Troubleshooting Injection Errors in Java EE CDI
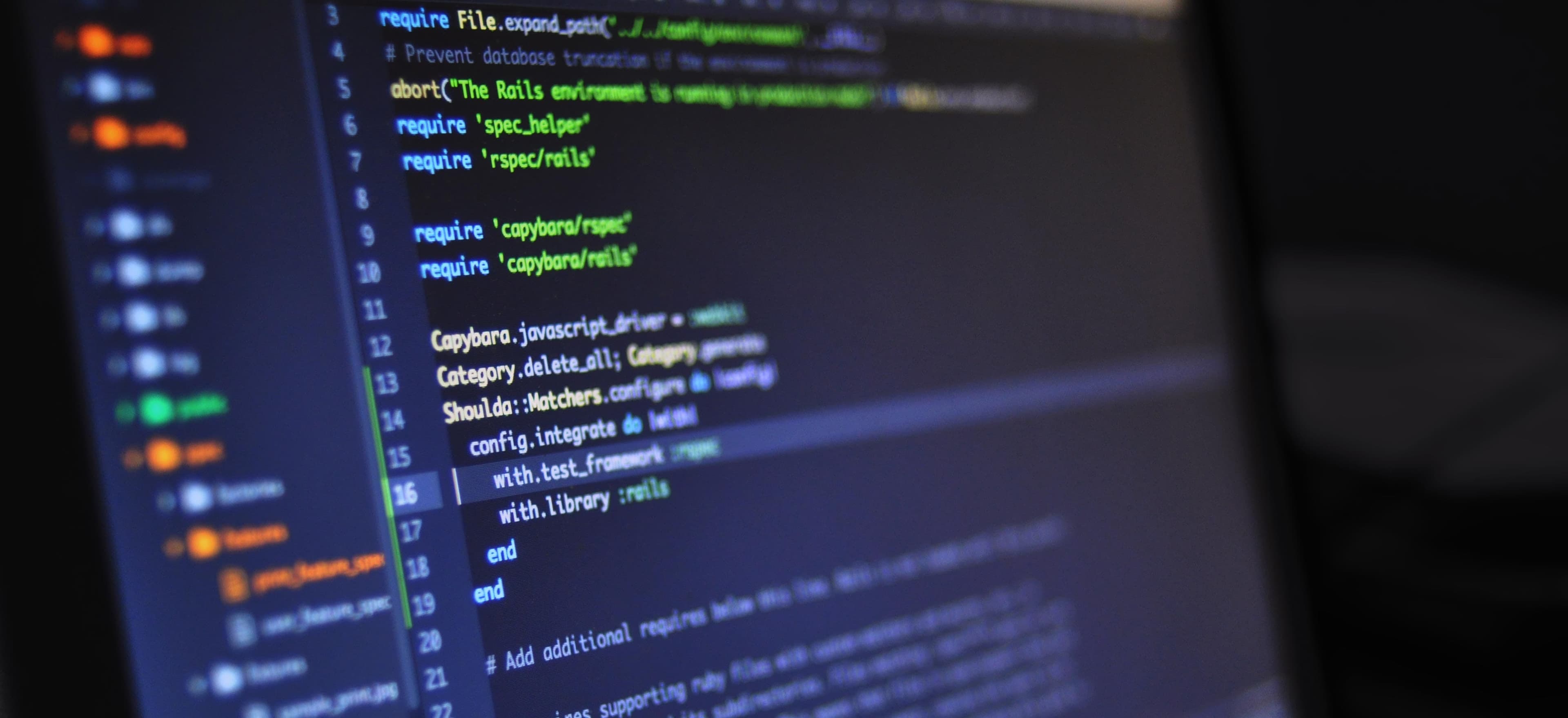
- Published on
Troubleshooting Injection Errors in Java EE CDI
In Java EE, Contexts and Dependency Injection (CDI) is a powerful tool for managing dependencies and context in your applications. However, like any technology, it's not immune to issues. One common problem that developers encounter when working with CDI is injection errors.
Injection errors can be frustrating to debug, but understanding the common causes and how to troubleshoot them can make the process much smoother. In this post, we'll explore some common injection errors in Java EE CDI and discuss how to troubleshoot and resolve them.
