Optimizing Microservices Load Balancing with Zookeeper Curator
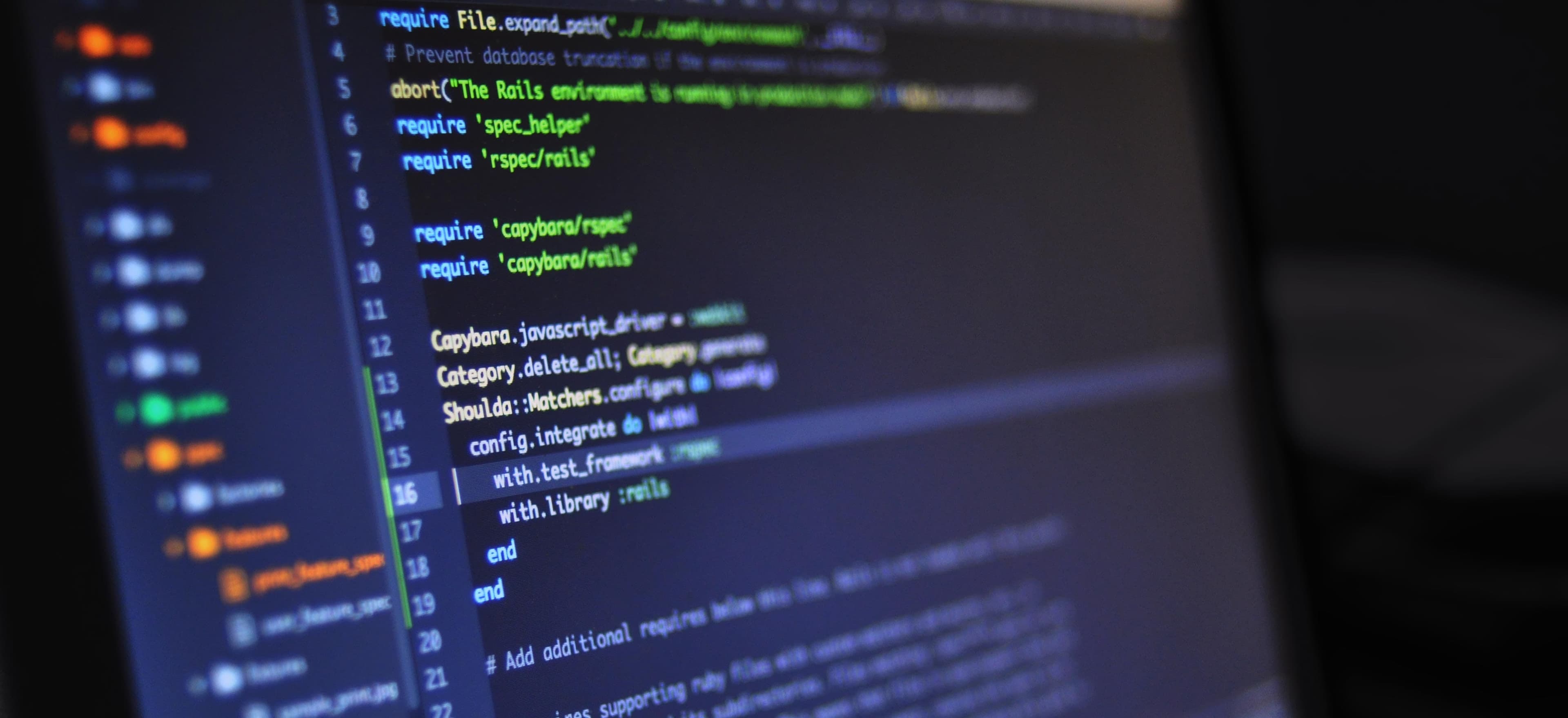
- Published on
Optimizing Microservices Load Balancing with Zookeeper Curator
In modern microservices architectures, load balancing plays a crucial role in ensuring high availability and efficient distribution of incoming traffic across instances of microservices. Zookeeper Curator is a powerful framework that facilitates the development of distributed systems, including load balancing within a microservices environment. In this article, we will explore how to use Zookeeper Curator to optimize microservices load balancing in a Java-based application.
Understanding Microservices Load Balancing
Before delving into the technical aspects of Zookeeper Curator, it's essential to understand the principles behind microservices load balancing. In a microservices architecture, multiple instances of a service are deployed to handle incoming requests. Load balancing is the process of distributing these requests across the available instances, ensuring that the workload is evenly distributed and that no single instance is overwhelmed.
