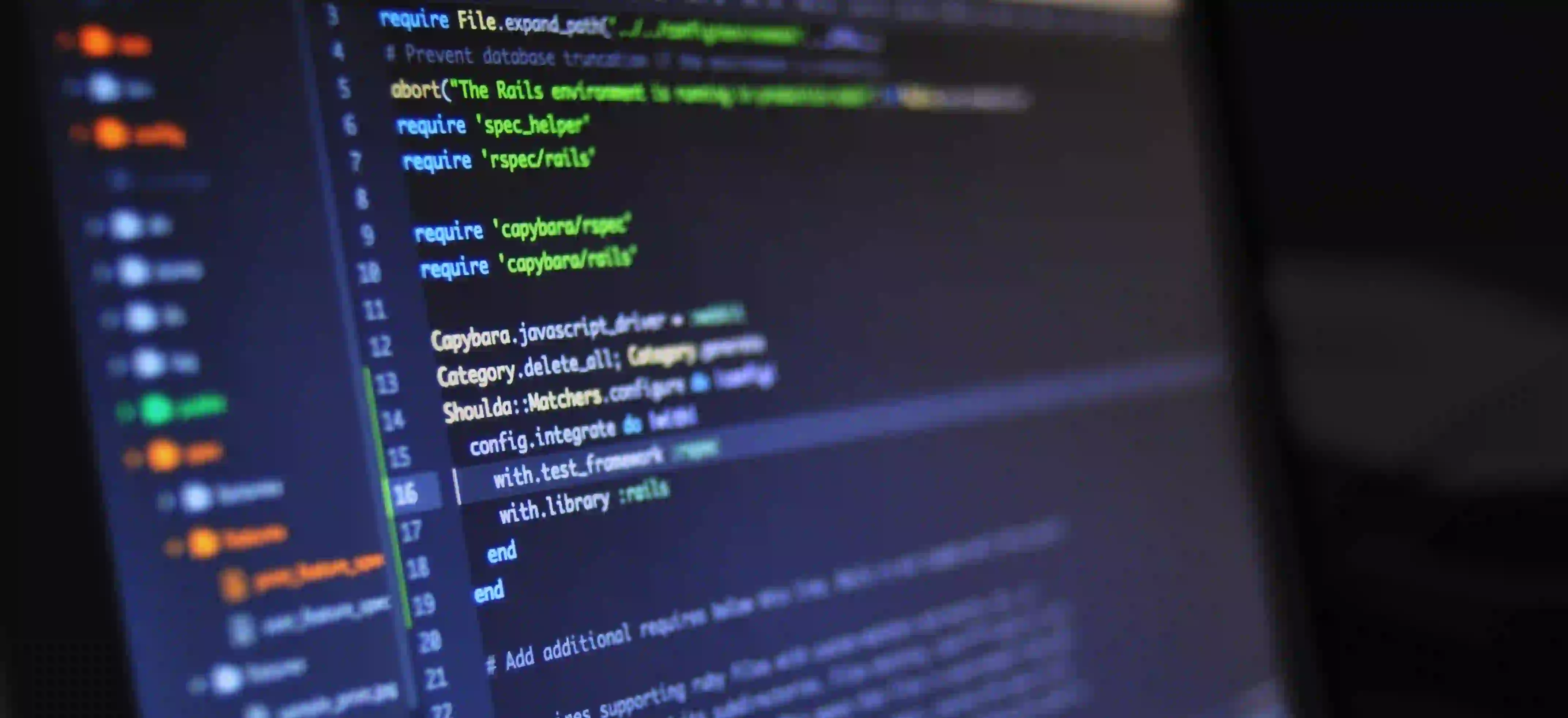
Securing Containers: Best Practices for Enhanced Protection
In recent years, containerization has become increasingly popular due to its scalability, flexibility, and portability. However, as with any technology, securing containers is of utmost importance to prevent potential vulnerabilities and attacks. In this article, we will explore best practices for enhancing the security of your Java containers to ensure the protection of your applications and data.
