Mastering the Reflection API in Java
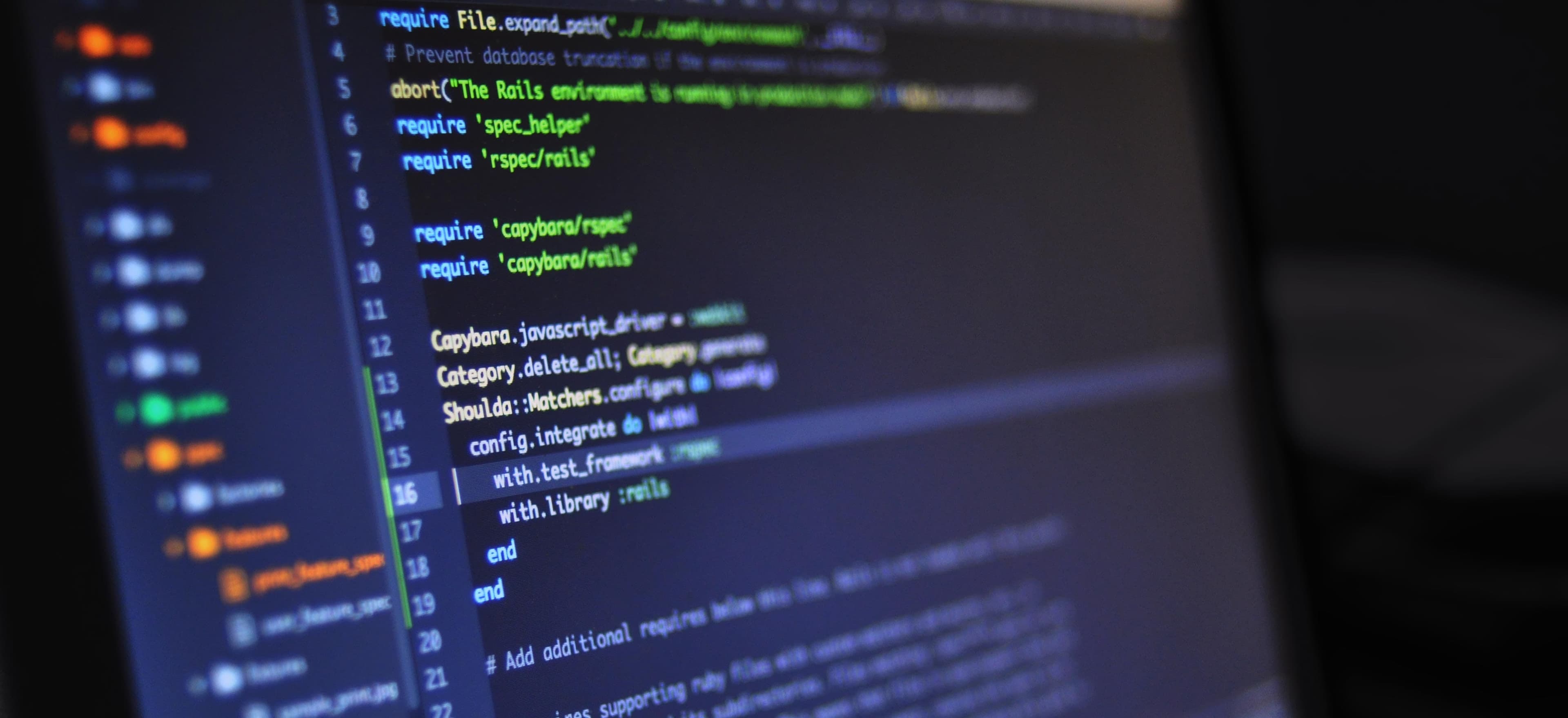
- Published on
Mastering the Reflection API in Java
When it comes to Java programming, the Reflection API stands as an exceptionally powerful tool in a developer's arsenal. It allows for the inspection and manipulation of classes, interfaces, fields, and methods at runtime. This capability provides a wide range of possibilities, including the dynamic instantiation of objects, exploration of class capabilities, and even modifying private fields. In this article, we will delve into the depths of the Reflection API, exploring its functionality and delving into its best practices.
