Optimizing Jenkins Docker Integration for Seamless Automation
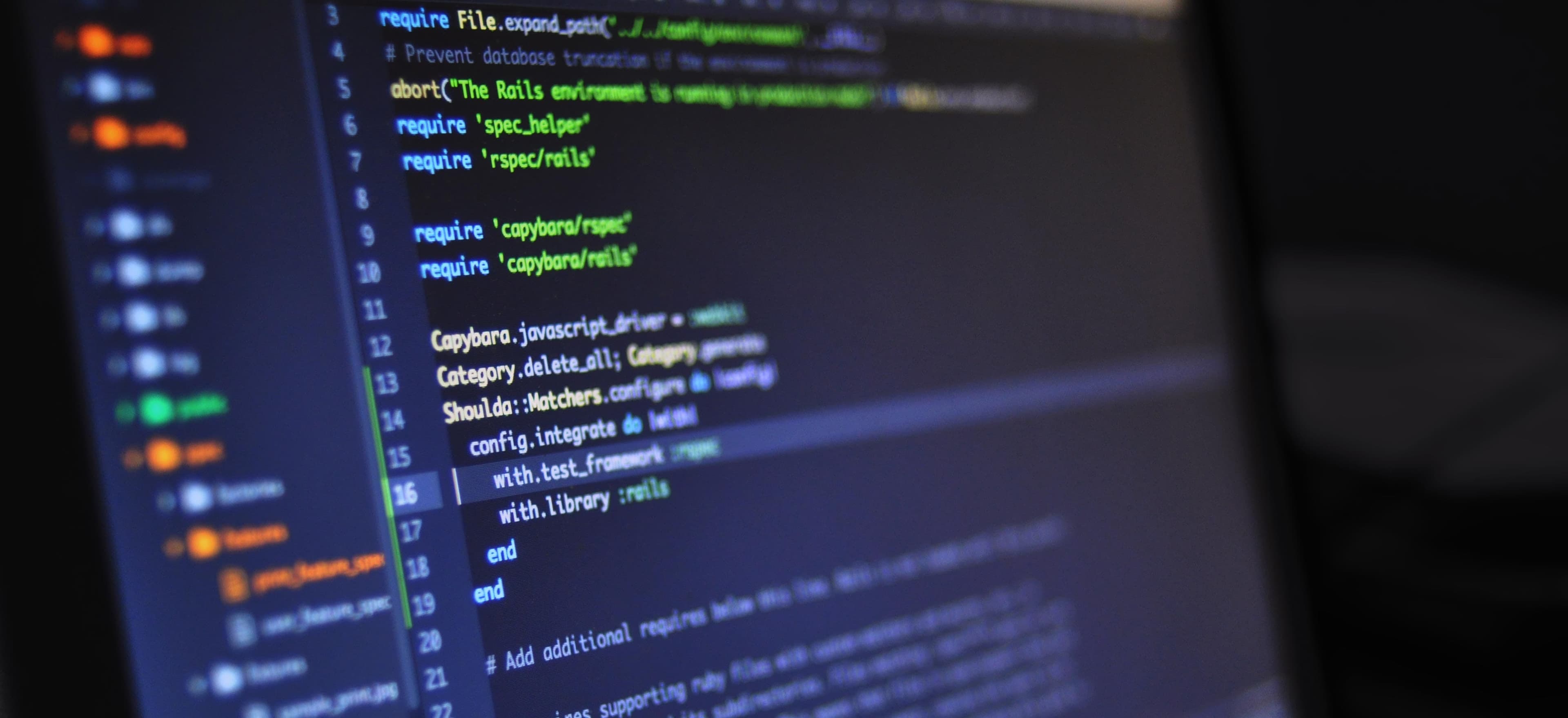
- Published on
Optimizing Jenkins Docker Integration for Seamless Automation
Jenkins is one of the most popular open-source automation servers, widely used for continuous integration and continuous delivery (CI/CD) processes. Docker, on the other hand, is a leading containerization platform that provides lightweight, portable, and self-sufficient containers for application deployment.
Integrating Jenkins with Docker can significantly enhance the automation process, allowing for efficient build, test, and deployment pipelines. In this blog post, we'll explore the best practices for optimizing Jenkins Docker integration to achieve seamless automation.
Understanding the Benefits of Jenkins Docker Integration
By combining Jenkins with Docker, teams can take advantage of several benefits:
Consistent Build Environments
Docker containers ensure that the build and test environments are consistent across different stages of the pipeline and among various team members. This consistency eliminates the infamous "it works on my machine" problem.
Scalability
Docker's lightweight containers make it easy to scale build agents on-demand, enabling efficient parallelization of tasks for faster build times.
Isolation and Security
Containers provide isolation between builds and improve security by encapsulating dependencies within a container, reducing the risk of conflicts between different builds.
Portability
Docker's portability allows builds and tests to be run in different environments seamlessly, from a developer's laptop to production servers.
Overall, integrating Jenkins with Docker streamlines the CI/CD process, improves resource utilization, and enhances the reproducibility of builds and tests.
