Common Bugs to Avoid When Using JDK 12's Switch Expressions
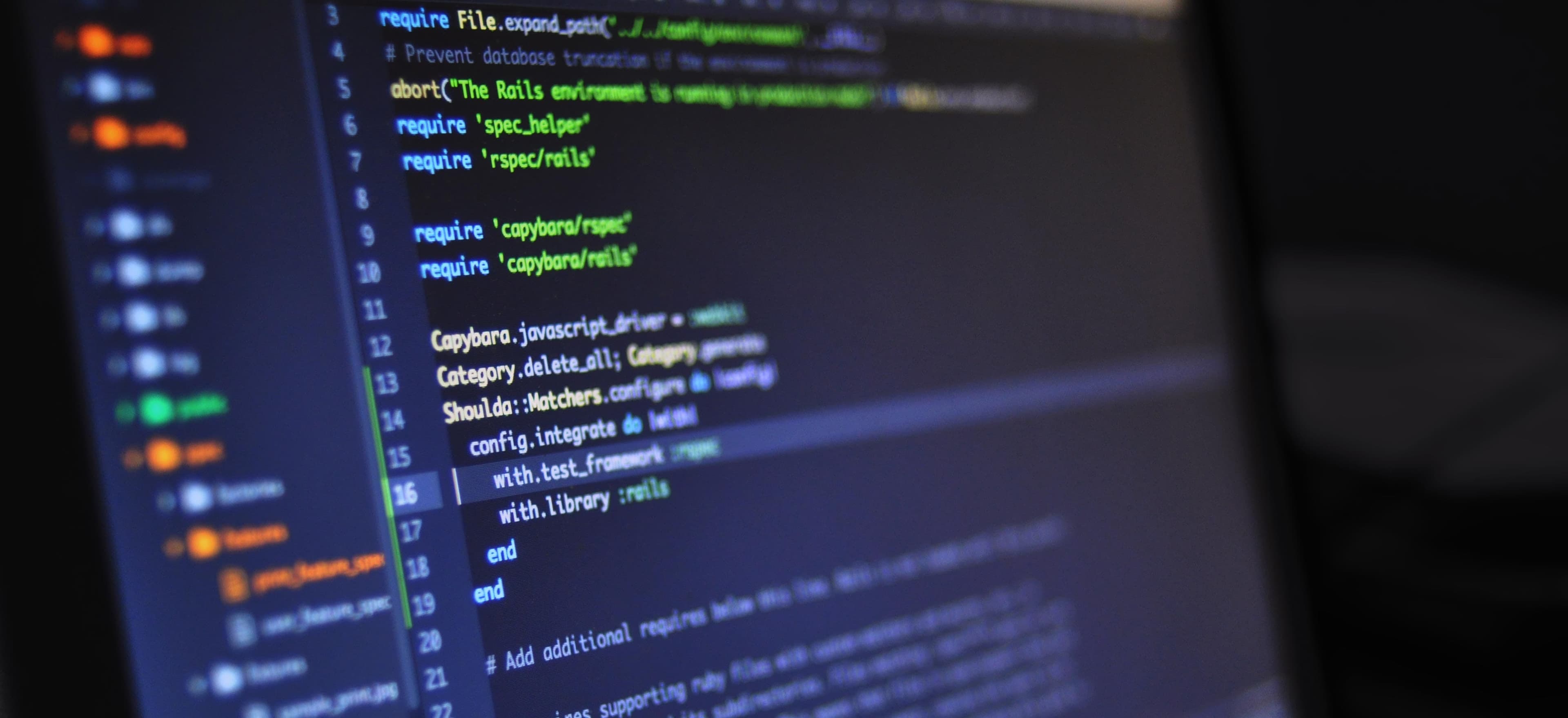
- Published on
Common Bugs to Avoid When Using JDK 12's Switch Expressions
In Java Development Kit (JDK) 12, Oracle introduced a new enhancement known as Switch Expressions. This feature aims to simplify the coding of switch statements by allowing them to be used as expressions. While this addition provides a more concise syntax and improved code readability, developers should remain cautious, as there are several common bugs associated with switch expressions that can lead to unexpected behaviors or errors. In this post, we'll explore these common bugs and discuss how to avoid them when using JDK 12's Switch Expressions.
1. Missing Break Statements
Traditionally, in switch statements, it's crucial to include break statements to exit the switch block after a particular case is executed. However, with switch expressions in JDK 12, a common mistake is forgetting to include break statements within case labels, as the new syntax allows cases to be used as expressions without the need for explicit breaks.
Example:
int dayNumber = 3;
String dayType = switch (dayNumber) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
case 4 -> "Thursday";
case 5 -> "Friday";
default -> "Weekend";
};
In this example, no break statements are necessary. The code is concise and easier to read.
2. Accidental Fall-Through
Another common bug with switch expressions is the accidental fall-through behavior, where multiple case labels are executed sequentially due to the omission of break statements.
Example:
int number = 2;
String result = switch (number) {
case 1, 2, 3 -> "Low";
case 4, 5, 6 -> "Medium";
case 7, 8, 9 -> "High";
default -> "Invalid";
};
In the above example, missing break statements leads to a fall-through scenario, resulting in unexpected behavior.
To avoid these issues, always review switch expressions thoroughly, ensuring that each case is terminated correctly with either a value or a break statement.
3. Overlapping Constant Labels
In traditional switch statements, sharing the same constant labels across different cases causes a compilation error. However, with switch expressions in JDK 12, overlapping constant labels are allowed, potentially leading to bugs or unexpected logic flows if not used carefully.
Example:
int number = 2;
String result = switch (number) {
case 1, 2, 3 -> "Low";
case 3, 4, 5 -> "Medium";
case 5, 6, 7 -> "High";
default -> "Invalid";
};
In this example, the constant label "3" is shared between the first and second case, which can result in unpredictable behavior.
To prevent issues related to overlapping constant labels, ensure that each constant value is used exclusively within its corresponding case.
Lessons Learned
While JDK 12's Switch Expressions offer a more concise and readable syntax, it's essential for developers to be aware of the common bugs associated with this feature. By paying close attention to the presence of break statements, guarding against accidental fall-through, and avoiding overlapping constant labels, developers can effectively mitigate these issues. Utilizing thorough code reviews and proper testing will further aid in identifying and addressing these bugs early in the development process.
By being mindful of these potential pitfalls, developers can fully harness the benefits of switch expressions in JDK 12 while minimizing the risk of encountering unexpected and undesirable behavior in their code.
By staying vigilant, Java developers can fully leverage the benefits of switch expressions in JDK 12 while sidestepping the risks of encountering unforeseen and undesired behavior in their code.
For more information on the topic, you can refer to the official Oracle documentation on JDK 12 Switch Expressions.
Incorporate this useful feature into your code while avoiding the most common bugs that come with it to ensure seamless integration and optimal performance.
Checkout our other articles