Boosting Web Application Speed with ActiveJ
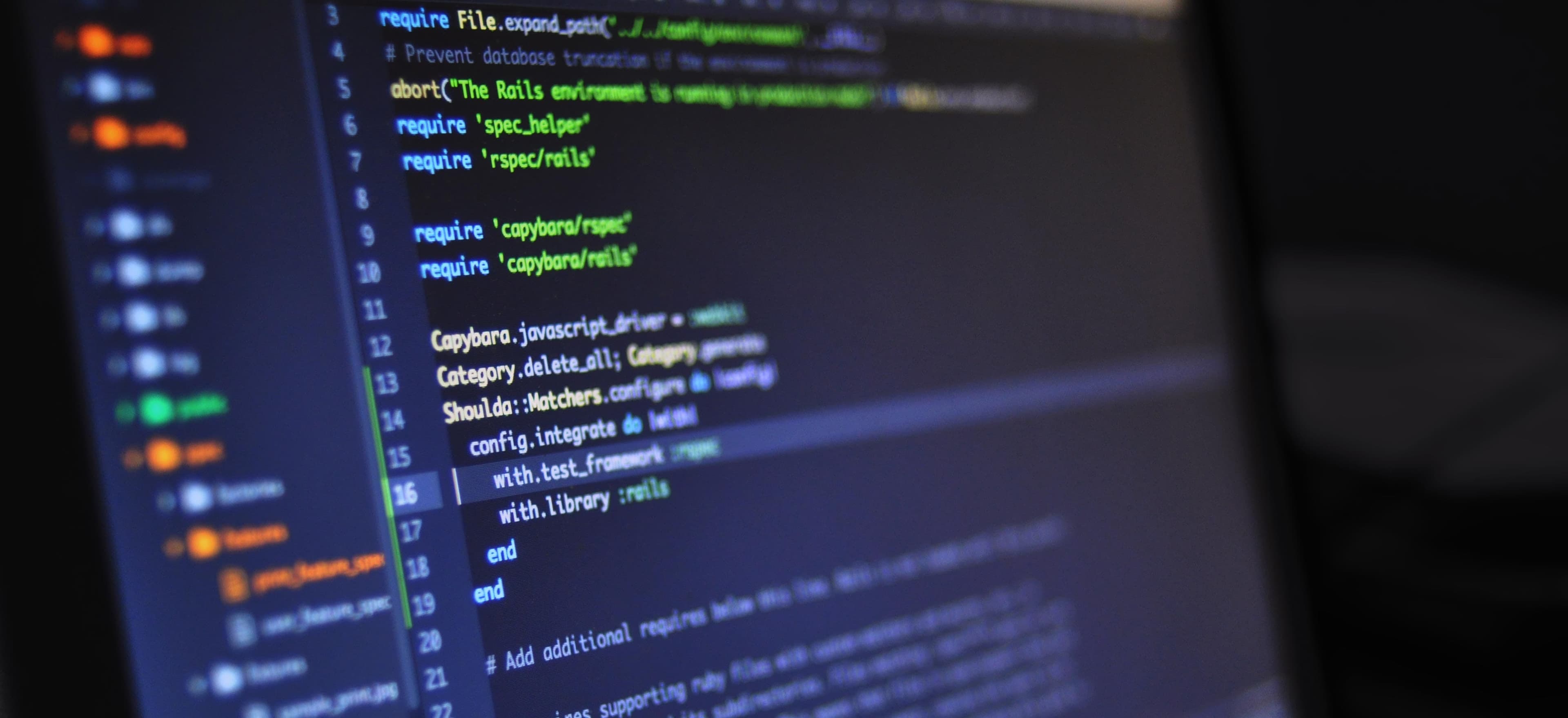
- Published on
Boosting Web Application Speed with ActiveJ
In today's competitive digital landscape, the speed and performance of web applications play a pivotal role in attracting and retaining users. With the Java ecosystem offering a plethora of frameworks and tools for web development, it's crucial to choose the right technologies to ensure optimal speed and efficiency.
One such powerful toolkit that has been gaining traction in the Java community is ActiveJ. In this article, we will explore how ActiveJ can be leveraged to enhance the speed of web applications, and discuss its key features that enable developers to build high-performance systems.
What is ActiveJ?
ActiveJ is a high-performance Java platform built for developing and running asynchronous, concurrent, and reactive applications. It provides a range of features and tools to streamline the development process and optimize the performance of web applications.
Key Features of ActiveJ
-
Asynchronous Programming: ActiveJ facilitates non-blocking, asynchronous programming, allowing applications to handle a large number of concurrent connections without creating a new thread for each request, thus conserving system resources and enhancing performance.
