Java Developers Beware: Understanding Kotlin's Peculiar Null Safety
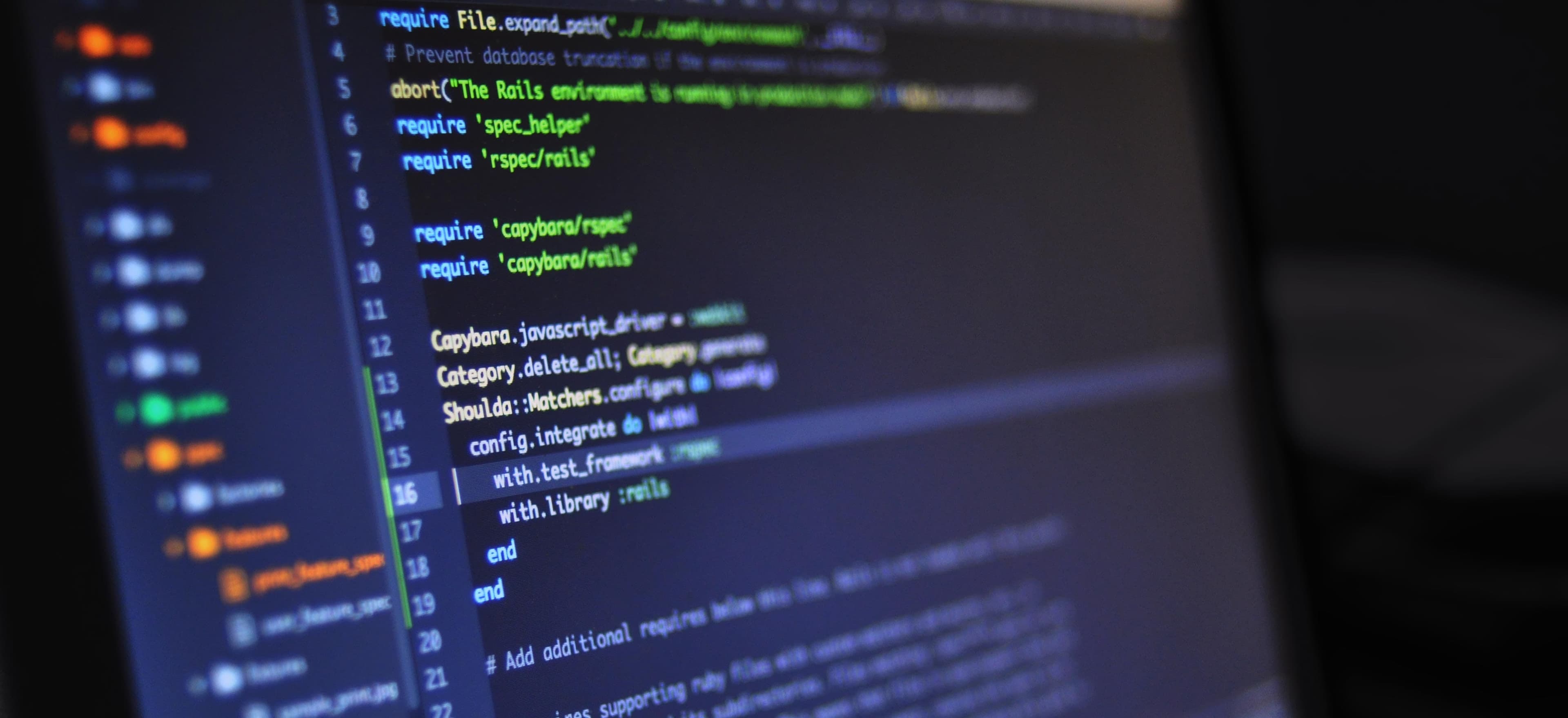
- Published on
Understanding Kotlin's Peculiar Null Safety
Getting Started
As a Java developer, you're likely familiar with the dreaded NullPointerException that tends to pop up during runtime, causing frustration and wasted time debugging. However, fear not, as Kotlin, a modern programming language for the Java Virtual Machine, provides a unique solution to this age-old problem with its built-in null safety features.