Understanding Reflection in Java
Reflection, in the context of Java, pertains to the ability of a program to examine and manipulate itself at runtime. This means a Java program can query information about classes, interfaces, fields, and methods, as well as use this information to instantiate objects, invoke methods, and access and modify fields, regardless of their access modifiers.
The Class Object and Reflection
In Java, every class or interface is represented as an object of the Class
type. The Reflection API provides a means to access these Class
objects through the class literal syntax or by calling the getClass()
method on an object. Once we have a Class
object, we can query information about the class, such as its name, superclass, interfaces it implements, constructors, methods, and fields.
Practical Applications of Reflection
Reflection finds extensive use in frameworks and libraries that require runtime information about classes. For instance, dependency injection frameworks often use reflection to discover and instantiate classes based on their annotations. Similarly, serialization frameworks use reflection to inspect and manipulate object properties during the serialization and deserialization processes.
Exploring the Reflection API
Let's delve into some practical examples to understand the power and flexibility that the Reflection API offers.
Example 1: Dynamic Instantiation of Objects
Consider the scenario where the class name is known only at runtime, and an instance of this class needs to be created. Reflection makes this dynamic instantiation possible through the newInstance()
method.
public class ReflectionExample {
public static void main(String[] args) {
String className = "com.example.MyClass";
try {
Class<?> clazz = Class.forName(className);
Object object = clazz.newInstance();
// Use the dynamically created object
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException ex) {
// Handle exceptions
}
}
}
In this example, the Class.forName(className)
method is used to obtain the Class
object for the specified class name. The newInstance()
method is then invoked on this Class
object to dynamically create an instance of the class.
Example 2: Accessing and Modifying Private Fields
Reflection also allows access to private fields, which is otherwise not permitted in regular code. The following example demonstrates how to access and modify a private field using reflection.
import java.lang.reflect.Field;
public class ReflectionExample {
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
MyClass obj = new MyClass();
Field privateField = MyClass.class.getDeclaredField("privateField");
privateField.setAccessible(true);
privateField.set(obj, "New value");
System.out.println(obj.getPrivateField());
}
}
In this example, we obtain the Field
object representing the private field using the getDeclaredField()
method. We then set the accessibility of the field to true
using setAccessible(true)
to modify its value, followed by retrieving the modified value using the regular getter method.
Best Practices and Caveats
While the Reflection API offers immense power and flexibility, it comes with certain caveats and best practices that developers should keep in mind.
Performance Considerations
Reflection, when used indiscriminately, can lead to performance overhead. Invoking methods, accessing fields, and creating instances through reflection is slower compared to regular method invocation and object instantiation. Therefore, it's crucial to use reflection judiciously and consider its performance implications.
Handling Exceptions
Reflection methods, such as forName()
, getMethod()
, and getField()
, can throw checked exceptions such as ClassNotFoundException
, NoSuchMethodException
, and NoSuchFieldException
. It's imperative to handle these exceptions appropriately to prevent runtime failures.
Security Restrictions
In a secured environment, such as in an applet or a Java EE container, security managers can prevent certain reflective operations. It's essential to be mindful of security restrictions and handle security exceptions that may occur when using reflection in restricted environments.
Accessing Private Members
Accessing and modifying private fields and methods using reflection can bypass the encapsulation provided by the Java language, potentially leading to unexpected behavior and violating the intended design of the classes. It's advisable to use this capability sparingly and with a thorough understanding of the implications.
The Bottom Line
The Reflection API in Java empowers developers with the ability to examine and manipulate classes, fields, and methods at runtime, providing a myriad of possibilities for dynamic behavior and introspection. By understanding its capabilities, best practices, and potential pitfalls, developers can harness the power of reflection while ensuring the robustness and maintainability of their code.
In conclusion, mastering the Reflection API in Java opens up a world of dynamic possibilities for developers, enabling them to build more flexible and extensible applications.
To deepen your knowledge of Java and its capabilities, consider exploring the official Java Reflection API documentation and experimenting with real-world scenarios where reflection can add value to your projects.