Understanding Container Security Challenges
Containers, while offering several advantages, also bring about unique security challenges. Understanding these challenges is crucial in implementing effective security measures.
1. Image Security
Container images serve as the foundation for running containers. However, using untrusted or outdated images can introduce security risks. It's essential to regularly update and scan container images for vulnerabilities using tools like Clair or Docker Security Scanning.
2. Orchestration Security
Container orchestration platforms like Kubernetes play a vital role in managing containerized applications. However, misconfigurations in these platforms can lead to severe security breaches. Employing strict access controls and regularly auditing configurations is critical.
3. Runtime Security
Containers in runtime pose potential risks such as privilege escalations and attacks on host systems. Employing mechanisms like AppArmor and SELinux can help mitigate these threats by enforcing strict access controls and isolation.
Best Practices for Java Container Security
Now let's delve into specific best practices for securing Java containers, focusing on application-level security, image security, and runtime security.
Application-Level Security
1. Implement Role-Based Access Control (RBAC)
Utilize RBAC mechanisms provided by frameworks like Spring Security to control user access and permissions within your Java applications. By defining granular roles and permissions, you can enforce the principle of least privilege, reducing the risk of unauthorized actions.
// Example of defining a custom role in Spring Security
@EnableGlobalMethodSecurity(securedEnabled = true)
public class MethodSecurityConfig extends GlobalMethodSecurityConfiguration {
@Override
protected AccessDecisionManager accessDecisionManager() {
RoleHierarchyImpl roleHierarchy = new RoleHierarchyImpl();
roleHierarchy.setHierarchy("ROLE_ADMIN > ROLE_USER");
return new AffirmativeBased(Collections.singletonList(new RoleVoter()),);
}
}
2. Input Validation and Sanitization
Ensure that your Java applications implement thorough input validation and sanitization to prevent common vulnerabilities like SQL injection and Cross-Site Scripting (XSS). Libraries such as Hibernate Validator can aid in validating input data and enforcing constraints.
// Example of input validation using Hibernate Validator
public class User {
@NotNull
@Size(min = 5, max = 20)
private String username;
@Email
private String email;
// Getters and setters
}
Image Security
1. Base Image Hardening
Avoid using unnecessarily large or insecure base images for building Java containers. Instead, leverage minimal and hardened base images such as AdoptOpenJDK or Alpine Linux to reduce the attack surface and potential vulnerabilities.
2. Image Scanning and Vulnerability Management
Incorporate container image scanning tools into your CI/CD pipeline to automatically detect and address vulnerabilities in your Java container images. Tools like Trivy and Anchore Engine can provide insights into vulnerable dependencies and insecure configurations.
Runtime Security
1. Least Privilege Principle
Apply the principle of least privilege by running Java containers with minimal necessary permissions. Avoid running processes as root within the containers and utilize user namespaces to map container users to non-privileged users on the host.
# Example of running a Java application as a non-root user in a Dockerfile
FROM adoptopenjdk:11-jre-hotspot
RUN addgroup -S myapp && adduser -S myapp -G myapp
USER myapp
COPY target/myapp.jar /app/myapp.jar
CMD ["java", "-jar", "/app/myapp.jar"]
2. Container Network Security
Implement network policies to restrict container-to-container communication and specify allowed ingress and egress traffic. Tools like Calico and Cilium can assist in enforcing network segmentation and policies within your containerized Java applications.
Bringing It All Together
Securing Java containers involves a multi-faceted approach, encompassing application-level security, image security, and runtime security. By adhering to best practices such as implementing RBAC, utilizing hardened base images, and enforcing network segmentation, you can strengthen the security posture of your containerized Java applications. Continuously monitoring and updating security measures is essential to adapt to evolving threats and maintain robust container security.
Incorporating these best practices will not only fortify the security of your Java containers but also contribute to the overall resilience of your containerized infrastructure. By embracing a proactive stance towards container security, you can confidently leverage the benefits of containerization while safeguarding your applications and data against potential threats.
To further explore container security best practices, refer to the official Kubernetes documentation and Docker security best practices. These valuable resources provide in-depth insights and recommendations for enhancing the security of containerized environments.