Troubleshooting Spring Cloud Stream with Kafka Issues
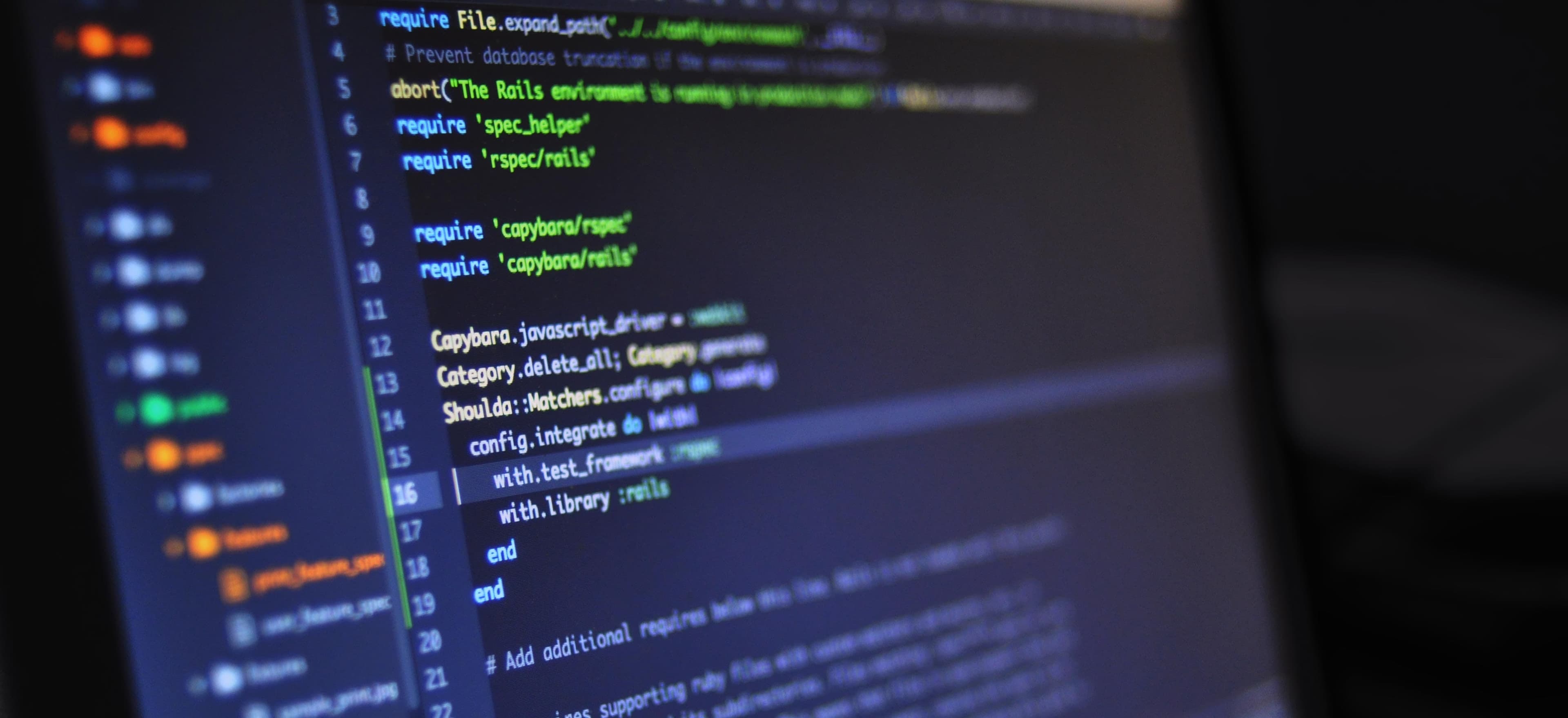
- Published on
Troubleshooting Spring Cloud Stream with Kafka Issues
Spring Cloud Stream is a powerful framework for building event-driven microservices using Spring Boot and messaging systems such as Apache Kafka. While working with Spring Cloud Stream and Kafka, developers sometimes encounter issues that can be challenging to troubleshoot. This guide will provide insights into common issues, how to diagnose them, and best practices for resolution.
Understanding Spring Cloud Stream and Kafka
Before diving into troubleshooting, it is essential to understand the components:
- Spring Cloud Stream: A framework for building message-driven microservices. It provides a simple programming model and powerful configuration options.
- Apache Kafka: A distributed streaming platform used for building real-time data pipelines and streaming applications. Kafka is known for its fault tolerance, scalability, and high throughput.