Load balancers can employ various strategies to distribute traffic, including round-robin, least connections, IP hash, and more. However, in a dynamic microservices environment where instances can scale up or down based on demand, traditional load balancing approaches may fall short. This is where Zookeeper Curator comes into play.
Introducing Zookeeper Curator
Zookeeper Curator is a robust Java library that provides high-level abstractions and utilities for interacting with Apache Zookeeper, a widely used coordination service for distributed applications. Within the context of microservices, Zookeeper Curator can be leveraged to maintain dynamic lists of available service instances and perform efficient load balancing.
In addition to its core features for working with Zookeeper, Zookeeper Curator includes recipes for common distributed system patterns, such as leader election, distributed locks, and service discovery. These recipes are instrumental in implementing reliable and scalable microservices architectures.
Implementing Dynamic Load Balancing with Zookeeper Curator
To demonstrate the use of Zookeeper Curator for dynamic load balancing in a Java-based microservices application, let's consider a simplified scenario where we have a service that needs to balance incoming requests across multiple instances.
Setting up Zookeeper Connection
The first step is to establish a connection to the Zookeeper ensemble. Zookeeper Curator provides a CuratorFramework
for interacting with Zookeeper. It handles the connection management and provides a fluent API for performing operations such as creating nodes, watching for changes, and more.
CuratorFramework client = CuratorFrameworkFactory.newClient("zookeeper-host:port", new RetryForever(1000));
client.start();
In this snippet, we initialize a CuratorFramework
instance by providing the connection string to the Zookeeper ensemble and a retry policy.
Registering Service Instances
Next, we need to register the instances of our service with Zookeeper. When a new instance comes online or a running instance goes offline, it should update its status in Zookeeper, allowing the load balancer to dynamically adjust.
String servicePath = "/services/my-service";
String instanceId = "instance-1";
String instancePath = servicePath + "/" + instanceId;
client.create().creatingParentsIfNeeded().withMode(CreateMode.EPHEMERAL).forPath(instancePath, "127.0.0.1:9000".getBytes());
In this example, we create an ephemeral node in Zookeeper to represent the instance of our service. The ephemeral node will automatically be removed if the instance goes offline ungracefully.
Watching for Service Changes
To perform dynamic load balancing, we need to watch for changes in the list of available service instances. Zookeeper Curator provides the PathChildrenCache
class for efficiently watching a ZNode and its children for changes.
PathChildrenCache cache = new PathChildrenCache(client, servicePath, true);
cache.getListenable().addListener((client, event) -> {
// Handle changes to the list of service instances
});
cache.start(PathChildrenCache.StartMode.BUILD_INITIAL_CACHE);
By using the PathChildrenCache
, we can keep track of changes to the list of service instances in real-time and adjust the load balancing accordingly.
Load Balancing Algorithm
With the ability to monitor changes in the list of service instances, we can now implement a load balancing algorithm that distributes incoming requests among the available instances. Depending on the specific requirements, various load balancing strategies can be applied, such as round-robin, weighted distribution, or least connection.
Here's a simplified example of a round-robin load balancing algorithm:
private AtomicInteger counter = new AtomicInteger(0);
public String getNextInstance(List<String> instances) {
int index = Math.abs(counter.getAndIncrement() % instances.size());
return instances.get(index);
}
In this example, we maintain an atomic counter to rotate through the list of instances in a round-robin fashion, ensuring that each instance gets its fair share of traffic.
Key Takeaways
In conclusion, Zookeeper Curator provides a powerful toolkit for implementing dynamic load balancing in a microservices environment. By leveraging its features for service registration, change detection, and distributed coordination, developers can build resilient and scalable load balancing solutions for their microservices architectures.
As microservices continue to gain traction in modern software development, the need for efficient load balancing mechanisms becomes increasingly critical. Zookeeper Curator, with its rich set of capabilities and battle-tested reliability, offers a robust foundation for addressing the challenges of microservices load balancing in Java-based applications.
By following the principles and examples outlined in this article, developers can harness the potential of Zookeeper Curator to optimize the performance and resilience of their microservices load balancing strategies, ultimately contributing to the overall stability and scalability of their distributed systems.
For more in-depth information about Zookeeper Curator and advanced use cases, refer to the official Zookeeper Curator documentation.