Handling Session Management in Java Web Applications
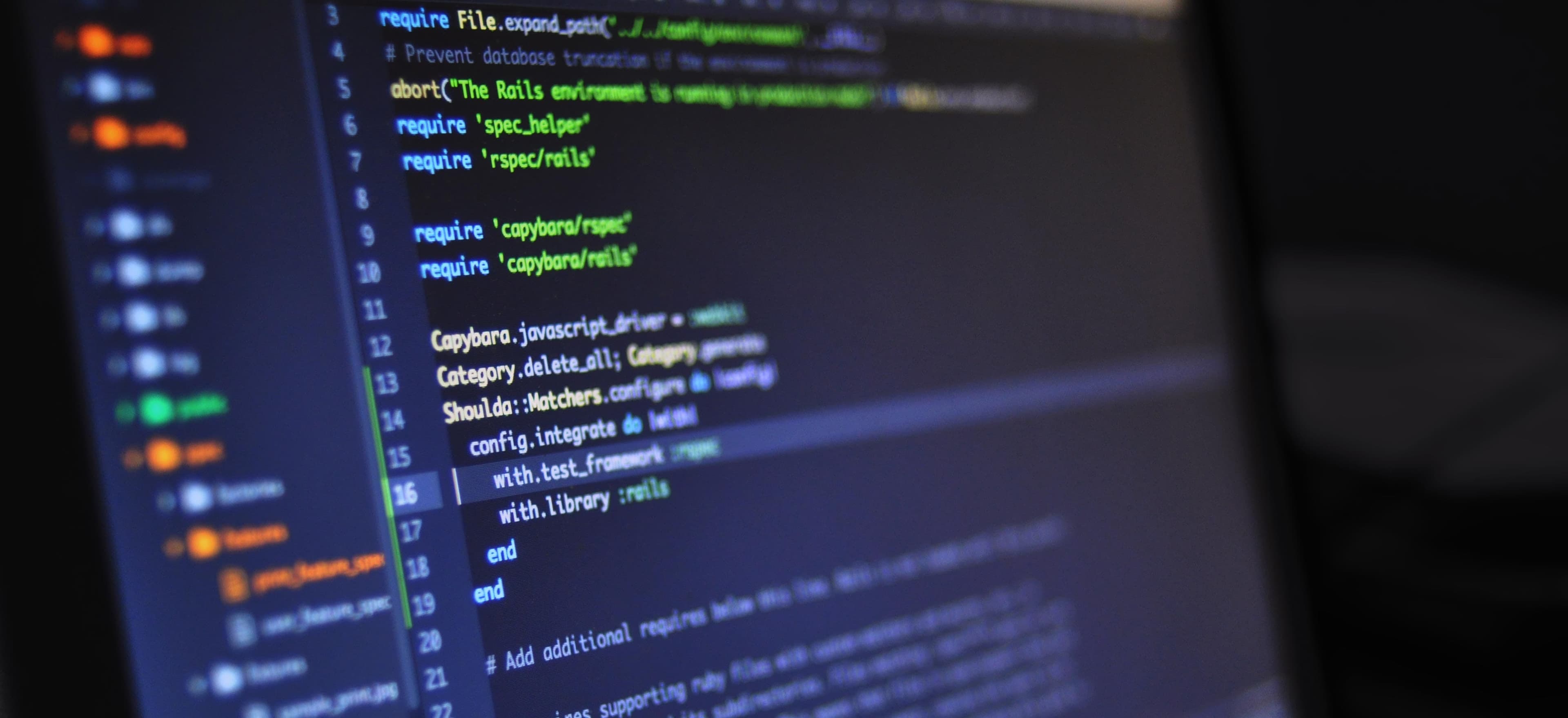
- Published on
Session management is a crucial aspect of web application development, as it allows the server to maintain state and user data across multiple requests. In Java web applications, session management is typically handled using the HTTP session. In this blog post, we will explore the fundamentals of session management in Java, including how to create, manage, and utilize sessions effectively in your web applications.
Understanding HTTP Sessions
In Java web development, an HTTP session is a mechanism to track user interactions with a web application across multiple HTTP requests. When a user accesses a web application, the server creates a unique session for that user, which is then used to store and retrieve user-specific information.
Creating and Accessing Sessions in Java
To create and access an HTTP session in a Java web application, you can use the HttpSession
interface provided by the javax.servlet.http
package.
// Creating or retrieving a session
HttpSession session = request.getSession();
This code snippet demonstrates how to create or retrieve an existing session associated with the current request. If a session already exists, it will be returned; otherwise, a new session will be created.
Storing and Retrieving Data in Sessions
Once you have a reference to the session, you can store and retrieve data using the setAttribute
and getAttribute
methods, respectively.
// Storing data in a session
session.setAttribute("username", "johnDoe");
// Retrieving data from a session
String username = (String) session.getAttribute("username");
In this example, we're storing the username "johnDoe" in the session using the key "username" and later retrieving it.
Session Expiration and Invalidation
Sessions in a Java web application have a defined lifespan, which can be configured in the deployment descriptor (web.xml) or through annotations in Servlet 3.0 and later.
// Setting session timeout (in seconds)
session.setMaxInactiveInterval(1800); // 30 minutes
By setting the maximum inactive interval, you can control how long the session remains active without any interaction from the user. Once the session exceeds this period of inactivity, it is considered expired and will be invalidated.
// Invalidating a session
session.invalidate();
Invalidating a session explicitly marks it as no longer valid, and all data associated with the session is discarded. This is commonly done when a user logs out of the application or when their session is deemed no longer needed.
Best Practices for Session Management
1. Secure Session Handling
It's important to ensure that sensitive data stored in sessions is encrypted and protected from unauthorized access. Avoid storing passwords or critical information in session attributes.
2. Use Session Cookies Securely
When a session is created, a session identifier is typically stored in a cookie on the client side. It's crucial to use secure and httponly flags for session cookies to prevent certain types of attacks.
3. Implement Session Timeout
Set a reasonable session timeout to automatically expire idle sessions and revoke access to sensitive information.
Closing the Chapter
In conclusion, effective session management is paramount for secure and efficient Java web applications. By understanding the fundamentals of session handling, implementing best practices, and leveraging the capabilities of the HttpSession
interface, developers can ensure a robust and reliable session management solution.
In this post, we've covered how to create, manage, and utilize sessions in Java web applications, alongside best practices for secure session management. By incorporating these techniques, developers can enhance the security and user experience of their web applications while maintaining the integrity of user data.
By mastering session management in Java web applications, developers can build secure, reliable, and performant web applications that provide a seamless user experience.
To delve deeper into Java web application development, take a look at our articles on Java Servlets and JSPs.
Stay tuned for more in-depth Java development insights and tutorials!