Best Practices for Optimizing Jenkins Docker Integration
Let's delve into the best practices for optimizing Jenkins Docker integration, from setting up Jenkins to leveraging Docker for build execution.
Using the Docker Plugin for Jenkins
The Docker plugin for Jenkins allows you to define and run Jenkins agents as Docker containers. This plugin simplifies the provisioning of build agents by spinning up containerized agents dynamically, based on the build requirements.
-
Why Use the Docker Plugin? The Docker plugin offers flexibility in managing build agents, as you can specify different tools, dependencies, and configurations within the Docker image used for the agent.
-
Code Snippet:
pipeline { agent { docker { image 'maven:3.6.3-jdk-11' args '-v $HOME/.m2:/root/.m2' } } stages { stage('Build') { steps { sh 'mvn clean install' } } } }
In this pipeline, the agent is defined as a Docker container based on the Maven image, with the host's Maven repository mounted inside the container.
Utilizing Dockerized Build Environments
Creating Docker images for build environments and leveraging them in Jenkins pipelines ensures consistency and reproducibility across builds.
-
Why Use Dockerized Build Environments? Dockerized build environments encapsulate the build tools, libraries, and dependencies, guaranteeing that the same environment is used during both development and the CI/CD process.
-
Code Snippet:
FROM maven:3.6.3-jdk-11 WORKDIR /app COPY pom.xml . RUN mvn -B -f pom.xml -s /usr/share/maven/ref/settings-docker.xml dependency:resolve COPY src ./src CMD mvn -B -s /usr/share/maven/ref/settings-docker.xml package
This Dockerfile defines a Maven-based build environment, resolving dependencies and packaging the application using the specified settings file.
Managing Docker Compose for Multi-Container Applications
For applications that consist of multiple interconnected containers, using Docker Compose within Jenkins pipelines simplifies the orchestration of multi-container environments for testing and deployment.
-
Why Use Docker Compose with Jenkins? Docker Compose provides an easy way to define and run multi-container Docker applications, enabling the creation of comprehensive integration tests within CI/CD pipelines.
-
Code Snippet:
pipeline { agent any stages { stage('Build') { steps { sh 'docker-compose -f docker-compose.test.yml build' } } stage('Test') { steps { sh 'docker-compose -f docker-compose.test.yml up -d' sh 'docker-compose -f docker-compose.test.yml run test' } } stage('Deploy') { steps { sh 'docker-compose up -d' } } } }
In this pipeline, Docker Compose is used to build, test, and deploy multi-container applications, encapsulating the orchestration within the Jenkins pipeline.
Implementing Docker Image Caching
Utilizing Docker image caching within Jenkins pipelines optimizes build times by leveraging cached layers for dependencies, reducing the need to rebuild the entire image from scratch.
-
Why Implement Docker Image Caching? Docker image caching minimizes the overhead of downloading dependencies and rebuilding the entire image repeatedly, leading to faster build times and improved pipeline efficiency.
-
Code Snippet:
FROM maven:3.6.3-jdk-11 AS builder WORKDIR /app COPY pom.xml . RUN mvn -B -f pom.xml -s /usr/share/maven/ref/settings-docker.xml dependency:go-offline # ... copy source code and build application ... FROM openjdk:11-jre-slim WORKDIR /app # Copy built artifacts from the builder stage COPY --from=builder /app/target/application.jar . CMD ["java", "-jar", "application.jar"]
In this Dockerfile, the Maven dependencies are cached in a separate builder stage, reducing the need to resolve dependencies during every build.
Leveraging Docker Volumes for Persistent Data
When using Docker containers within Jenkins pipelines, leveraging Docker volumes for persistent data storage ensures that important artifacts, such as build outputs and test results, are preserved across builds and stages.
-
Why Use Docker Volumes? Docker volumes provide a way to persist data generated within containers, allowing artifacts to be shared across different stages of the pipeline and ensuring their availability for further analysis or deployment.
-
Code Snippet:
pipeline { agent { docker { image 'maven:3.6.3-jdk-11' args '-v $HOME/.m2:/root/.m2 -v $WORKSPACE:/app' } } stages { stage('Build') { steps { sh 'mvn clean install' } } stage('Archive Artifacts') { steps { archiveArtifacts artifacts: 'target/*.jar', fingerprint: true } } } }
In this pipeline, a Docker volume is used to mount the Jenkins workspace (
$WORKSPACE
) inside the Maven container, enabling the archiving of build artifacts for further use.
The Closing Argument
Optimizing Jenkins Docker integration is crucial for achieving efficient and reliable automation within CI/CD pipelines. By following the best practices discussed in this post, teams can streamline their build processes, enhance consistency, and improve the overall efficiency of their automation workflows.
By leveraging Docker containers in Jenkins pipelines, teams can create consistent, reproducible, and scalable automation processes that align with modern DevOps practices. Embracing these best practices will lead to more reliable builds, faster feedback cycles, and ultimately, higher-quality software delivery.
With the steps and code snippets provided, you are now equipped to optimize Jenkins Docker integration and drive seamless automation within your development and deployment workflows.
References:
We hope this blog post has been informative and insightful. Thank you for reading!