The Pitfalls of Overusing the 'var' Keyword in Java 10
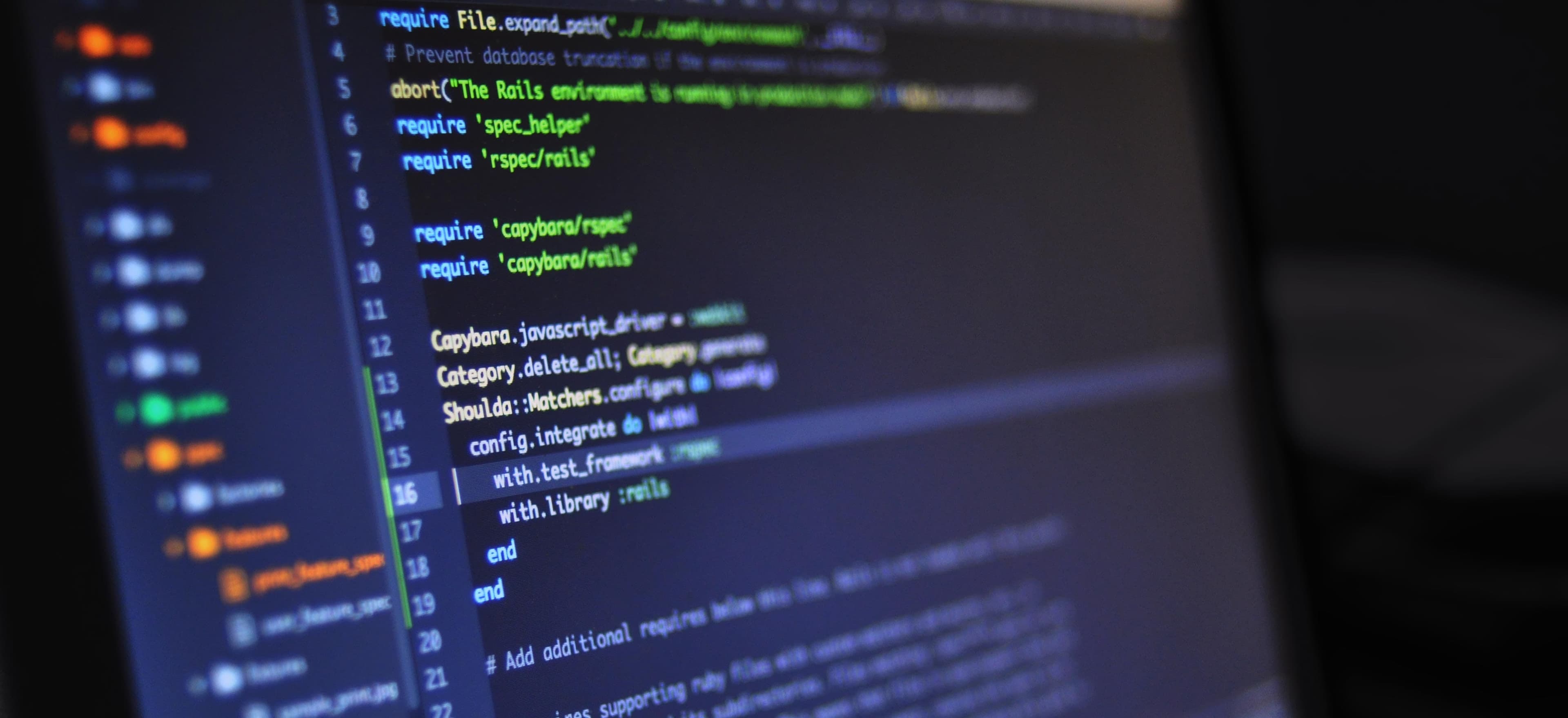
- Published on
The Pitfalls of Overusing the 'var' Keyword in Java 10
In September 2018, Java 10 introduced the 'var' keyword, which allows developers to declare local variables with implicit type inference. This new feature aimed to enhance code readability and reduce boilerplate, especially when dealing with complex generic types.