Understanding Injection Errors
Injection errors in CDI typically occur when the container cannot properly inject a dependency into a target class. This can happen for a variety of reasons, including misconfiguration, scope issues, or even simple typos in your code.
When an injection error occurs, it's important to understand the error message and stack trace to diagnose the issue. Common error messages include "Unsatisfied dependencies" or "Ambiguous dependencies." These messages can provide clues about what went wrong and where to start looking for a solution.
Common Causes of Injection Errors
Incorrect Annotations or Qualifiers
One common cause of injection errors is using incorrect annotations or qualifiers. For example, if you use @Autowired
instead of @Inject
in your code, the container won't be able to resolve the dependency, leading to an injection error.
Ambiguous Dependencies
Ambiguous dependencies can occur when the container is unable to determine which bean to inject due to multiple beans of the same type being available. This can happen if you have multiple beans that meet the injection point and the container cannot determine which one to use.
Unresolvable Dependencies
Unresolvable dependencies occur when the container cannot find a bean that matches the injection point. This can happen if the bean is not properly configured or if there is a typo in the injection point or bean name.
Troubleshooting Injection Errors
Now that we've covered some common causes of injection errors, let's discuss how to troubleshoot and resolve these issues.
Review Your Annotations and Qualifiers
When faced with an injection error, start by reviewing the annotations and qualifiers used in your code. Make sure you're using @Inject
and @Qualifier
where necessary, and that the qualifiers match the corresponding beans. Also, double-check that you're using the correct import statements for these annotations.
import javax.inject.Inject;
import javax.inject.Named;
public class SomeClass {
@Inject
@Named("someBean")
private SomeBean someBean;
}
In the example above, we use @Inject
to denote the injection point and @Named
with the corresponding qualifier to specify which bean to inject. Ensuring the correct usage of annotations and qualifiers can resolve many injection errors.
Qualifier Resolution
If you have multiple beans that could be injected into a specific injection point, you might need to use qualifiers to disambiguate them. Qualifiers allow you to specify additional criteria for selecting the bean to inject.
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.METHOD, ElementType.PARAMETER, ElementType.TYPE})
public @interface CustomQualifier {
String value();
}
@CustomQualifier("customBean")
public class CustomBeanA {
// Class implementation
}
@CustomQualifier("customBean")
public class CustomBeanB {
// Class implementation
}
public class SomeClass {
@Inject
@CustomQualifier("customBean")
private SomeInterface customBean;
}
In the code above, we define a custom qualifier @CustomQualifier
to disambiguate between CustomBeanA
and CustomBeanB
when injecting the SomeInterface
dependency.
Check Scope Annotations
Another potential cause of injection errors is the improper use of scope annotations. If the scope of the bean does not match the injection point, the container may not be able to resolve the dependency.
Ensure that the scope of the bean aligns with the intended injection point. For example, if you are injecting a session-scoped bean into a request-scoped bean, the container may not be able to fulfill the injection dependency.
@RequestScoped
public class RequestScopedBean {
// Class implementation
}
@SessionScoped
public class SessionScopedBean {
// Class implementation
}
In the code above, injecting the SessionScopedBean
into the RequestScopedBean
may result in an injection error due to the mismatch in scope.
Analyze Bean Configuration
It's crucial to check the configuration of your beans, including the bean names, scopes, and any qualifiers. Ensure that the bean you're trying to inject is properly configured and that there are no typos in the bean name or qualifier.
Logging and Debugging
When troubleshooting injection errors, leverage logging and debugging tools to understand the flow of dependency resolution in your application. Use logging statements to track which beans are being created and which injection points are being resolved. This can provide valuable insights into the root cause of injection errors.
Final Thoughts
Injection errors are a common stumbling block when working with Java EE CDI, but with a solid understanding of the common causes and effective troubleshooting techniques, you can quickly diagnose and resolve these issues. By reviewing annotations and qualifiers, using qualifiers to disambiguate dependencies, checking scope annotations, analyzing bean configuration, and leveraging logging and debugging, you can effectively troubleshoot injection errors in your Java EE applications.
Remember that thorough testing and continuous integration can help catch injection errors early in the development lifecycle, allowing for quick iteration and resolution. With these strategies in your toolkit, you'll be well-equipped to tackle injection errors in your Java EE CDI projects.
Further Reading:
- Java EE CDI Documentation
- Understanding CDI in Java EE
Checkout our other articles