Optimizing Performance with Lazy Relations
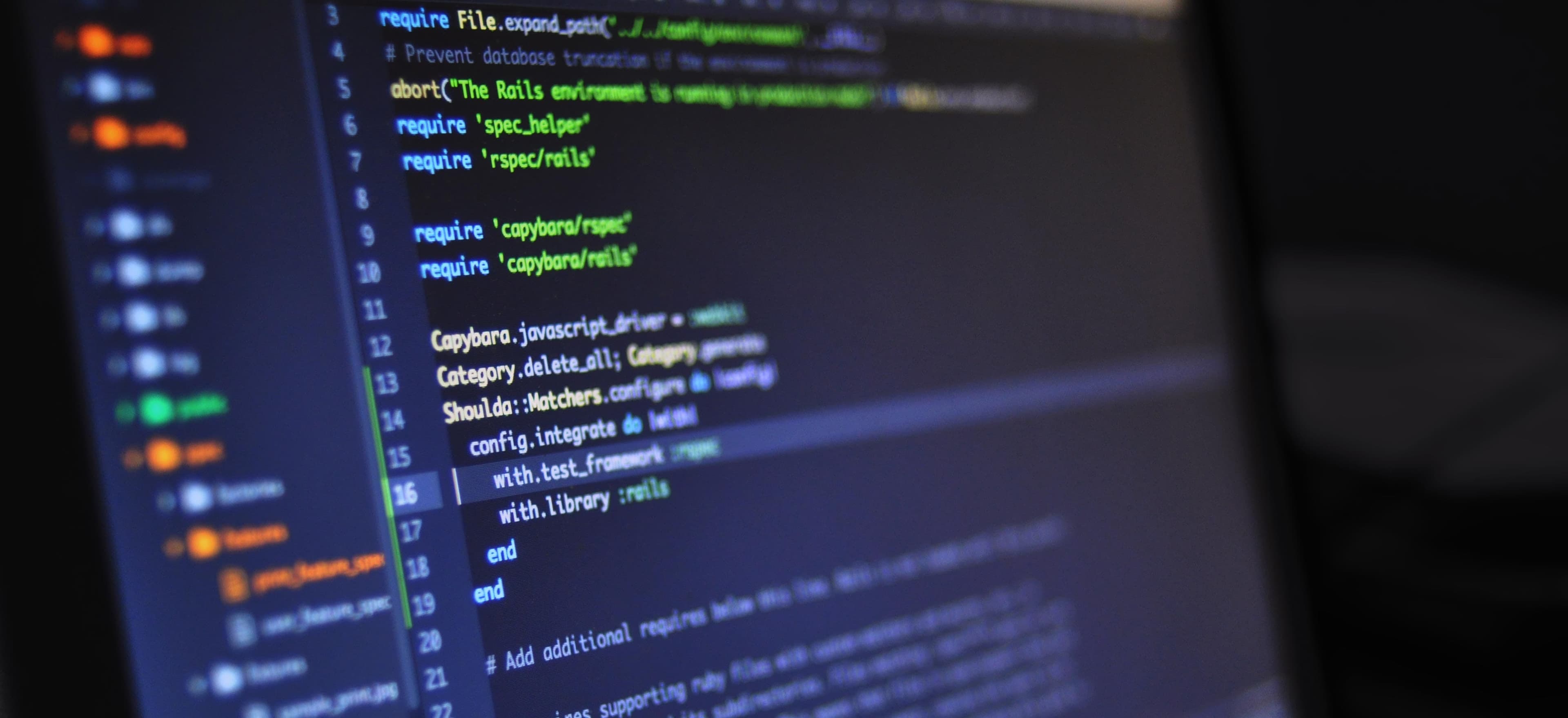
- Published on
Optimizing Performance with Lazy Relations in Java
When working with large datasets and complex object relationships in Java, optimizing performance becomes a crucial aspect of the development process. One common approach to improving performance is to employ lazy loading for object relations. In this article, we'll explore the concept of lazy relations, discuss its benefits, and provide examples of how to implement lazy loading in Java.
Understanding Lazy Loading
Lazy loading is a design pattern used to defer the initialization of an object until the point at which it is needed. In the context of object relationships, lazy loading allows us to delay the loading of related objects until they are explicitly accessed. This can be particularly beneficial when dealing with large datasets, as it helps to reduce memory consumption and improve overall application performance.
In Java, lazy loading of object relations is often achieved using libraries such as Hibernate, which provides support for lazy loading through its entity relationships. When a related object is marked as lazy, the data associated with that object is only loaded when it is accessed for the first time. This can prevent unnecessary querying and loading of data, resulting in a more efficient use of database resources.
Benefits of Lazy Loading
Implementing lazy loading for object relations in Java offers several benefits, including:
-
Reduced Memory Footprint: By deferring the loading of related objects until they are needed, we can conserve memory and prevent unnecessary loading of data that might not be used.
-
Improved Performance: Lazy loading helps to minimize the number of database queries and data retrieval operations, which can lead to faster application response times and improved overall performance.
-
Optimized Resource Utilization: By loading related objects on-demand, we can optimize the use of database resources and reduce the potential for resource contention.
Implementing Lazy Loading in Java
Let's consider a simple example to demonstrate how lazy loading can be implemented in Java using Hibernate. Suppose we have a Department
entity with a lazy-loaded relationship to Employee
entities. Here's how we can define the entity relationship in Java:
@Entity
public class Department {
// other attributes
@OneToMany(mappedBy = "department", fetch = FetchType.LAZY)
private List<Employee> employees;
// getters and setters
}
In this example, the employees
relationship is marked as lazy, indicating that the associated Employee
entities will only be loaded from the database when explicitly accessed. By using the FetchType.LAZY
option, we instruct Hibernate to apply lazy loading for the employees
collection.
When we retrieve a Department
entity from the database, the employees
collection will initially contain proxy objects instead of fully-initialized Employee
entities. The actual data will be loaded from the database only when we access the employees
collection for the first time.
Best Practices for Lazy Loading
While lazy loading can offer significant performance improvements, it's important to follow best practices to avoid potential pitfalls. Here are some tips for effectively implementing lazy loading in Java:
-
Avoid N+1 Query Problem: Be mindful of the N+1 query problem, where lazy loading results in an excessive number of database queries due to repeated access to related objects within a loop or iteration. Consider using batch fetching or join fetching to mitigate this issue.
-
Use Eager Loading When Appropriate: While lazy loading is beneficial in many scenarios, there are cases where eager loading might be more suitable, especially when the related objects are frequently accessed together with the parent object.
-
Monitor Database Access Patterns: Keep a close eye on database access patterns and query execution plans to identify any performance bottlenecks related to lazy loading. Tools like Hibernate statistics can provide valuable insights into the efficiency of lazy loading strategies.
Key Takeaways
In conclusion, lazy loading of object relations in Java can greatly improve application performance and resource efficiency. By deferring the loading of related objects until they are explicitly accessed, we can optimize memory usage, reduce database queries, and enhance overall responsiveness. When implemented thoughtfully and in accordance with best practices, lazy loading offers a powerful tool for achieving efficient data access and manipulation in Java applications.
Employing lazy loading with Hibernate is just one of the many ways to optimize performance in Java. For more insights into Java performance tuning, check out this comprehensive guide. Additionally, you can explore advanced strategies for optimizing database interactions with Java through this article.
Remember, optimizing performance is an ongoing process, and leveraging techniques like lazy loading can make a significant impact on the scalability and responsiveness of your Java applications.
Start incorporating lazy loading into your Java projects today and witness the tangible performance improvements it brings!