In this article, we'll delve into Kotlin's approach to null safety and understand how it differs from Java's handling of null values. By the end, you'll have a clear understanding of Kotlin's peculiar null safety and how it can make your code more robust and less error-prone.
Understanding the Nullable Type
In Kotlin, every type is non-nullable by default, meaning a variable cannot hold a null value unless explicitly specified. This is in stark contrast to Java, where every reference type can potentially be null, leading to the risk of NullPointerExceptions.
Let's take a look at a simple example in Kotlin:
var name: String = "John"
// name = null // Compiler Error: Null cannot be a value of a non-null type String
In the above code snippet, the variable name
is of type String
, and trying to assign null
to it will result in a compile-time error. This explicit handling of null values at compile time alleviates the need for excessive null checks at runtime, making the code more robust.
However, there are scenarios where we do need to allow null values, and Kotlin handles this elegantly through the use of nullable types denoted by the ?
symbol. Let's extend our example to accommodate null values:
var nullableName: String? = "Marry"
nullableName = null // No compile-time error
By appending ?
to the type declaration, as shown in the nullableName
variable, we indicate that it can hold either a valid string value or a null reference. This explicit declaration makes it clear which variables can potentially be null, thus enhancing the code's readability and safety.
Safe Calls and the Elvis Operator
In Java, working with nullable references often involves verbose null checks using conditional statements. However, Kotlin streamlines this process with its safe call operator, ?.
, and the concise Elvis operator, ?:
.
Let's consider a scenario where we want to perform an operation on a nullable value and provide a default if it is null:
val length: Int = nullableName?.length ?: 0
In the above code, nullableName?.length
checks if nullableName
is not null before accessing its length
property. If nullableName
is null, the Elvis operator ?:
provides a default value of 0. This succinct syntax replaces the boilerplate null checks present in Java, leading to more readable and maintainable code.
The !! Operator and its Risks
While Kotlin's null safety features greatly reduce the occurrence of NullPointerExceptions, there are instances where you, as a developer, may want to explicitly throw a NullPointerException. This is where the not-null assertion operator, !!
, comes into play.
The !!
operator essentially tells the compiler that you are certain that the value is not null, allowing you to bypass the null check. However, it's crucial to exercise caution when using this operator, as it can lead to unexpected runtime exceptions if the value does turn out to be null.
val length: Int = nullableName!!.length // Throws NullPointerException if nullableName is null
In the above code, the !!
operator is used to access the length
property of nullableName
without a null check. While this can be useful in certain scenarios, it should be used sparingly and with a thorough understanding of the potential risks.
Null Safety and Interoperability with Java
Given that Kotlin is designed to be fully interoperable with Java, it's essential to understand how Kotlin's null safety aligns with Java's handling of null values.
When calling Kotlin code from Java, nullable types are represented using the platform type, denoted by String!
, indicating that it can be both null and non-null. Consequently, when working with Kotlin code from Java, it's important to explicitly handle nullability, either through null checks or by using Kotlin's safe call and Elvis operators.
Final Thoughts
In conclusion, Kotlin's peculiar null safety mechanisms offer a refreshing approach to handling null values, effectively mitigating the risk of NullPointerExceptions that plague Java developers. By leveraging nullable types, safe calls, and the not-null assertion operator, Kotlin enables concise, robust, and error-resistant code.
As a Java developer exploring Kotlin, it's vital to embrace and fully comprehend Kotlin's null safety features to harness the language's full potential and write more reliable and maintainable code.
Intrigued by Kotlin's null safety and want to dive deeper into the language? Check out the official Kotlin documentation for comprehensive insights into its features and best practices.
Now armed with a newfound understanding of Kotlin's null safety, you're well-equipped to embark on your journey of writing safer and more resilient code in Kotlin. Happy coding!
Remember, embracing Kotlin's null safety may seem peculiar at first, but in the long run, it will save you countless hours of debugging and frustration.