-
Reactive Components: With ActiveJ, developers can build reactive components that enable the application to respond to changes and events in a timely and efficient manner, promoting a more responsive and interactive user experience.
-
Dependency Injection: ActiveJ includes a robust dependency injection framework that simplifies the management of component dependencies, leading to more modular and maintainable code, while also enhancing performance by optimizing resource allocation.
-
Highly Concurrent: ActiveJ is designed to support high levels of concurrency, ensuring that web applications can process multiple requests simultaneously while maintaining responsiveness and scalability.
Now, let's delve into how ActiveJ can be utilized to boost the speed of web applications.
Leveraging ActiveJ for Speed Optimization
Asynchronous Request Handling
Traditional web application frameworks often utilize a synchronous request-handling model, where each incoming request is allocated a dedicated thread, potentially leading to resource wastage and decreased performance under heavy loads. ActiveJ, however, leverages asynchronous request handling, allowing for efficient utilization of system resources and improved throughput.
Let's take a look at a simple HTTP server implementation using ActiveJ to handle requests asynchronously:
import io.activej.http.AsyncHttpServer;
import io.activej.http.AsyncServlet;
import io.activej.http.HttpResponse;
import io.activej.http.RoutingServlet;
import io.activej.inject.annotation.Inject;
public class AsyncHttpServerExample {
@Inject
private AsyncHttpServer server;
public void startServer() {
server.withListenPort(8080)
.withHandler(RoutingServlet.create()
.map("/", request -> HttpResponse.ok200().withPlainText("Hello, World!")))
.listen();
}
public static void main(String[] args) {
AsyncHttpServerExample example = new AsyncHttpServerExample();
example.startServer();
}
}
In this example, we create an instance of AsyncHttpServer
and define a simple HTTP handler that responds with "Hello, World!" to incoming requests. By leveraging ActiveJ's asynchronous capabilities, the server can handle a large number of concurrent requests with minimal resource overhead, thus enhancing the speed and responsiveness of the web application.
Reactive Data Processing
Another area where ActiveJ excels in speeding up web applications is through its support for reactive data processing. By utilizing reactive streams and components, developers can design data processing pipelines that are inherently asynchronous and capable of handling large volumes of data with minimal latency.
Let's consider a scenario where we use ActiveJ to process incoming data streams reactively:
import io.activej.datastream.StreamSupplier;
import io.activej.promise.Promise;
import io.activej.stream.StreamConsumer;
public class ReactiveDataProcessingExample {
public Promise<Void> processData() {
StreamSupplier<String> supplier = ... // Instantiate the data supplier
StreamConsumer<String> consumer = ... // Instantiate the data consumer
return supplier.streamTo(consumer);
}
}
In this example, we create a data processing pipeline using StreamSupplier
and StreamConsumer
provided by ActiveJ. The reactive nature of the processing pipeline ensures that data is handled asynchronously, leading to improved throughput and responsiveness in the web application.
Dependency Injection for Performance Optimization
ActiveJ's built-in dependency injection framework plays a crucial role in optimizing the performance of web applications by managing component dependencies efficiently and promoting modular, loosely coupled designs. By reducing the overhead associated with manual resource allocation and management, dependency injection can contribute to the overall speed and stability of web applications.
Let's demonstrate how dependency injection with ActiveJ can enhance performance:
import io.activej.http.AsyncServlet;
import io.activej.inject.annotation.Provides;
public class DependencyInjectionExample {
@Provides
AsyncServlet asyncServlet() {
return request -> ... // Define the servlet logic
}
}
In this example, we utilize ActiveJ's @Provides
annotation to declare a dependency-providing method for an asynchronous servlet. By leveraging ActiveJ's dependency injection framework, the instantiation and management of servlet instances are handled efficiently, contributing to the overall speed and performance of the web application.
Key Takeaways
ActiveJ presents a compelling option for developers seeking to optimize the speed and performance of their web applications. Its support for asynchronous programming, reactive data processing, and efficient dependency injection equips developers with the tools necessary to build high-performance systems that can handle concurrent loads with ease.
By harnessing the capabilities of ActiveJ, developers can streamline their web application development process and deliver responsive, efficient, and scalable applications that meet the demands of modern digital experiences.
In conclusion, ActiveJ stands as a potent enabler for boosting web application speed in the Java ecosystem, and its adoption can pave the way for the development of next-generation, high-performance web applications.
To learn more about ActiveJ, you can explore the official documentation on ActiveJ's website and join the community discussions to stay updated with the latest developments in high-performance Java web development.
Give ActiveJ a try and experience the power of speed-optimized web applications in the Java ecosystem!