Optimizing Test Execution Order in JUnit 5
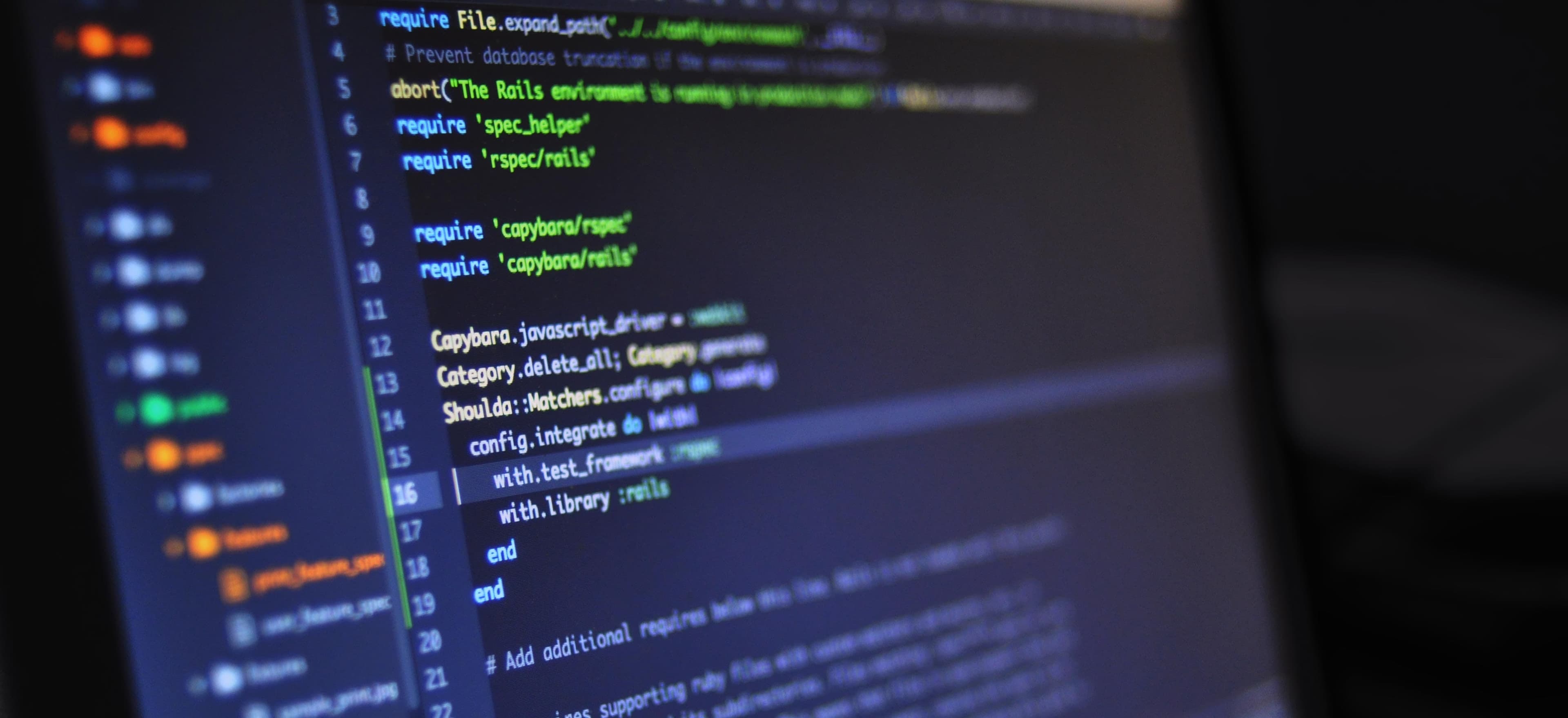
- Published on
Optimizing Test Execution Order in JUnit 5
When writing automated tests in Java, it's crucial to ensure that the order of test execution doesn't affect the outcome. However, in some cases, there may be a need to control the order in which tests are executed. This can be particularly important when dealing with integration tests or when certain tests depend on the state set by others.
In this article, we will explore how to optimize test execution order in JUnit 5, the latest version of the popular testing framework for Java. We will cover different ways to specify the order of test execution and discuss best practices for doing so.