While the 'var' keyword certainly has its benefits, its overuse can lead to a number of pitfalls. In this article, we will explore the advantages and disadvantages of using 'var' excessively in Java 10 and provide best practices for its usage.
Benefits of the 'var' Keyword
The 'var' keyword in Java 10 brings several advantages to the table:
- Reduced Boilerplate Code: Replace verbose type declarations with 'var' to make the code more concise and readable.
- Enhanced Code Readability: When used judiciously, 'var' can improve the clarity of code by focusing on the intent rather than the type details.
- Handling Complex Types: 'var' simplifies the declaration of complex generic types, such as those involving streams and lambdas.
Pitfalls of Overusing 'var'
While 'var' can greatly improve the clarity and conciseness of Java code, its overuse can lead to several pitfalls:
- Reduced Readability: Excessive use of 'var' can make the code less readable, especially for developers unfamiliar with the codebase. Explicitly declaring types can provide clear insights into the variable's purpose.
- Loss of Intention: Omitting the explicit type declaration with 'var' may obscure the original intention of the variable, making the code harder to understand and maintain.
- Compiler Dependency: Relying too heavily on 'var' ties the code's readability to the compiler, potentially causing confusion when transitioning to older Java versions or other developers using different setups.
Best Practices for Using 'var'
To maximize the benefits of 'var' while mitigating its drawbacks, here are some best practices to follow:
-
Use 'var' for Long Type Declarations: Prefer using 'var' for cases where the type is lengthy or verbose, such as complex generic types or deeply nested structures. This can significantly streamline the code without sacrificing readability.
// Before: Verbose type declaration Map<String, List<Integer>> myMap = new HashMap<>(); // After: Utilizing 'var' for conciseness var myMap = new HashMap<String, List<Integer>>();
It's crucial to note that utilizing 'var' for shorter and straightforward types may obscure the variable's intent, leading to reduced readability.
-
Retain Explicit Type for Clarity: Reserve the use of 'var' for cases where the variable's type is evident from the right-hand side of the declaration. For instance, when the type is explicitly mentioned in the instantiation, using 'var' can streamline the code without affecting its clarity.
// Explicit type in instantiation List<String> names = new ArrayList<>(); // Utilizing 'var' without sacrificing readability var names = new ArrayList<String>();
In cases where the type is not immediately evident from the right-hand side or where the variable's intent is crucial, it's preferable to retain the explicit type declaration for clarity and maintainability.
-
Maintain Consistency within the Codebase: Establish clear conventions within the codebase regarding the usage of 'var'. Consistency enhances readability and reduces cognitive overhead when navigating the code.
Adhering to consistent guidelines ensures that all developers working in the codebase understand when and where to use 'var', thereby preventing confusion and maintaining a unified style.
-
Consider Code Reviews and Feedback: Incorporate feedback from code reviews to evaluate the appropriate usage of 'var'. Collaborating with team members can help establish best practices and strike a balance between streamlined code and clear intent.
By soliciting feedback and engaging in discussions about the usage of 'var', developers can ensure that the codebase maintains a healthy balance between conciseness and readability.
The Bottom Line
The introduction of the 'var' keyword in Java 10 brought a significant enhancement to the language, enabling developers to write more concise and readable code. However, the overuse of 'var' can lead to reduced code clarity and maintenance challenges. By adhering to best practices and maintaining a balance between conciseness and explicitness, developers can leverage the benefits of 'var' without succumbing to its pitfalls.
Carefully evaluating the intent and readability of code snippets and considering feedback from peers can significantly contribute to a codebase that is not only concise but also comprehensible and maintainable.
By embracing a thoughtful and balanced approach to the usage of 'var', developers can harness its strengths while mitigating its potential pitfalls, thereby enhancing the overall quality and maintainability of Java codebases.
Checkout our other articles