Basic Concepts
In Spring Cloud Stream, messages are sent and received via bound channels. Here’s a simple example of how to create a Kafka producer and consumer:
@SpringBootApplication
@EnableBinding(Processor.class)
public class KafkaApplication {
public static void main(String[] args) {
SpringApplication.run(KafkaApplication.class, args);
}
@Bean
public CommandLineRunner commandLineRunner(Producer producer) {
return args -> {
// Sending a message to Kafka topic
producer.sendMessage("Hello, Kafka!");
};
}
}
@Component
public class Producer {
@Autowired
private MessageChannel output;
public void sendMessage(String message) {
Message<String> msg = MessageBuilder.withPayload(message).build();
output.send(msg);
}
}
In this example, we create a Producer
component that sends messages to a Kafka topic defined in the application properties.
Common Issues and How to Troubleshoot
1. Configurations
Problem: Incorrect configurations can lead to various issues in message sending and receiving.
Solution: Ensure that your application.yml
or application.properties
file is correctly configured. Here’s an example of a typical Kafka configuration:
spring:
cloud:
stream:
bindings:
output:
destination: my-topic
producer:
partition-key-expression: headers['my-key']
input:
destination: my-topic
kafka:
binder:
brokers: localhost:9092
- Brokers: Check that the broker address is correct and that Kafka is up and running.
- Topics: Ensure that the configured topics exist in Kafka.
Why: Proper configuration is pivotal for communication between your Spring application and Kafka. Any misconfiguration can lead to lost messages or failures in communication.
2. Connection Issues
Problem: Connectivity issues to Kafka may arise due to firewall settings or incorrect broker addresses.
Solution: Use the following code to test your Kafka connection:
@Autowired
private KafkaTemplate<String, String> kafkaTemplate;
public void testKafkaConnection() {
try {
kafkaTemplate.send("test-topic", "Connection Test").get();
System.out.println("Connection successful");
} catch (Exception e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
Why: This snippet attempts to send a message to a specified topic. It helps confirm whether your application can communicate with Kafka effectively.
3. Message Serialization/Deserialization Issues
Problem: Mismatched serialization/deserialization can lead to errors when sending/receiving messages.
Solution: Specify the correct serializer and deserializer in your configuration:
spring:
cloud:
stream:
kafka:
binder:
configuration:
key.serializer: org.apache.kafka.common.serialization.StringSerializer
value.serializer: org.apache.kafka.common.serialization.StringSerializer
Why: Proper serialization ensures that the data formats are consistent, which prevents deserialization errors when messages are sent and received.
4. Consumer Lag
Problem: Consumer lag occurs when a consumer is unable to process messages as quickly as they are being produced.
Solution: Monitor your consumer's performance and check for any blocking calls in your processing code. To improve processing time, consider using asynchronous processing:
@StreamListener(Processor.INPUT)
public void handle(String message) {
CompletableFuture.runAsync(() -> {
// Process message asynchronously
processMessage(message);
});
}
Why: Asynchronous processing can reduce blockage and enhance throughput, thereby reducing consumer lag.
5. Error Handling
Problem: Unhandled exceptions during message processing can lead to message loss or retries.
Solution: Implement a dedicated error handler:
@Bean
public KafkaTemplate<String, String> kafkaTemplate() {
return new KafkaTemplate<>(producerFactory());
}
@StreamListener(Processor.INPUT)
public void process(String message) {
try {
// Processing message
} catch (Exception e) {
// Custom error handling logic
log.error("Error processing message: {}", message, e);
}
}
Why: Custom error handling ensures that your application can gracefully recover from failures and log problematic messages for further investigation.
6. Monitoring and Metrics
Problem: Lack of visibility can make it challenging to detect and troubleshoot issues dynamically.
Solution: Integrate monitoring tools such as Kafka’s JMX metrics, Micrometer, or Spring Boot Actuator to keep track of application health. For example, you can enable Actuator metrics in your configuration:
management:
endpoints:
web:
exposure:
include: "*"
Why: Monitoring provides insight into the state of your application and Kafka, helping you identify issues before they become critical.
Final Considerations
When working with Spring Cloud Stream and Apache Kafka, encountering issues is part of the development journey. However, with the right understanding of configurations, error handling, and monitoring, you can effectively troubleshoot and resolve these issues.
Additional Resources
By following the best practices outlined in this post and utilizing the provided code snippets, you can enhance your Spring Cloud Stream applications and ensure a smoother integration with Kafka. Happy coding!