Understanding Default Test Execution Order
By default, JUnit 5 does not guarantee the execution order of test methods within a test class. This is by design, as it encourages developers to write independent and isolated tests. This means that each test method should be able to run in any order without impacting the overall test suite.
However, there are scenarios where test dependencies or shared setup/teardown logic may require a specific execution order. In such cases, it becomes essential to have control over the test execution order.
Using @TestMethodOrder Annotation
JUnit 5 provides the @TestMethodOrder
annotation to specify the order in which test methods should be executed within a test class. This annotation can be used in conjunction with one of the built-in MethodOrderer
implementations provided by JUnit 5.
Let's take a look at an example of using @TestMethodOrder
with the MethodNameOrderer
:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.MethodOrderer;
import org.junit.jupiter.api.Order;
import org.junit.jupiter.api.TestMethodOrder;
@TestMethodOrder(MethodOrderer.MethodName.class)
public class TestExecutionOrderExample {
@Test
@Order(1)
public void firstTest() {
// Test logic
}
@Test
@Order(2)
public void secondTest() {
// Test logic
}
@Test
@Order(3)
public void thirdTest() {
// Test logic
}
}
In this example, the @TestMethodOrder
annotation is used to specify that the test methods should be ordered based on their names. Additionally, the @Order
annotation is used to explicitly define the execution order of the test methods.
By using the MethodNameOrderer
, the test methods will be executed in the lexicographic order of their names - firstTest
, secondTest
, and thirdTest
.
Customizing Test Method Order
Apart from the built-in MethodOrderer
implementations, JUnit 5 also allows you to create custom orderers by implementing the MethodOrderer
interface.
Here's an example of a custom MethodOrderer
implementation that orders test methods based on a custom annotation:
import org.junit.jupiter.api.MethodOrderer;
import org.junit.jupiter.api.MethodOrdererContext;
import org.junit.jupiter.api.TestMethodOrder;
import org.junit.platform.commons.annotation.Testable;
@TestMethodOrder(MyCustomOrderer.class)
public class CustomOrderExample {
@Test
@CustomOrder(2)
public void secondTest() {
// Test logic
}
@Test
@CustomOrder(1)
public void firstTest() {
// Test logic
}
}
public class MyCustomOrderer implements MethodOrderer {
@Override
public void orderMethods(MethodOrdererContext context) {
// Implement custom ordering logic
}
}
In this example, we define a custom annotation @CustomOrder
to specify the order of test methods. Then, we create a custom MethodOrderer
named MyCustomOrderer
to implement the custom ordering logic based on the @CustomOrder
annotation.
By using custom method orderers, you have the flexibility to define complex ordering strategies based on your specific requirements.
Test Execution Order Pitfalls
While controlling the test execution order can sometimes be necessary, it's important to note that relying too heavily on a specific order can lead to fragile and tightly coupled tests. Test cases should ideally be independent of each other.
Relying on a specific test execution order can hinder the reusability and maintainability of tests. A change in the order of test execution might result in unexpected test failures, making it harder to debug and maintain the test suite.
It's advisable to use test execution order control sparingly and only when absolutely necessary. Consider refactoring tests to eliminate order dependencies where possible.
Final Considerations
In this article, we discussed the importance of optimizing test execution order in JUnit 5 and explored various ways to achieve it. We looked at using the @TestMethodOrder
annotation with built-in and custom MethodOrderer
implementations, and also highlighted the potential pitfalls of relying heavily on test execution order.
When controlling the test execution order, it's crucial to strike a balance between ensuring the necessary order for some tests while maintaining the independence and isolation of test cases. As with any testing practice, it's important to carefully consider the trade-offs and use best practices to ensure a robust and maintainable test suite.
By understanding how to optimize test execution order in JUnit 5, you can effectively manage test dependencies and ensure the reliability of your test suite.
Remember, while optimizing test execution order can be useful, strive to write tests that are resilient to changes in execution order and do not rely on specific timing or state set by other tests.
For further reading, you may find this guide on test execution order in the official JUnit 5 documentation helpful.
As demonstrated, optimizing test execution order in JUnit 5 can be crucial for certain scenarios, but it comes with its own set of considerations. Understanding the available tools and techniques provides developers with the flexibility to manage their test suite effectively while maintaining test independence and reliability. Whether using built-in method orderers or creating custom ones, the goal remains the same - to ensure a robust and maintainable test suite for Java applications.